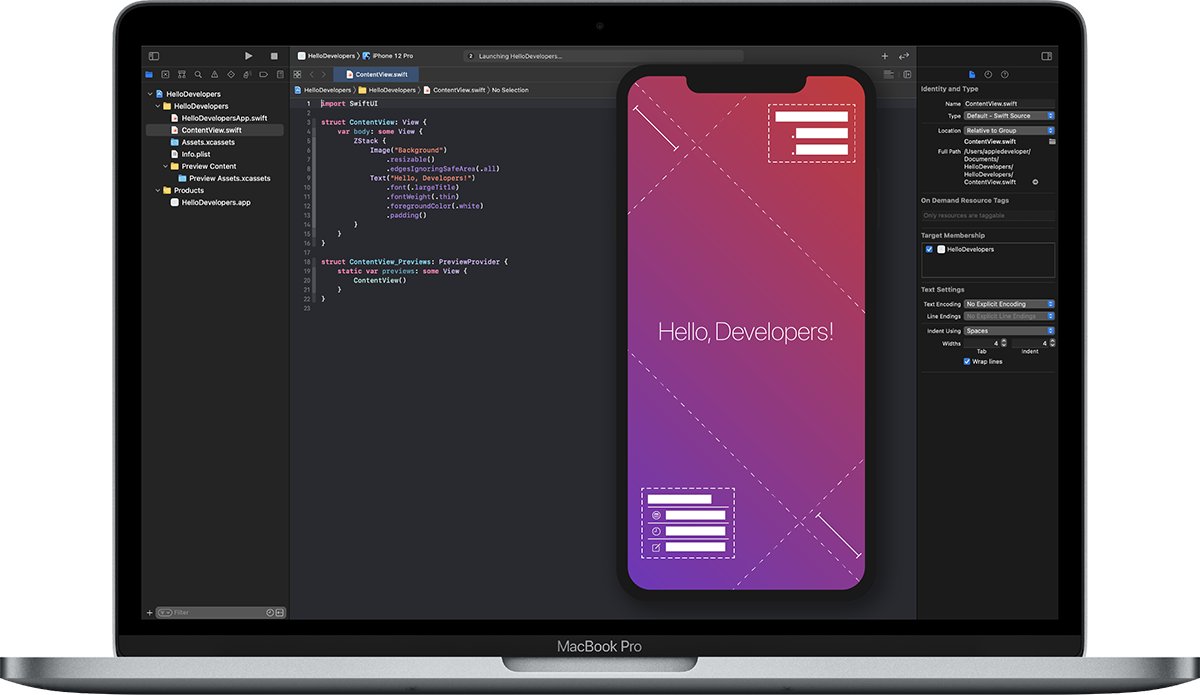
Are you ready to take your Swift programming skills to the next level? In this comprehensive guide, we will delve into the world of advanced collections in Swift. Collections are fundamental data structures that enable you to efficiently store, manage, and manipulate groups of data. From arrays and dictionaries to sets and tuples, you will learn how to use these powerful collection types to solve complex problems and optimize your code. Whether you’re a seasoned Swift developer or just starting out, this article will provide you with the knowledge and techniques needed to master advanced collections in Swift. So let’s dive in and unlock the full potential of Swift’s collection framework!
Inside This Article
Arrays
Arrays are a fundamental concept in programming and are widely used in Swift. They allow you to store and organize collections of values of the same type. Whether you need to create a list of numbers, strings, or any other data type, arrays are the go-to data structure.
In Swift, arrays are ordered collections, meaning the elements are stored in a particular sequence. This allows you to access elements by their index number, starting from zero. For example, if you have an array of names, you can access the first name using the index 0, the second name using the index 1, and so on.
To declare an array in Swift, you specify the type of data it will hold, followed by square brackets. Here’s an example:
swift
var numbers: [Int] = [1, 2, 3, 4, 5]
In the example above, we declare an array called “numbers” that will hold integers. We initialize it with five values: 1, 2, 3, 4, and 5.
You can also declare an empty array and populate it with data later, like this:
swift
var fruits: [String] = []
fruits.append(“apple”)
fruits.append(“banana”)
fruits.append(“orange”)
In this case, we declare an empty array called “fruits” that will hold strings. We then use the `append` method to add elements to the array.
