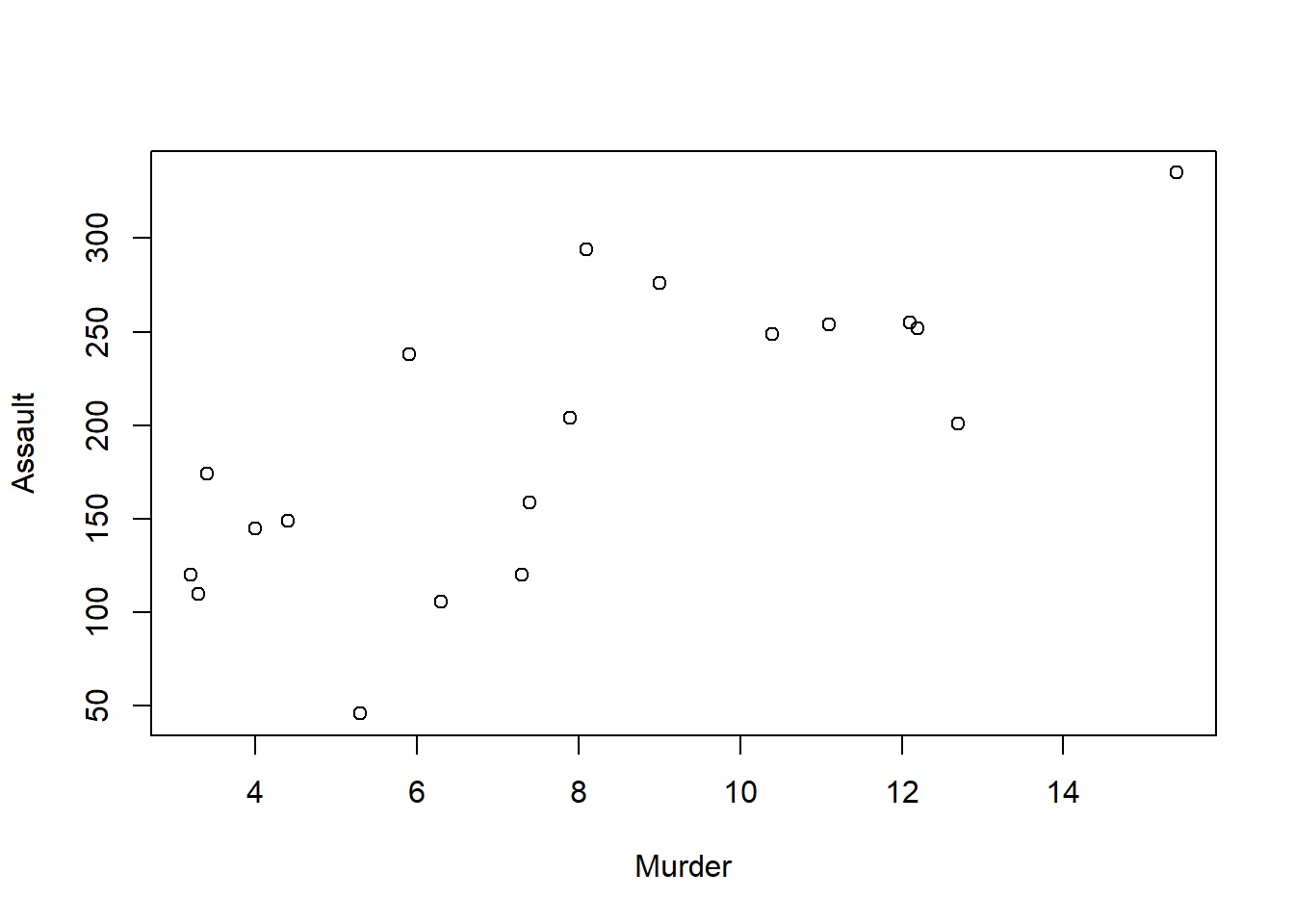
When working with data in R, it is often necessary to subset or filter specific portions of the data based on certain conditions. Subset selection allows you to extract only the subset of data that meets a specific criteria, making it easier to analyze and visualize the relevant information.
Whether you need to filter data based on numerical values, categorical variables, or a combination of conditions, R provides powerful functions and operators to help you accomplish this task. In this article, we will explore different methods to subset data in R based on condition, providing step-by-step instructions and examples.
By learning how to subset data in R, you can gain greater control over your analysis, focus on the data that matters most, and extract valuable insights from your dataset. So let’s dive right in and discover the various techniques for subset selection in R!
