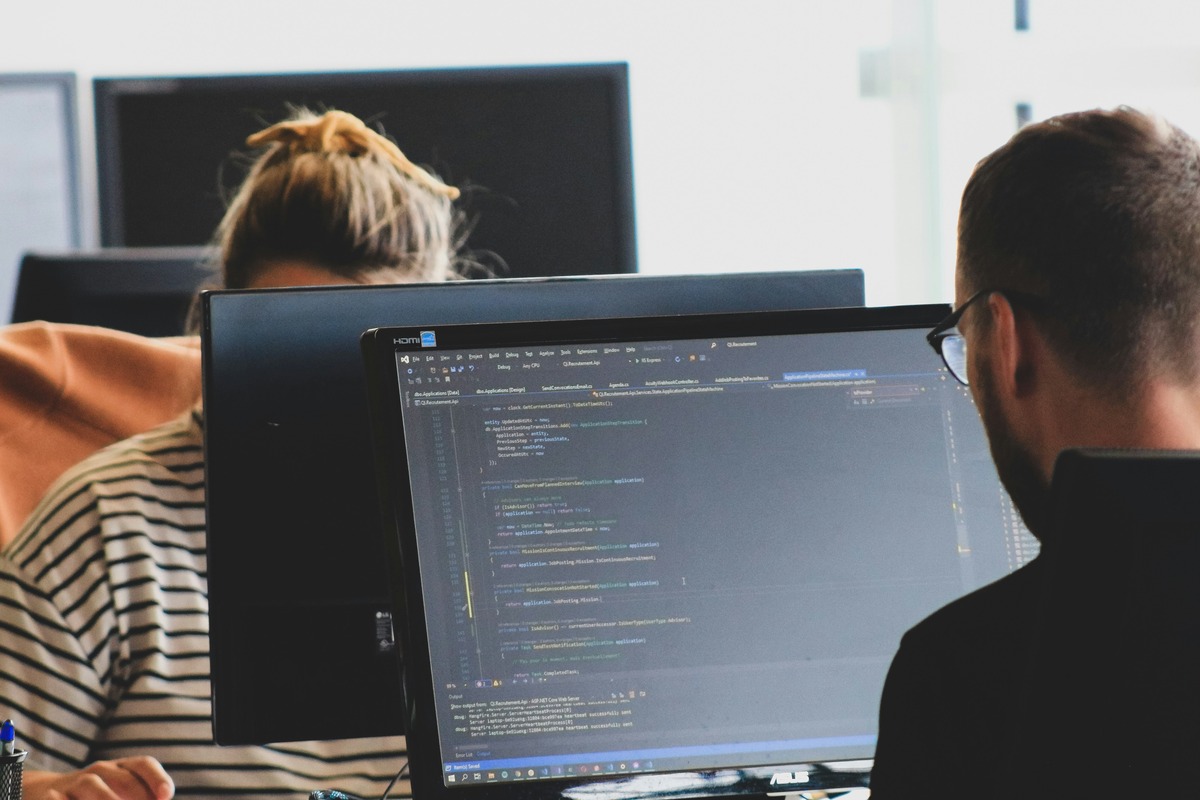
When working with JavaScript, it is important to have an understanding of data types. Data types determine the kind of values that can be stored and manipulated in a programming language. In JavaScript, there are several data types, including numbers, strings, booleans, objects, null, and undefined.
Knowing how to check the data type of a value in JavaScript is crucial for ensuring the correct handling and manipulation of data. Fortunately, JavaScript provides several methods and operators that allow developers to check the data type of a variable or value.
In this article, we will explore different techniques to check the data type in JavaScript. Whether you are a beginner or an experienced JavaScript developer, this guide will provide you with the knowledge and tools necessary to effectively determine the data type of any value in your code.