Arrays in Swift provide various built-in methods and properties to manipulate and access their elements. You can use methods like `count` to get the number of elements in an array, `isEmpty` to check if an array is empty, and `first`/`last` to access the first/last element of the array, among others.
Another powerful feature of arrays in Swift is the ability to iterate over their elements using a loop. You can use a `for-in` loop to traverse the array and perform operations on each element:
swift
for number in numbers {
print(“Current number: \(number)”)
}
This loop will iterate over each element in the array “numbers” and print its value.
Additionally, Swift provides a shorthand notation called “array literals” that allows you to declare and initialize an array in a single line. Here’s an example:
swift
let weekdays = [“Monday”, “Tuesday”, “Wednesday”, “Thursday”, “Friday”]
In this case, we declare a constant array called “weekdays” and initialize it with the specified string values.
Overall, arrays are a versatile and essential tool in Swift programming. They allow you to store, access, and manipulate collections of data efficiently, making them a fundamental building block for many types of applications.
Sets
Sets are another essential collection type in Swift programming. A set is an unordered collection of unique values. It is especially useful when you need to track a list of items where each item should only appear once.
Let’s say you are building a mobile app that allows users to create playlists of their favorite songs. You want to ensure that each song is added to the playlist only once, regardless of how many times the user tries to add it. This is where sets come in handy.
To create a set, you use the `Set` keyword followed by angle brackets containing the type of the elements in the set. For example, if you want to create a set of integers, you would write:
var numberSet = Set
Alternatively, you can use the simplified syntax:
var numberSet: Set
Once you have created a set, you can add elements to it using the `insert(_:)` method and remove elements using the `remove(_:)` method. Sets also provide useful operations such as checking for containment, performing set operations like union, intersection, and difference, and iterating over the elements.
One important characteristic of sets is that they do not allow duplicate values. If you try to add an element that already exists in the set, it will be ignored. This ensures the uniqueness of the values stored in the set.
Here’s an example:
swift
var fruitSet: Set
fruitSet.insert(“Orange”) // Ignored because “Orange” already exists
print(fruitSet) // Output: [“Apple”, “Banana”, “Orange”]
fruitSet.remove(“Banana”)
print(fruitSet) // Output: [“Apple”, “Orange”]
Sets are a powerful tool when it comes to eliminating duplicates and efficiently performing operations on unique values. They can greatly simplify your code and improve its performance.
Now that you have an understanding of sets, let’s move on to the next topic: dictionaries.
Dictionaries
In the world of Swift programming, dictionaries play a crucial role in organizing and managing data. A dictionary is a collection that holds key-value pairs, where each key is unique and associated with a corresponding value. This data structure provides a powerful way to store and retrieve information.
Unlike arrays and sets, dictionaries do not rely on the order of elements. This means that rather than accessing values by their index, you retrieve them by using their corresponding key. This key-value relationship allows for efficient lookups and quick retrieval of data within a dictionary.
Creating a dictionary in Swift is straightforward. You start by declaring the type of both the key and value, and then initialize it with a set of key-value pairs enclosed in square brackets. For example:
swift
var capitalCities = [“USA”: “Washington D.C.”, “France”: “Paris”, “Japan”: “Tokyo”]
In this example, we have a dictionary called `capitalCities`, where each key represents a country and its associated value represents the capital city. The key-value pairs are separated by a colon (:), and each pair is separated by a comma.
You can access the values in a dictionary by using the subscript syntax, specifying the respective key within square brackets. For example, to retrieve the capital city of Japan from the `capitalCities` dictionary, you would write:
swift
let japanCapital = capitalCities[“Japan”]
The value returned would be “Tokyo”.
Swift provides various methods to modify dictionaries, such as adding and removing key-value pairs. To add a new entry to a dictionary, you can use the subscript syntax and assign a value to a key that does not yet exist. For example:
swift
capitalCities[“Germany”] = “Berlin”
This will add a new key-value pair to the `capitalCities` dictionary, with “Germany” as the key and “Berlin” as the value.
Alternatively, you can also use the `updateValue(_:forKey:)` method to add or update a key-value pair. This method returns the previous value associated with the key if it exists, or nil if it does not. For example:
swift
let previousValue = capitalCities.updateValue(“Ottawa”, forKey: “Canada”)
This method would update the value of the “Canada” key to “Ottawa” and return the previous capital city (“Tokyo”).
