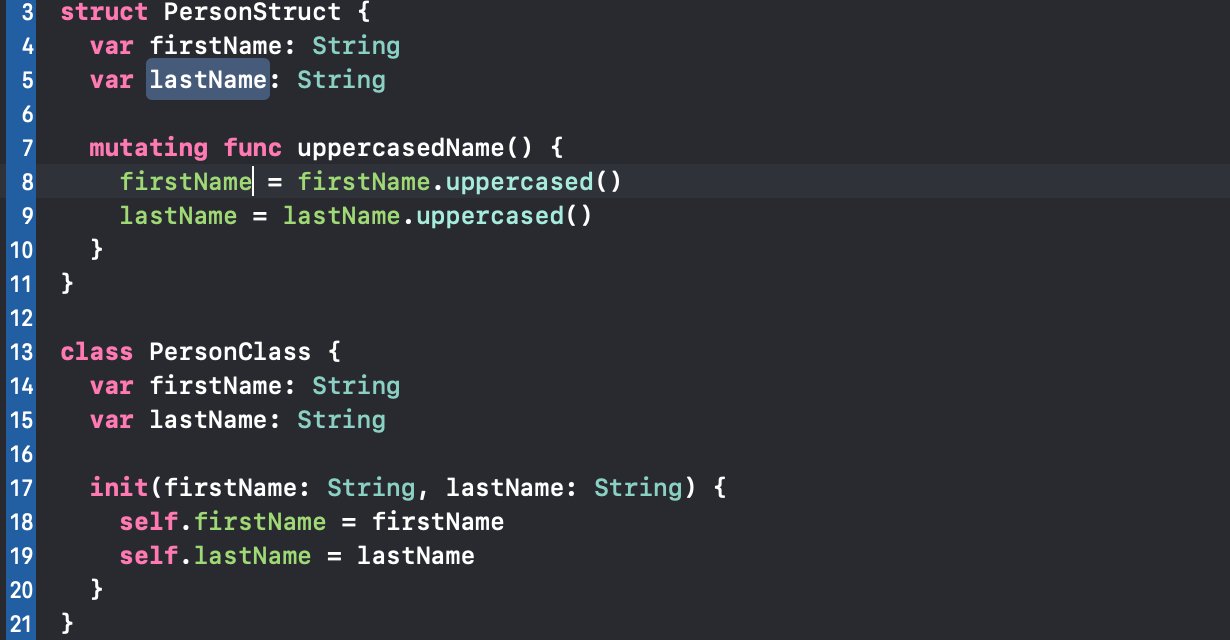
Welcome to “Swift 101 – Classes, Variables, Properties & Methods”! In this comprehensive guide, we will dive deep into the foundations of Swift, one of the most popular programming languages for iOS and macOS app development.
Understanding classes, variables, properties, and methods is crucial for any aspiring Swift developer. These concepts form the building blocks of object-oriented programming and play a significant role in structuring and manipulating data in Swift applications.
Whether you’re a beginner or looking to brush up on your Swift skills, this article will provide you with a solid understanding of classes, variables, properties, and methods in Swift. By the end, you’ll have the knowledge and confidence to start creating your own robust and efficient Swift applications.
Inside This Article
Classes
When it comes to object-oriented programming, classes are the foundation. A class is like a blueprint or a template that defines the structure and behavior of an object. It encapsulates data and functions together, allowing you to create multiple instances of the class, known as objects.
In Swift, creating a class is easy. You start by using the class
keyword followed by the name of the class. You can then define properties and methods inside the class to add functionality. Here’s an example:
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func sayHello() {
print("Hello, my name is \(name) and I am \(age) years old.")
}
}
In the example above, we created a class called Person with two properties: name
of type String
and age
of type Int
. We also have an init
method, which is called when creating a new instance of the class. The sayHello()
method allows the person object to introduce themselves.
To create an instance of a class, you simply use the class name followed by parentheses. You can then access the properties and methods of the object using dot notation. Here’s how you would use the Person class:
let john = Person(name: "John Doe", age: 25)
john.sayHello()
This will create a new Person object named John Doe with an age of 25 and then call the sayHello() method to introduce himself.
Classes are invaluable when it comes to organizing and structuring your code. They allow for code reuse and modularity, making it easier to manage large and complex projects. With classes, you can create objects that have their own unique state and behavior, enabling you to build powerful and flexible applications.
Variables
When it comes to programming, variables play a crucial role in storing and manipulating data. In Swift, variables are used to hold values that can be changed or updated throughout the execution of a program.
To declare a variable in Swift, we use the keyword var
followed by the name of the variable and its type. For example, we can declare a variable to store an integer value like this:
var num: Int
Here, we have declared a variable named num
of type Int
. The type annotation : Int
informs the compiler that the variable will store integers. However, we haven’t assigned any value to this variable yet.
Once a variable is declared, we can assign a value to it using the assignment operator (=
). For example:
num = 10
Here, the value 10
is assigned to the variable num
. Now, the variable holds the value 10
.
