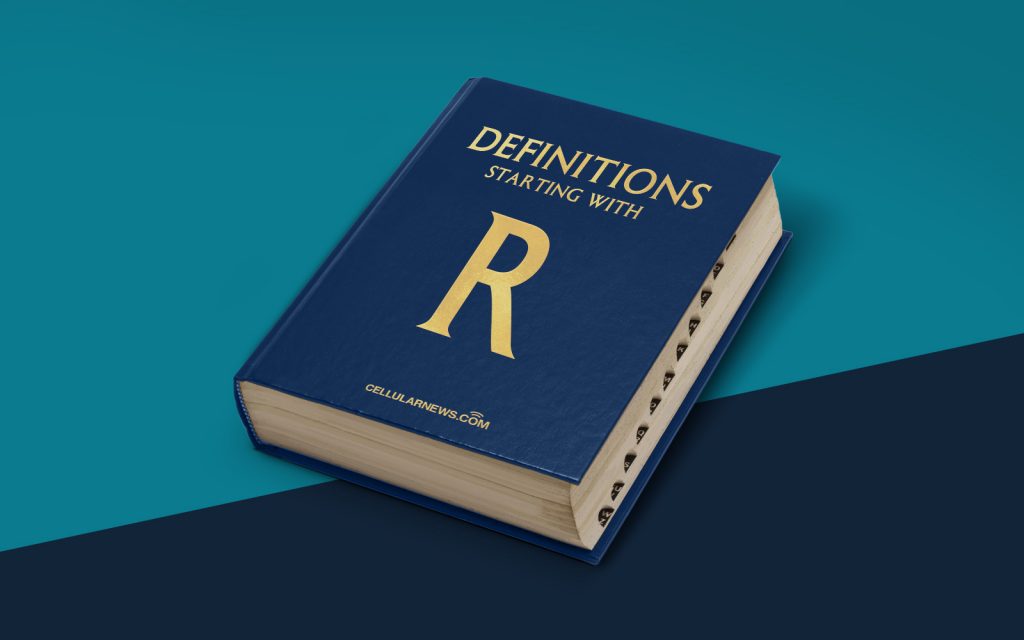
What is a Recursive Loop? A Closer Look at this Fundamental Programming Concept
Welcome back to our “DEFINITIONS” series, where we dive deep into the world of programming terminology! In today’s post, we are going to explore the concept of a recursive loop.
Have you ever wondered how a computer program can perform repetitive tasks without getting stuck in an endless cycle? That’s where recursive loops come into play. A recursive loop, also known as recursion, is a programming technique that allows a function or method to call itself repeatedly until it meets a specified condition.
Key Takeaways:
- A recursive loop is a programming technique where a function calls itself until a specific condition is met.
- Recursive loops are commonly used in algorithms, such as those involving tree or graph traversals.
Understanding the Mechanics of a Recursive Loop
Let’s break down how a recursive loop works in a step-by-step manner:
- The function starts executing with an initial input value.
- The function checks a base condition. If the condition is met, the function stops calling itself and returns a result.
- If the base condition is not met, the function calls itself again with a modified input value.
- The process repeats until the base condition is met.
- Each recursive call of the function creates a new instance of itself, allowing it to maintain a separate state and process different inputs.
- The final result is obtained by combining the results of each recursive call.
Real-Life Analogy: The Russian Nesting Dolls
To better understand recursion, let’s use a real-life analogy: the Russian nesting dolls, also known as Matryoshka dolls. These dolls come in a set, with each doll fitting inside a larger one.
Similarly, in a recursive loop, each function call acts like a smaller nested doll, containing its own set of instructions and calling itself with a modified input. This process continues until a base condition is met, just as the largest doll in the set contains no other dolls inside.
Applying Recursive Loops in Programming
Recursive loops provide elegant solutions to certain programming problems. Here are a few scenarios where recursive loops are commonly used:
- Tree and graph traversals: Recursive loops can efficiently traverse complex data structures, such as trees and graphs, by recursively exploring each node or vertex.
- Fibonacci sequence: The Fibonacci sequence is a classic example of recursion, where each number is calculated by summing up the two preceding numbers.
- Factorial calculations: Recursive loops can compute factorials, which involve multiplying a number by all smaller integers, by repeatedly calling the function with a decremented value.
- Maze solving: Recursive loops can be employed to solve mazes by exploring adjacent cells or paths until the exit is reached.
Key Takeaways:
- Recursive loops are a powerful programming technique used to solve specific problems efficiently.
- Understanding recursion is an essential skill for programmers, as it allows them to break down complex problems into simpler and more manageable steps.
Now that you have a good grasp of what a recursive loop is and how it works, you can start exploring and employing this fundamental programming concept in your own projects. Stay tuned for more exciting definitions in our “DEFINITIONS” series!