Inside This Article
- Overview of Data Types in JavaScript
- Using the typeof operator
- Checking for Primitive Data Types
- Checking for Object Data Types
- Conclusion
- FAQs
Overview of Data Types in JavaScript
In JavaScript, data types are used to determine the type and behavior of a particular value. Understanding data types is crucial for writing efficient and error-free JavaScript code. JavaScript has a dynamic or loosely-typed nature, which means that variables are not limited to a specific data type and can hold different types of values during runtime.
JavaScript has seven basic data types:
- Undefined: Represents a variable that has been declared but has not been assigned a value yet.
- Null: Represents the intentional absence of any object value.
- Boolean: Represents a logical value, either true or false.
- Number: Represents numeric values, including integers and floating-point numbers.
- String: Represents a sequence of characters, enclosed in single or double quotation marks.
- Symbol: Represents a unique identifier and is mainly used for creating unique object properties.
- Object: Represents a collection of key-value pairs, where values can be of any data type.
It is important to note that JavaScript treats arrays and functions as objects, as they can have properties and methods. Additionally, JavaScript also has other data types such as BigInt and Date, which are used for handling large integers and dates, respectively.
To manipulate and perform operations on different data types, JavaScript provides a range of built-in methods and operators. Understanding how to check and verify the data type of a value is crucial for writing reliable code and avoiding unexpected behavior.
Using the typeof operator
In JavaScript, the typeof operator is used to determine the data type of a given value or variable. It returns a string representation of the data type.
Here’s an example:
let x = 10;
let y = "Hello";
let z = true;
console.log(typeof x); // output: "number"
console.log(typeof y); // output: "string"
console.log(typeof z); // output: "boolean
In the above code, the typeof operator is used to check the data type of the variables x
, y
, and z
. The console.log statement outputs the respective data types.
The typeof operator can be used with any variable or value in JavaScript, including built-in data types like numbers, strings, booleans, as well as user-defined data types like objects and functions.
It’s important to note that the typeof operator returns a string representation of the data type, not the actual data type itself. For example, typeof 10 returns “number”, not the Number data type.
The typeof operator can also be used to check if a variable or value is undefined:
let x;
console.log(typeof x); // output: "undefined"
Additionally, if typeof is used with null, it returns “object. This is considered a historical bug in JavaScript and has been preserved for backward compatibility.
When using the typeof operator to check for data types, it’s important to handle certain edge cases properly. For example, typeof NaN returns “number”, typeof [] (an empty array) returns “object”, and typeof function(){} returns “function”.
Checking for Primitive Data Types
When working with JavaScript, it is often necessary to check the data type of a variable. This is especially important when dealing with primitive data types, such as numbers, strings, booleans, null, and undefined. Fortunately, JavaScript provides several ways to accomplish this.
One of the simplest ways to check the data type of a variable is by using the typeof
operator. The typeof
operator returns a string indicating the data type of the variable. For example:
let age = 25;
console.log(typeof age); // Output: "number"
In the above code snippet, the typeof
operator is used to check the data type of the age
variable, which holds a number. The output will be “number”.
Similarly, you can use the typeof
operator to check the data type of other primitive values:
typeof "Hello"
will return “string”typeof true
will return “boolean”typeof null
will return “object” (This is a known bug in JavaScript)typeof undefined
will return “undefined”
It is important to note that the typeof
operator returns a string representation of the data type. This can be useful in many scenarios, such as validating user inputs or handling different data types in conditional statements.
Another way to check for primitive data types is by using the instanceof
operator. The instanceof
operator checks if an object is an instance of a particular class or constructor function. This can be useful when dealing with objects that are created from custom constructor functions.
For example, let’s say we have a custom constructor function called Person
:
function Person(name, age) {
this.name = name;
this.age = age;
}
let john = new Person("John", 30);
console.log(john instanceof Person); // Output: true
In the above code snippet, we create an instance of the Person
class using the new
keyword. We then use the instanceof
operator to check if the john
object is an instance of the Person
class. The output will be true
.
It is important to note that the instanceof
operator can only be used with objects, not with primitive values. If you try to use it with a primitive value, such as a string or a number, it will always return false
.