Inside This Article
- Subsetting Data using the Base R Subset Function
- Subsetting Data using the dplyr Package
- Subsetting Data based on a Single Condition
- Subsetting Data based on Multiple Conditions
- Conclusion
- FAQs
Subsetting Data using the Base R Subset Function
When working with data in R, it is often necessary to extract a subset of the data based on certain conditions. The base R subset function provides a simple and straightforward way to accomplish this task.
The syntax for subsetting data using the base R subset function is as follows:
subset(x, subset, select, drop = NULL)
where x
is the data object, subset
is the condition to be applied, select
is the variables to be selected, and drop
specifies whether to drop unused factors or not.
To understand how the subset function works, let’s consider an example:
data <- data.frame(ID = c(1, 2, 3, 4, 5),
Name = c("John", "Jane", "Eric", "Amy", "Tom"),
Age = c(25, 30, 27, 32, 35),
Gender = c("Male", "Female", "Male", "Female", "Male"))
Suppose we want to extract the rows from the above data frame where the age is greater than 30. We can do this using the subset function as follows:
new_data <- subset(data, Age > 30)
The resulting new_data
data frame will only contain the rows where the age is greater than 30.
In addition to single conditions, the subset function can also handle multiple conditions using logical operators such as &&
(AND) and ||
(OR).
For example, if we want to extract the rows where the age is greater than 30 and the gender is "Male", we can do so with the following code:
new_data <- subset(data, Age > 30 & Gender == "Male")
The resulting new_data
data frame will contain the rows that satisfy both conditions.
The subset function in base R provides a flexible and efficient way to subset data based on specific conditions. It is a powerful tool for data manipulation and analysis.
Please note that the subset function works on data frames, matrices, and vectors, making it a versatile function for data subsetting in R.
Subsetting Data using the dplyr Package
The dplyr package is a powerful tool for data manipulation and transformation in R. It provides a concise and intuitive syntax for subsetting data based on conditions. With dplyr, you can easily filter and extract subsets of data that meet specific criteria.
To begin subsetting data using the dplyr package, you first need to install and load the package by running the following code:
R
install.packages("dplyr")
library(dplyr)
Once you have loaded the dplyr package, you can use the `filter()` function to subset data based on one or more conditions. The `filter()` function takes a data frame as its first argument and one or more conditions as subsequent arguments.
Let's say you have a data frame called `my_data` with columns like "name", "age", and "gender". To subset the data and filter only the rows where the age is greater than 30, you can use the following code:
R
filtered_data <- filter(my_data, age > 30)
In this example, the `filter()` function extracts only the rows where the "age" variable is greater than 30 and assigns the result to a new data frame called `filtered_data`.
You can also apply multiple conditions while subsetting data using the dplyr package. For example, to filter the rows where the age is greater than 30 and the gender is "female", you can use the following code:
R
filtered_data <- filter(my_data, age > 30, gender == "female")
In this case, the `filter()` function combines the conditions using logical operators such as `&&` (and) or `||` (or).
The dplyr package also provides other useful functions, such as `select()`, `arrange()`, and `mutate()`, which can be used in combination with `filter()` for more complex data subsetting and manipulation tasks.
Overall, the dplyr package offers a streamlined and efficient way to subset data in R. Its intuitive syntax and powerful functions make it a valuable tool for any data analyst or scientist working with large datasets.
Subsetting Data based on a Single Condition
Subsetting data based on a single condition is a common task in data analysis using R. It allows you to extract a subset of data that satisfies a specific condition. This is useful when you want to focus on specific subsets of your dataset for further analysis or visualization.
In R, you can subset data based on a single condition using various approaches. One of the simplest and most commonly used methods is using the subset() function. This function allows you to extract rows from a data frame that meet a specified condition.
For example, let's say you have a data frame named "df" that contains information about students, including their names, ages, and test scores. If you want to extract the rows of students who scored above 80 on the test, you can use the following code:
subset_df <- subset(df, score > 80)
This code creates a new data frame called "subset_df" which contains only the rows from the original data frame "df" where the score is greater than 80.
Another approach to subset data based on a single condition is by using logical indexing. This involves creating a logical vector that indicates whether each row in the data frame satisfies the condition or not. You can then use this logical vector to subset the data frame.
Using the previous example, you can create a logical vector by comparing the scores to 80:
logical_vector <- df$score > 80
The resulting logical vector will be a series of "TRUE" and "FALSE" values, indicating which rows satisfy the condition.
