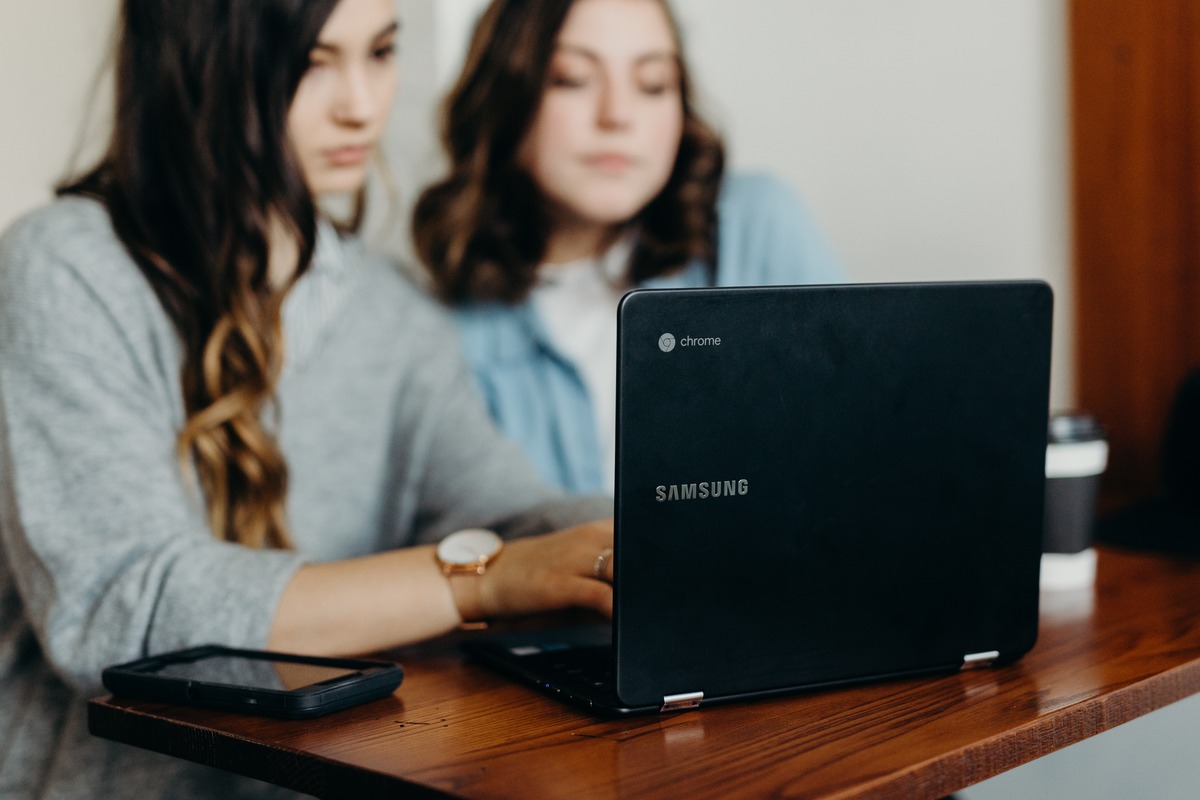
Are you interested in finding the height of a tree in data structure? Look no further, as we have you covered! Understanding the height of a tree is crucial for various algorithms and operations in data structures. Whether you’re a computer science student or a software developer, having this knowledge can greatly enhance your problem-solving skills. In this article, we will dive deep into the concept of tree height, explain how to calculate it efficiently, and provide practical examples to solidify your understanding. By the end of this article, you will have the tools and knowledge to confidently determine the height of any tree in data structure. So, let’s get started on this enlightening journey!
Inside This Article
- Overview of the problem
- Approach 1: Using Depth-First Search (DFS)
- Approach 2: Using Breadth-First Search (BFS)
- Approach 3: Using Recursion
- Conclusion
- FAQs
Overview of the problem
When working with data structures, one common task is determining the height of a tree. The height of a tree refers to the length of the longest path from the root to any leaf node. It is a fundamental metric in understanding the structure and complexity of a tree.
The height of a tree has significant implications in various applications, including but not limited to:
- Optimizing search and traversal algorithms
- Evaluating the efficiency of data storage and retrieval
- Designing balanced tree structures
- Analyzing the performance of sorting and clustering algorithms
In order to find the height of a tree, we need to consider the type of tree we are working with. Common tree types include binary trees, n-ary trees, AVL trees, and red-black trees. Each tree type may require a slightly different approach to calculate its height.
Regardless of the tree type, there are a few general approaches to finding the height:
- Using Depth-First Search (DFS)
- Using Breadth-First Search (BFS)
- Using Recursion
Each approach has its own advantages and considerations, and the choice depends on the specific requirements and characteristics of the tree structure.
In the following sections, we will explore each of these approaches in detail and discuss their implementation and efficiency.
Approach 1: Using Depth-First Search (DFS)
When it comes to finding the height of a tree in a data structure, one efficient approach is to use Depth-First Search (DFS). DFS is a traversal algorithm that explores as far as possible along each branch before backtracking.
The basic idea behind using DFS to find the height of a tree is to start from the root node and recursively visit each child node. By keeping track of the depth or level at each recursive call, we can determine the maximum depth reached, which corresponds to the height of the tree.
The algorithm follows these steps:
- Start from the root node of the tree.
- Perform a depth-first traversal, visiting each child node.
- At each recursive call, update the maximum depth reached.
- Once all child nodes have been visited, return the maximum depth.