To remove a key-value pair from a dictionary, you can use the `removeValue(forKey:)` method. This method removes the entry with the specified key and returns the associated value, or nil if the key is not found. For example:
swift
let removedValue = capitalCities.removeValue(forKey: “France”)
This would remove the key-value pair for “France” and return “Paris”.
Dictionaries in Swift offer a powerful and flexible way to organize and access data. Whether you need to store information about countries and their capital cities or any other key-value relationship, dictionaries can efficiently handle the task. Understanding how to create, access, and modify dictionaries will enable you to leverage this fundamental Swift collection type to its fullest extent.
Optionals and Nil-Coalescing Operator
In Swift programming, optionals play a vital role when it comes to handling values that might be absent or have unknown states. Optionals provide a way to represent the absence of a value, which is particularly useful when dealing with variables that can be nil or uninitialized.
An optional variable is denoted by appending a question mark “?” after the type declaration. This indicates that the variable may or may not have a value. For example, if we declare a variable “age” of type Int as an optional:
swift
var age: Int?
Now, the “age” variable can either hold an actual integer value or it can be nil. To safely unwrap an optional variable and access its value, Swift provides optional binding using if-let statements. This allows you to check if the optional has a value and safely extract it in a single statement. Here’s an example:
swift
if let unwrappedAge = age {
print(“The age is \(unwrappedAge)”)
} else {
print(“The age is unknown”)
}
The if-let statement first checks if “age” has a value. If it does, it assigns the unwrapped value to the constant “unwrappedAge” and executes the code within the if block. If “age” is nil, the else block is executed.
However, there are cases where you may want to provide a default value in case the optional is nil. This is where the nil-coalescing operator comes into play. The nil-coalescing operator, denoted by “??”, allows you to provide a default value to be used if the optional is nil.
Here’s an example:
swift
let name: String? = “John”
let unwrappedName = name ?? “Guest”
print(“Welcome, \(unwrappedName)”)
In this example, if “name” has a value, it will be unwrapped and assigned to “unwrappedName”. If “name” is nil, the default value “Guest” will be assigned to “unwrappedName”. In either case, the unwrapped value is then used to print the welcome message.
The nil-coalescing operator can be a valuable tool to handle optionals more efficiently and provide meaningful default values when required. It simplifies code and reduces the need for additional if statements or optional binding.
By understanding the concept of optionals and mastering the use of the nil-coalescing operator, you can write more robust and concise Swift code that gracefully handles scenarios where values may or may not be present.
Conclusion
Learning about advanced collections in Swift programming can greatly enhance your skills and enable you to build more efficient and powerful applications. Throughout this article, we have explored various advanced collection types such as sets, dictionaries, and tuples, and delved into the operations and benefits they offer.
By leveraging advanced collections, you can handle complex data structures, improve the performance of your code, and leverage the unique features each collection type provides. Understanding how to effectively utilize these collections will give you greater flexibility and control in your Swift programming projects.
As you continue your journey in Swift programming, keep in mind the importance of choosing the right collection type for your specific needs. Whether it’s storing unique values, retrieving values based on keys, or working with multiple values as a single unit, the knowledge gained from mastering advanced collections will undoubtedly be a valuable asset in your programming toolkit.
So, don’t be afraid to dive deeper into the world of collections in Swift and unlock the full potential of your code!
FAQs
1. What are advanced collections in Swift programming?
Advanced collections refer to the specialized data structures in Swift that provide efficient storage and retrieval of data. They offer additional functionality and performance improvements over the basic collection types like arrays and dictionaries.
2. What are some examples of advanced collections in Swift?
Some examples of advanced collections in Swift include sets (unordered collections of unique values), tuples (groups of values combined into a single compound value), and generics (allowing the creation of reusable code).
3. How do advanced collections enhance Swift programming?
Advanced collections enhance Swift programming by providing more powerful and flexible options for data storage and manipulation. They enable developers to optimize performance, handle complex scenarios, and write more efficient and readable code.
4. Can advanced collections be customized or extended in Swift?
Yes, advanced collections can be customized and extended in Swift. Developers can define their own collection types by adopting and conforming to Swift protocols, such as the Collection or MutableCollection protocols, and implementing the required methods and properties.
5. Where can I find more information on advanced collections in Swift?
The official Swift documentation and Apple’s developer resources provide comprehensive information on advanced collections in Swift. You can also refer to online tutorials, forums, and community-driven resources for practical examples and best practices.