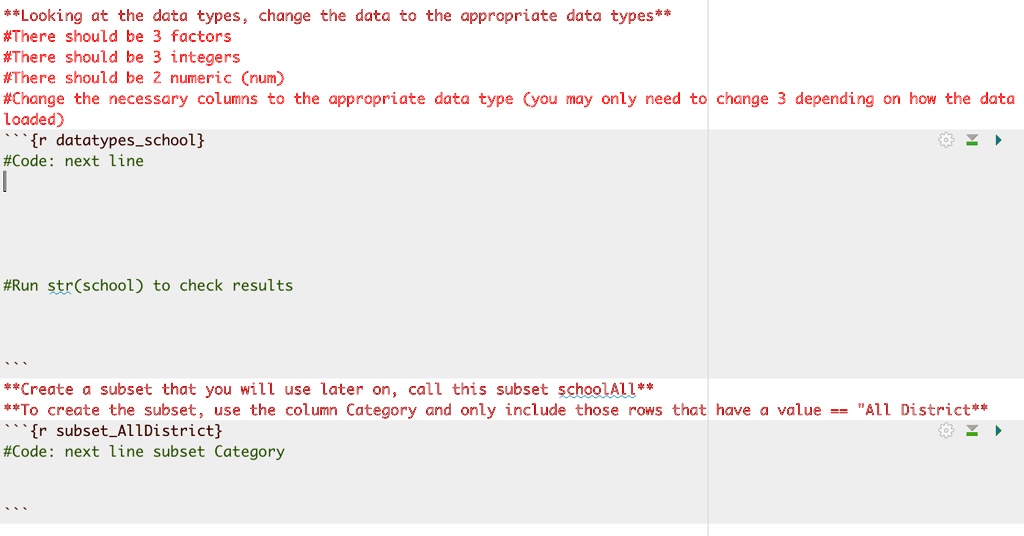
When working with data in R, it’s essential to understand the data type of the variables you’re dealing with. The data type determines how the data is stored and what operations can be performed on it. Whether you’re a beginner or an experienced R programmer, being able to check the data type is a fundamental skill.
Knowing the data type can help you troubleshoot errors, ensure proper data manipulation, and choose the appropriate statistical analysis for your data. In this article, we will explore various methods to check the data type in R. From simple functions like typeof() and class() to more advanced techniques such as str() and summary(), we will cover a range of options to meet your specific needs and preferences.
So, if you’re ready to dive into the world of data types in R and gain a deeper understanding of your data, let’s get started!
Inside This Article
- Methods to Check the Data Type in R
- Using the typeof() function
- Using the class() function
- Using the is.*() functions
- Using the `str()` function
- Conclusion
- FAQs
Methods to Check the Data Type in R
When working with data in R, understanding the data types is crucial for data manipulation and analysis. R is a dynamically typed language, which means that variables can hold values of different data types. In this article, we will explore different methods to check the data type of variables in R.
There are several functions in R that can be used to determine the data type:
- typeof(): The typeof() function returns a character string representing the type of the object. It is the most basic and fundamental way to check the data type.
- class(): The class() function returns the class or classes of the object. It is commonly used to determine the data type of an object. The class of an object determines how the object behaves in R’s object-oriented programming system.
- is.*(): R provides a set of is.*() functions that return logical values indicating whether an object belongs to a certain class or type. For example, is.numeric() returns TRUE if the object is of type numeric.
- str(): The str() function provides a concise summary of the structure of an R object, including its data type. It gives a more detailed output compared to the other methods mentioned above.
Let’s explore each method in more detail.
The typeof()
Function
The typeof() function is the most basic way to check the data type in R. It returns a character string representing the data type of the object. For example:
x <- 10
typeof(x)
# Output: "double"
y <- "Hello"
typeof(y)
# Output: "character"
The typeof() function provides the fundamental data type of the object, such as “double” for numeric values and “character” for strings.
The class()
Function
The class() function returns the class or classes of an object. It is commonly used to check the data type of an object. For example:
v <- c(1, 2, 3)
class(v)
# Output: "numeric"
w <- data.frame(a = c(1, 2, 3), b = c("A", "B", "C"))
class(w)
# Output: "data.frame"
The class() function provides more specific information about the data type of the object, such as “numeric” for numeric vectors and “data.frame” for data frames.
