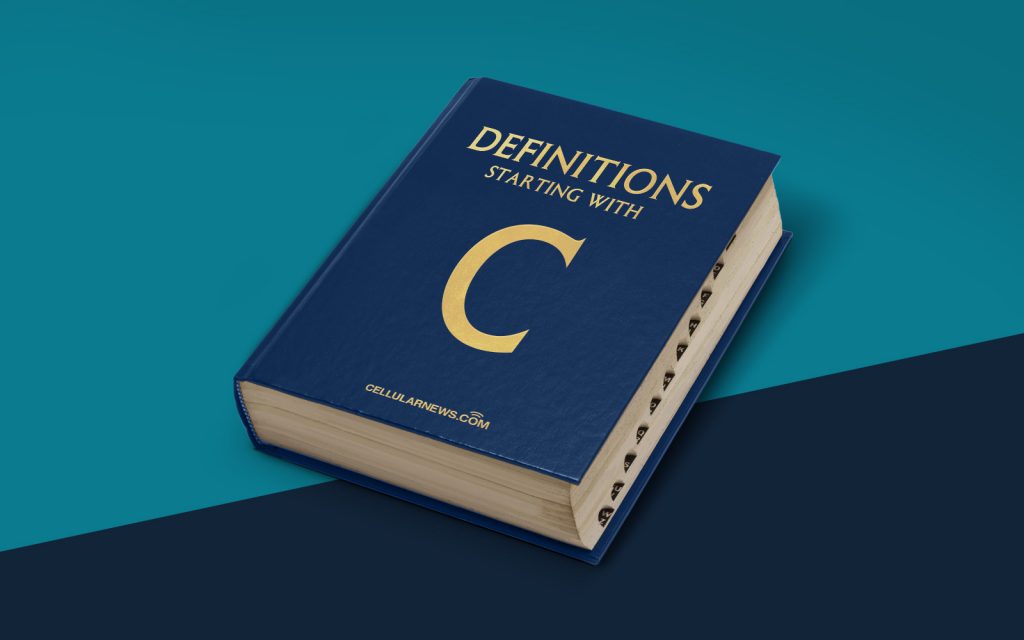
What is a Checked Operator? Understanding the Definition
Welcome to our “Definitions” category! Today, we’re diving into the world of programming and discussing a fascinating concept called the Checked Operator. If you’re new to programming or curious about expanding your knowledge, you’ve come to the right place.
So, you might be wondering, “What is a Checked Operator?” Well, I’m here to provide you with a clear definition and break it down into easily digestible terms.
Key Takeaways:
- The Checked Operator is a concept used in programming to handle arithmetic operations on numerical data types.
- It helps prevent overflow or underflow errors that may occur when values exceed the range of a particular data type.
Understanding the Checked Operator:
In programming, various arithmetic operations are performed on variables and values. However, when dealing with numerical data types, there are limits to the values they can hold. This is where the Checked Operator comes into play.
The Checked Operator is a feature or keyword in programming languages that enables checks to be placed on arithmetic operations to ensure they do not result in overflow or underflow errors.
Overflow occurs when the result of an arithmetic operation exceeds the maximum value that a data type can hold. On the other hand, underflow occurs when the result falls below the minimum value that a data type can represent. Both overflow and underflow can lead to unexpected and incorrect results in a program.
By using the Checked Operator, programmers can detect and handle such errors more effectively. When the Checked Operator is enabled for an arithmetic operation, the program verifies if the result will fit within the range of the data type. If an overflow or underflow is detected, an exception or error message is raised, allowing for appropriate actions to be taken to prevent further issues.
Now, let’s take a look at a simple example to illustrate the use of the Checked Operator:
“`csharp
int x = 2147483647; // Maximum value for an integer
int y = 1;
// Without Checked Operator – Overflow Error
int result = x + y;
Console.WriteLine(result); // Throws an exception
// With Checked Operator – Exception Handling
checked
{
int checkedResult = x + y;
Console.WriteLine(checkedResult); // Exception can be caught and handled gracefully
}
“`
As you can see from the example above, when the Checked Operator is not used, the arithmetic operation results in an overflow error due to the maximum value being exceeded. However, by enabling the Checked Operator, the exception can be caught and gracefully handled, allowing for more robust and reliable code.
Key Takeaways Recap:
- The Checked Operator is a concept used in programming to handle arithmetic operations on numerical data types.
- It helps prevent overflow or underflow errors that may occur when values exceed the range of a particular data type.
Now that you have a better understanding of what a Checked Operator is and how it can be utilized within programming, you can approach arithmetic operations with confidence, knowing that you can mitigate potential errors. Remember, implementing proper error handling techniques contributes to writing efficient and reliable code!
We hope you found this blog post both informative and insightful! Stay tuned for more exciting definitions in our “Definitions” category. Happy coding!