Checking for Object Data Types
In JavaScript, you can use the typeof operator to check the data type of a variable or value. However, when it comes to checking for object data types, typeof may not provide the expected results. This is because typeof treats all objects, including arrays and null, as “object”.
To perform a more accurate check for object data types, you can use the instanceof operator. The instanceof operator allows you to check if an object is an instance of a specific class or constructor function.
Here’s an example:
let car = new Vehicle();
console.log(car instanceof Vehicle);
In this example, we create a new object “car” using the “Vehicle” constructor function. We then use the instanceof operator to check if “car” is an instance of “Vehicle”. If the output is true, it means that the object is of the specified data type.
Another way to check for object data types is by using the Object.prototype.toString() method. This method returns a string representation of the object’s data type. By calling toString() on an object and using the slice() method, we can extract the data type name from the string representation.
Here’s an example:
let book = {};
console.log(Object.prototype.toString.call(book).slice(8, -1));
In this example, we create an empty object “book”. We then use Object.prototype.toString.call() to get the string representation of the object’s data type. By using the slice() method, we extract the data type name from the string, which in this case would be “Object”.
These methods provide more accurate ways to check for object data types in JavaScript. However, it’s important to note that JavaScript is a dynamically typed language, which means that variables can hold values of different data types at different times. Therefore, it’s always a good practice to check the data type of a variable before performing any operations on it.
The ability to check data types in JavaScript is essential for any developer. By utilizing the built-in typeof operator, developers can easily determine the type of a variable or value. This information can be used for error handling, data validation, or conditionally executing certain blocks of code based on the data type.
Throughout this article, we explored various methods for checking data types in JavaScript. From using typeof for primitive types like strings and numbers, to using instanceof for objects and constructors, we covered a range of scenarios. Additionally, we discussed the limitations of typeof, such as its inability to distinguish between arrays and objects.
Understanding the data types in JavaScript is crucial for writing robust and error-free code. By effectively checking data types, developers can ensure that their code behaves as expected and avoid potential bugs or compatibility issues. Keep exploring and practicing these techniques, and you’ll be well-equipped to handle any data type challenge in JavaScript.
FAQs
**Q: How do I check the data type of a variable in JavaScript?**
A: To check the data type of a variable in JavaScript, you can use the `typeof` operator. For example, `typeof myVariable` will return a string indicating the data type of `myVariable`. The possible values returned by `typeof` are:
- `”undefined”` – if the variable has not been assigned a value.
- `”number”` – if the variable is a number.
- `”string”` – if the variable is a string.
- `”boolean”` – if the variable is a boolean.
- `”object”` – if the variable is an object or null.
- `”function”` – if the variable is a function.
**Q: How can I check if a variable is an array in JavaScript?**
A: In JavaScript, you can use the `Array.isArray()` method to check if a variable is an array. This method returns `true` if the variable is an array, and `false` otherwise. For example:
if (Array.isArray(myVariable)) { console.log("myVariable is an array"); } else { console.log("myVariable is not an array"); }
**Q: What is the difference between `null` and `undefined` in JavaScript?**
A: In JavaScript, `null` and `undefined` are both used to represent the absence of a value, but they have slightly different meanings. `undefined` is used when a variable has been declared but has not been assigned a value. In contrast, `null` is an assignment value that can be assigned to a variable to indicate the intentional absence of any object value. In other words, `null` is used to denote that a variable is currently empty or has no value.
**Q: How do I check if a variable is defined in JavaScript?**
A: To check if a variable is defined in JavaScript, you can use the `typeof` operator along with a comparison against the string `”undefined”`. For example:
if (typeof myVariable !== "undefined") { console.log("myVariable is defined"); } else { console.log("myVariable is undefined"); }
**Q: Can I check the data type of an object in JavaScript?**
A: Yes, you can check the data type of an object in JavaScript using the `typeof` operator. However, keep in mind that the `typeof` operator will always return `”object”` for objects, regardless of their specific type or class. If you need to determine the specific type of an object, you can use other approaches, such as the `instanceof` operator or the `Object.prototype.toString.call()` method.