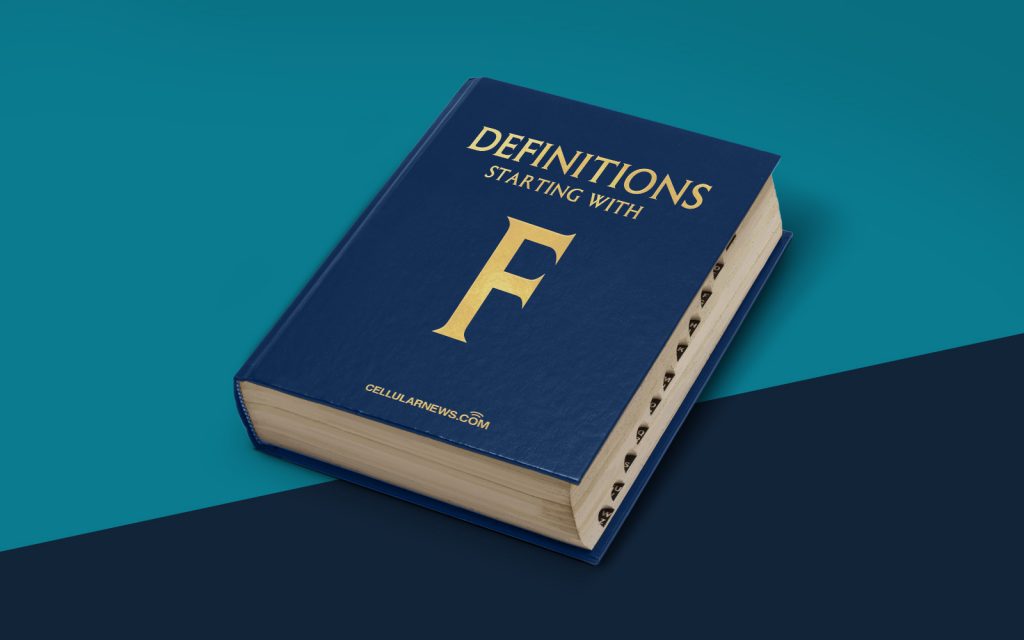
What is a For Loop?
Have you ever wondered what a for loop is and how it works? If you are new to programming or just curious about the concept, look no further! In this blog post, we will dive into the world of for loops and demystify its functionality. So, let’s get started!
The Definition
In programming, a for loop is a control structure that allows you to repeat a block of code a certain number of times. It’s an efficient way to automate repetitive tasks and iterate over a collection of elements. This loop consists of three main components:
- Initialization: Setting an initial value for the loop counter
- Condition: Evaluating a condition that defines the number of iterations
- Increment/Decrement: Modifying the loop counter after each iteration
Key Takeaways
- A for loop is a control structure used for repetitive tasks in programming.
- It consists of an initialization step, a condition to be evaluated, and an increment/decrement operation.
How Does it Work?
Let’s break down the components of a for loop and understand how they work together:
- Initialization: At the beginning of the loop, we set an initial value for the loop counter. This step is executed only once.
- Condition: The loop continues to execute as long as the condition evaluates to true. If the condition becomes false, the loop terminates.
- Code Block: The code within the loop is executed repeatedly until the condition is met.
- Increment/Decrement: After each iteration, the loop counter is modified according to the increment or decrement operation specified. This step allows the loop to advance or terminate the execution.
Example:
Let’s see a simple example to better understand how a for loop works:
<html>
<body>
<script>
for (let i = 1; i <= 5; i++) {
console.log("Iteration: " + i);
}
</script>
</body>
</html>
In this example, we have a for loop that starts with an initial value of i = 1. The loop will continue as long as i <= 5, printing the current iteration number to the console. After each iteration, the value of i will be incremented by 1. The loop will terminate when i becomes 6, as the condition becomes false. So, this loop will execute five times, printing the numbers 1 to 5 on the console.
Conclusion
In summary, a for loop is a powerful programming construct that allows you to repeat a block of code multiple times. It consists of an initialization step, a condition to be evaluated, a code block, and an increment or decrement operation. By understanding how for loops work, you can efficiently automate repetitive tasks and iterate over collections. So, the next time you encounter a for loop, you’ll know exactly what it does!