By implementing this approach, we can efficiently find the height of a tree in a data structure. It is important to note that the height of a tree can also be interpreted as the length of the longest path from the root to any leaf node.
Let’s illustrate this approach with an example:
Consider a binary tree with the following structure:
In this case, starting from the root node, we would traverse the tree using DFS. Each recursive call would update the maximum depth reached at that point.
After visiting all nodes, we would find that the maximum depth is 3. Therefore, the height of the tree is 3.
This approach is efficient and allows us to find the height of a tree in a data structure using Depth-First Search (DFS).
Approach 2: Using Breadth-First Search (BFS)
In this section, we will explore how to find the height of a tree using the Breadth-First Search (BFS) algorithm. The BFS algorithm begins at the root node of the tree and traverses the tree layer by layer.
Here’s how the BFS algorithm works in finding the height of a tree:
- Create an empty queue and enqueue the root node.
- Initialize a variable height to 0.
- While the queue is not empty, do the following:
- Dequeue a node from the queue.
- For each child of the dequeued node, enqueue the child.
- Increment the height variable by 1.
- Return the height variable, which represents the height of the tree.
The BFS algorithm is based on the idea of exploring all nodes at the same depth level before moving on to the next level. By keeping track of the number of levels traversed, we can determine the height of the tree.
Let’s illustrate this approach with an example:
Consider the following binary tree:
5 / \ 3 8 / \ \ 2 4 10 \ 12
Using the BFS algorithm, we start at the root node with a height of 0. We enqueue the root node and enter the while loop. In the first iteration, we dequeue the root node and enqueue its children, which are 3 and 8. The height is incremented to 1. In the second iteration, we dequeue node 3 and enqueue its children, which are 2 and 4. The height is incremented to 2. In the third iteration, we dequeue node 8 and enqueue its only child, which is 10. The height is incremented to 3. In the final iteration, we dequeue nodes 2, 4, 10, and 12, but no more children are added. The height remains at 3. Therefore, the height of the tree is determined to be 3.
Using BFS to find the height of a tree provides a simple and efficient solution. The algorithm ensures that all nodes at the same depth level are explored before moving to the next level, allowing us to accurately determine the height of the tree.
Now, we have explored two approaches – DFS and BFS – to find the height of a tree in a data structure. In the next section, we will discuss another approach using recursion.
Approach 3: Using Recursion
In this approach, we can use recursion to find the height of a tree in a data structure. Recursion is a powerful technique in computer science that involves solving a problem by solving smaller instances of the same problem.
To find the height of a tree using recursion, we need to consider the following steps:
- Check if the tree is empty. If it is, then the height is 0.
- If the tree is not empty, recursively find the height of the left subtree.
- Recursively find the height of the right subtree.
- Return the maximum height between the left and right subtrees, plus 1.
Let’s break down these steps further:
Step 1: Check if the tree is empty – This step is crucial as it serves as the base case for our recursion. If the tree is empty, it means there are no nodes in the tree, and thus, the height is 0.
Step 2: Recursively find the height of the left subtree – In this step, we recursively call the function to find the height of the left subtree. This involves moving down the left branch of the tree and counting the number of levels or steps needed to reach the leaf nodes.
Step 3: Recursively find the height of the right subtree – Similar to step 2, we recursively call the function to find the height of the right subtree. This involves moving down the right branch of the tree and counting the number of levels or steps needed to reach the leaf nodes.
Step 4: Return the maximum height between the left and right subtrees, plus 1 – Once we have obtained the heights of both the left and right subtrees, we compare them and return the maximum height between the two. Adding 1 accounts for the current node being counted as a level or step.
By following this recursive approach, we can efficiently determine the height of a tree in a data structure.
Conclusion
Calculating the height of a tree is a fundamental concept in data structures. By understanding the different algorithms, such as recursive and iterative approaches, you can confidently determine the height of a tree. The recursive method is particularly efficient for complex and deeply nested trees, while the iterative method allows for a more iterative approach.
Regardless of the specific algorithm, it is important to note that the height of a tree provides valuable insights into its overall structure and performance. By knowing the height, you can optimize search operations, balance the tree, and manage memory efficiently.
With the knowledge gained from this article, you can now confidently navigate through the intricacies of calculating the height of a tree in data structures. Whether you are a computer science student or a seasoned developer, understanding this concept is crucial for building efficient and robust algorithms.
FAQs
- Q: Can I find the height of a tree in a data structure?
- Q: How can I find the height of a tree?
- Q: What is the difference between height and depth of a tree?
- Q: Can a tree have a height of zero?
- Q: How does the height of a tree affect its performance?
A: Yes, you can find the height of a tree in a data structure. The height of a tree is defined as the number of edges on the longest path between the root node and a leaf node in the tree.
A: To find the height of a tree, you can use various algorithms such as depth-first search (DFS) or breadth-first search (BFS). These algorithms will traverse the tree and keep track of the maximum depth or level reached during the traversal, which corresponds to the height of the tree.
A: The height of a tree is defined as the number of edges on the longest path between the root node and a leaf node in the tree. On the other hand, the depth of a node in the tree is defined as the number of edges on the path from the root node to that particular node.
A: No, a tree cannot have a height of zero. At the minimum, a tree must have one node, which is the root node. Therefore, the height of a tree with only one node is one.
A: The height of a tree can have a significant impact on the performance of certain operations in the data structure. For balanced trees, where the height is minimized, operations such as insertion, deletion, and searching can be performed in O(log n) time. However, in the case of unbalanced trees with larger heights, these operations may take longer, potentially leading to slower performance.