Finally, you can use this logical vector to subset the data frame:
subset_df <- df[logical_vector, ]
This code selects only the rows from the data frame "df" where the corresponding values in the logical vector are "TRUE". The comma after the logical vector indicates that you want to select all columns.
Subsetting data based on a single condition allows you to focus your analysis on specific subsets of your dataset. Whether you choose to use the subset() function or logical indexing, both approaches are effective in extracting the desired data and enabling further analysis.
Subsetting Data based on Multiple Conditions
When working with data in R, there are often cases where you need to subset the data based on multiple conditions. This can be useful when you want to filter specific rows or observations that meet certain criteria. Fortunately, R provides several ways to achieve this.
One of the common ways to subset data based on multiple conditions in R is by using the logical operators "AND" and "OR. The logical operator "AND" is represented by "&" and requires both conditions to be true for the subset to be selected. On the other hand, the logical operator "OR" is represented by "|" and selects the subset if any of the conditions are true.
To demonstrate this, let's say we have a dataset of cell phone sales and we want to subset the data where the phone brand is "Samsung" and the price is greater than $500. We can use the following code:
subset_data <- sales_data[sales_data$brand == "Samsung" & sales_data$price > 500, ]
In this code, we are using the subset function to create a new dataset called subset_data. We specify the conditions inside the square brackets [], where "sales_data$brand == "Samsung"" checks for rows with the brand "Samsung" and "sales_data$price > 500" checks for rows with a price greater than 500. The comma at the end is used to indicate that we want to select all columns.
Another way to subset data based on multiple conditions is by using the %in% operator. This operator allows you to check if a value is present in a vector. For example, if we want to subset the data where the phone brand is either "Samsung" or "Apple", we can use the following code:
subset_data <- sales_data[sales_data$brand %in% c("Samsung", "Apple"), ]
In this code, we are using the %in% operator to check if the brand is either "Samsung" or "Apple". The resulting subset will contain all rows where the brand matches either of these two options.
It is also possible to nest multiple conditions within parentheses to create more complex subsets. For example, if we want to subset the data where the phone brand is "Samsung" or "Apple" and the price is greater than $500, we can use the following code:
subset_data <- sales_data[(sales_data$brand %in% c("Samsung", "Apple")) & sales_data$price > 500, ]
In this code, we are nesting the brand conditions within parentheses to apply the logical operator "AND" with the price condition. This will give us a subset of data that meets both conditions.
Overall, subsetting data based on multiple conditions in R allows you to filter and extract specific information from your dataset. By using logical operators and the %in% operator, you can create complex subsets that meet your specific criteria.
Conclusion
Subset data in R based on condition allows for efficient data manipulation and analysis. By using the subset() function or logical operators, you can extract specific rows or columns from a dataset that meet specific criteria. This enables you to focus on relevant data and perform targeted analyses.
When subset data, it is important to ensure that the conditions are accurately defined and that you have a clear understanding of your dataset. By using logical operators like "==", "<", ">", you can define conditions to filter the data accordingly.
Furthermore, understanding how to subset data can be a powerful tool when dealing with large datasets or when you need to analyze specific segments of your data. It can save time, enhance efficiency, and provide more precise insights.
By mastering the art of subsetting data in R, you gain a valuable skill in data analysis that will greatly contribute to your ability to make informed decisions and uncover meaningful patterns in your data.
FAQs
1. What is data subset in R?
Data subset in R refers to the process of selecting a specific subset of data from a larger dataset based on certain conditions or criteria. It allows you to filter and extract only the relevant data that meets the specified conditions.
2. How can I subset data in R based on a condition?
To subset data in R based on a condition, you can use the subset() function or the square bracket [ ] notation. The subset() function allows you to specify the condition as an argument, while the [ ] notation allows you to directly filter the data using logical conditions.
3. Can you provide an example of subsetting data in R?
Sure! Let's say you have a dataset named "data" with columns "age" and "gender". To subset the data where age is greater than 30 and gender is "Male", you can use the following code:
subset_data <- subset(data, age > 30 & gender == "Male")
Alternatively, you can use the [ ] notation as follows:
subset_data <- data[data$age > 30 & data$gender == "Male", ]
4. What if my condition involves multiple options?
If your condition involves multiple options, you can use the %in% operator. For example, to subset data where the "gender" column is either "Male" or "Female", you can use the following code:
subset_data <- subset(data, gender %in% c("Male", "Female"))
5. Are there any other functions in R for data subsetting?
Yes, apart from the subset() function and the [ ] notation, you can also use the filter() function from the dplyr package for data subsetting in R. The filter() function allows you to specify multiple conditions using logical operators to subset data efficiently.