The is.*()
Functions
R provides a set of is.*() functions that return logical values indicating whether an object belongs to a certain class or type. These functions are useful when you want to check if an object is of a specific data type. For example:
x <- 5
is.numeric(x)
# Output: TRUE
y <- "Hello"
is.character(y)
# Output: TRUE
The is.numeric() function returns TRUE if the object is of type numeric, while is.character() returns TRUE if the object is of type character.
The str()
Function
The str() function provides a concise summary of the structure of an R object, including its data type. It is especially useful for complex objects like data frames. For example:
data(mtcars)
str(mtcars)
# Output:
# 'data.frame': 32 obs. of 11 variables:
# $ mpg : num 21 21 22.8 21.4 18.7 18.1 14.3 24.4 22.8 19.2 ...
# $ cyl : num 6 6 4 6 8 6 8 4 4 6 ...
# $ disp: num 160 160 108 258 360 ...
# $ hp : num 110 110 93 110 175 105 245 62 95 123 ...
# $ drat: num 3.9 3.9 3.85 3.08 3.15 2.76 3.21 3.69 3.92 3.92 ...
# $ wt : num 2.62 2.88 2.32 3.21 3.44 ...
# $ qsec: num 16.5 17 18.6 19.4 17 ...
# $ vs : num 0 0 1 1 0 1 0 1 1 1 ...
# $ am : num 1 1 1 0 0 0 0 0 0 0 ...
# $ gear: num 4 4 4 3 3 3 3 4 4 4 ...
# $ carb: num 4 4 1 1 2 1 4 2 2 4 ...
The str() function gives a detailed output, including both the data type and the structure of each variable in the data frame.
These methods provide various ways to check the data type in R. By understanding the data types, you can effectively manipulate and analyze your data to extract meaningful insights.
Using the typeof() function
In R, one of the most straightforward ways to check the data type of a variable or object is by using the typeof()
function. This function returns a character string indicating the data type of the object.
For example, if you have a variable named age
and you want to check its data type, you can simply use the typeof(age)
expression. The function will output a character string representing the data type, such as “numeric”, “character”, “logical”, or “list”.
Here’s an example:
age <- 25
typeof(age)
# Output: "double"
In this example, the age
variable is of type "double", which is the default data type for numeric values in R.
It's important to note that the typeof()
function is not always the most precise way to determine the data type, especially for complex objects like data frames or lists. In such cases, using the class()
or is.*()
functions, which will be covered in the following sections, might provide more accurate results.
Using the class() function
The class() function in R allows you to check the data type of an object. It returns the class or classes of an object as a character vector.
To use the class() function, simply pass the object you want to check as the argument. The function will return the class or classes of the object. If the object has multiple classes, they will be listed in the order of precedence.
Here is an example to illustrate how to use the class() function:
R
# Create an object of type character
my_string <- "Hello, World!"
# Check the class of the object
class_result <- class(my_string)
# Print the result
print(class_result)
In this example, we defined a variable called `my_string` and assigned it the value "Hello, World!". We then used the class() function to check the class of the object and stored the result in the `class_result` variable.
When we print the `class_result` variable, we will see that the class of the object is "character".
The class() function is particularly useful when you have objects with multiple classes. For example, in R, a data frame is a special type of object that can have both a data frame class and additional classes for each column.
Using the class() function, you can easily determine the classes associated with the different components of the data frame.
Here is an example:
R
# Create a data frame
df <- data.frame(name = c("Alice", "Bob", "Charlie"),
age = c(25, 30, 35))
# Check the class of the data frame
class_result <- class(df)
# Print the result
print(class_result)
In this example, we created a data frame called `df` with two columns: "name" and "age". We then used the class() function to check the class of the data frame and stored the result in the `class_result` variable.
When we print the `class_result` variable, we will see that the class of the data frame is "data.frame" and "list".
By using the class() function, you can easily check the data type of an object in R. This can be helpful when working with complex data structures or when debugging your code.
Using the is.*() functions
Another way to check the data type in R is by using the is.*() functions. These functions return a logical value indicating whether an object belongs to a specific data type. Here are some commonly used is.*() functions:
- is.character(): This function checks whether an object is of type character. It returns TRUE if the object is a character, and FALSE otherwise.
