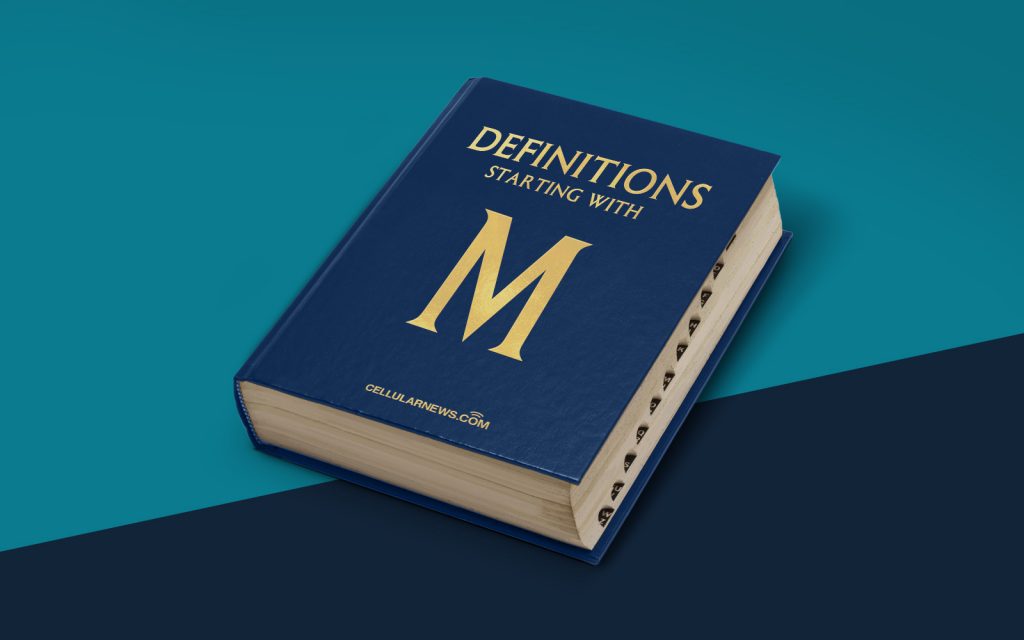
What is a Mutator in C#?
Greetings, aspiring programmers! Welcome to the “Definitions” category of our blog, where we unravel the mysteries of coding terms. Today, we are going to dive into the concept of mutators in C#. So, what exactly is a mutator in C#?
A mutator, also known as a setter, is a special type of method in C# that allows you to modify the value of an object’s private fields. In simpler terms, mutators act as a gateway to change the state or values of private variables within a class. By using mutators, you can control how the variables are set and provide additional logic or validation.
Key Takeaways:
- A mutator, or setter, is a method in C# that allows you to modify the value of an object’s private fields.
- Mutators help control how variables are set and provide additional logic or validation.
In C#, mutators are commonly used as part of the property syntax. When you define a property, you can provide both a getter and a mutator. The getter allows you to retrieve the value of the private field, while the mutator allows you to modify its value. This encapsulation of fields with mutators helps maintain data integrity and adhere to the principles of object-oriented programming.
Here’s an example to illustrate how a mutator enables controlled modification of private fields:
“`csharp
public class Person
{
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
}
“`
In the above code snippet, we have a class called “Person” with a private field called “name. The public property “Name” uses a mutator to enable access to the private field. By assigning a value to the “Name” property, the mutator allows us to change the value of the “name” field while maintaining control and enforcing any necessary rules or conditions.
Using mutators can be particularly beneficial when you need to perform validations or calculations before setting the value of a field. For example, you could implement logic inside a mutator to ensure that a string is never assigned an empty value, or to calculate and update a derived property whenever its dependency changes.
Remember, mutators give you the power to control the modification of private fields. They help with data encapsulation, maintain code integrity, and allow for additional logic or validation. So, the next time you’re building a C# application, make sure to leverage mutators wisely for a more robust and maintainable codebase!
In Summary
- A mutator, or setter, is a special type of method in C# that allows you to modify the value of an object’s private fields.
- Using mutators helps control how variables are set and provides additional logic or validation.
- They are commonly used in the property syntax to enable controlled modification of private fields.
- Mutators are essential for maintaining data integrity and adhering to object-oriented principles.
Thank you for joining us for this enlightening journey into the world of mutators in C#. Stay tuned for more definitions and programming insights in our “Definitions” category. Happy coding!