- is.numeric(): This function checks whether an object is of type numeric. It returns TRUE if the object is a numeric value, and FALSE otherwise.
- is.integer(): This function checks whether an object is of type integer. It returns TRUE if the object is an integer value, and FALSE otherwise.
- is.logical(): This function checks whether an object is of type logical. It returns TRUE if the object is a logical value (TRUE or FALSE), and FALSE otherwise.
- is.factor(): This function checks whether an object is of type factor. It returns TRUE if the object is a factor variable, and FALSE otherwise.
- is.data.frame(): This function checks whether an object is of type data frame. It returns TRUE if the object is a data frame, and FALSE otherwise.
To use these functions, you simply pass the object you want to check as an argument. For example:
my_string <- "Hello, World!"
is.character(my_string) # Returns TRUE
my_numeric <- 15.7
is.numeric(my_numeric) # Returns TRUE
my_logical <- TRUE
is.logical(my_logical) # Returns TRUE
By using the is.*() functions, you can easily check the data type of an object in R. This can be useful when you need to perform different operations or apply specific functions based on the data type.
Using the `str()` function
The `str()` function in R is a powerful tool for checking the data type and structure of an object. It provides a concise and informative summary of the object's structure, including its class, dimensions, and variable types.
To use the `str()` function, simply pass the object as the argument. For example:
str(my_data)
This will display a detailed summary of the object `my_data`, including the class of the object and information about each variable within it.
The output of the `str()` function is highly informative and allows you to quickly understand the structure of your data. It provides information such as the number of observations and variables, the data type of each variable, and any missing values present.
The `str()` function also handles nested structures efficiently. If you have a list or dataframe with complex nested structures, the `str()` function will recursively display the structure of the nested elements, making it easier to understand the overall structure.
One of the advantages of using the `str()` function is its ability to handle large datasets efficiently. It does not show the entire dataset, but rather provides a summary of the structure. This makes it time and memory-efficient, especially when dealing with large datasets.
Furthermore, the `str()` function is a great debugging tool. It allows you to quickly identify any inconsistencies or unexpected data types in your object. By understanding the structure of your data, you can easily spot any errors or mismatches that need to be addressed.
Conclusion
Checking the data type in R is an essential skill for any data scientist or analyst. By understanding the nature of your data, you can ensure that it is handled appropriately and effectively in your analysis. In this article, we have explored various methods to check the data type in R, including the class() function, typeof() function, and is.* functions. Each of these methods provides valuable insights into the structure and characteristics of the data at hand.
Using the class() function allows you to determine the basic data type of an object, while the typeof() function provides more detailed information. Additionally, the is.* functions help you determine if an object belongs to a specific data type or class. By combining these methods, you can confidently assess and manipulate your data based on its type.
Remember to always check the data type before applying any operations or transformations, as it will guide you in choosing the appropriate methods and techniques. Having a thorough understanding of the data type in R will improve the accuracy, efficiency, and reliability of your analysis.
FAQs
Q: How can I check the data type in R?
To check the data type in R, you can use the `class()` function. For example, if you want to check the data type of a variable named `x`, you can use `class(x)`. This will return the data type of the variable.
Q: Can I convert a variable's data type in R?
Yes, you can convert a variable's data type in R using various functions such as `as.integer()`, `as.character()`, `as.logical()`, etc. These functions allow you to convert a variable to a specific data type.
Q: How can I check the data type of a column in a data frame?
To check the data type of a column in a data frame, you can use the `class()` function on that specific column. For example, if you want to check the data type of a column named `age` in a data frame named `df`, you can use `class(df$age)`.
Q: What are the different data types in R?
R has several data types, including numeric, integer, character, logical, complex, and factor. Numeric data types are used to represent continuous numerical values, integer data types represent whole numbers, character data types represent text or string values, logical data types represent TRUE or FALSE values,complex data types represent complex numbers, and factor data types represent categorical variables.
Q: How do I convert a data type in a data frame in R?
To convert a data type in a data frame in R, you can use the `mutate()` function from the `dplyr` package. This function allows you to create a new column in the data frame with a different data type by using functions like `as.integer()`, `as.character()`, `as.logical()`, etc.