It’s worth noting that Swift is a strongly-typed language, which means that the type of a variable is checked during compilation and cannot be changed once it is assigned a specific type. In the example above, once we declare num
as an Int
, we cannot assign a value of a different type to it.
We can also declare and assign a value to a variable in a single line. This is called variable initialization. For example:
var age: Int = 25
In this case, we have declared a variable named age
of type Int
and assigned an initial value of 25
at the same time.
Variables in Swift can be modified by assigning a new value to them. For example, we can change the value of the age
variable like this:
age = 30
After this assignment, the variable age
now holds the value 30
.
Using variables allows us to store and manipulate data in our programs, making them dynamic and adaptable to changing conditions. Understanding variables and how to use them effectively is fundamental in Swift programming.
Properties
In Swift, properties are used to define values associated with a particular class, structure, or enumeration. They can be considered as variables or constants that can store data or values. Properties are essential for storing and accessing information within an object. Let’s explore more about properties and their usage in Swift.
Properties can be classified into two types: stored properties and computed properties.
1. Stored Properties: These properties store constant or variable values directly. They can be defined in classes or structures. Stored properties have various modifiers, such as public, private, or internal, which determine their visibility and accessibility.
2. Computed Properties: Unlike stored properties, computed properties don’t store values directly. Instead, they provide a getter and setter to calculate or derive a value based on other properties or data. Computed properties are declared with the { get }
and { set }
blocks.
Properties can also have property observers, which are used to observe and respond to changes in the property’s value. The property observer consists of two parts: willSet
and didSet
. The willSet
observer is called just before the value changes, and the didSet
observer is called immediately after the new value is set.
Let’s consider an example to understand properties better:
swift
class Circle {
var radius: Double
var area: Double {
return 3.14 * radius * radius
}
init(radius: Double) {
self.radius = radius
}
}
let myCircle = Circle(radius: 5.0)
print(“Area of the circle: \(myCircle.area)”) // Output: Area of the circle: 78.5
In the above example, the `Circle` class has two properties: `radius` and `area`. The `radius` property is a stored property that stores the value provided during initialization. The `area` property is a computed property that calculates the area of the circle based on the `radius` value.
Properties in Swift play a vital role in encapsulating data and providing access to it. They enable us to define and manage the state of an object effectively, making our code more modular and readable.
Now that we have understood the basics of properties, let’s move on to the next topic: methods.
Methods
In object-oriented programming, a method is a set of instructions or actions that can be performed on an object. It encapsulates a specific behavior or functionality of the class. Methods allow us to define reusable code blocks that can be called whenever needed.
Methods are defined within a class and are associated with objects created from that class. They can access and manipulate the data stored in the object’s properties. Methods can also receive parameters, enabling them to perform different actions based on the input received.
One of the key advantages of methods is code reusability. By defining methods, we can avoid repeating the same code in multiple parts of our program. Instead, we can call the method whenever we need to perform a specific set of actions. This promotes cleaner and more organized code.
Methods can have a return type, which specifies the type of value that the method will return once executed. If a method does not have a return type, it is commonly referred to as a void method. Void methods are used when we want to perform actions without expecting any return value.
To call a method, we use the object’s name followed by the method name and any required parameters. The method will then execute its set of instructions and may or may not return a value.
Methods can also be overloaded, which means we can define multiple methods with the same name but with different parameters. The compiler determines which method to execute based on the arguments provided during the method call.
By defining methods, we can break down complex tasks into smaller, more manageable components. This improves code readability, maintainability, and extensibility. It also allows for better code organization and modularity, making it easier to work on different parts of the program simultaneously.
Overall, methods are an essential concept in object-oriented programming as they enable us to define reusable behaviors and actions within our classes. They help promote code reusability and maintainability, making our programs more efficient and easier to work with.
Conclusion
With this comprehensive guide to Swift 101, you are now equipped with the knowledge to work with classes, variables, properties, and methods in Swift. Understanding these fundamental concepts lays a solid foundation for your journey as an iOS developer.
By mastering the concept of classes, you can create reusable and modular code, allowing you to build complex applications with ease. Variables and properties enable you to store and manipulate data, while methods allow you to define the behavior and functionality of your classes.
Remember, practice is key. Take the time to experiment and explore different scenarios. As you gain more experience, you will become more proficient in Swift, and your programming skills will continue to grow.
So, what are you waiting for? Start applying what you’ve learned and unleash your creativity in the world of iOS development with Swift!
FAQs
Q: What is the difference between a class and a variable?
A: A class is a blueprint or template for creating objects, while a variable is a placeholder that holds a value or a reference to an object. In simpler terms, a class defines the characteristics and behaviors of objects, whereas a variable is used to store data.
Q: What are properties in Swift?
A: Properties are the values associated with a class, structure, or enumeration. They can be variables (var) or constants (let) and store information specific to each instance of the class, structure, or enumeration. Properties can have default values, or they can be set and modified using getter and setter methods.
Q: How do I declare a variable in Swift?
A: To declare a variable in Swift, you use the var keyword, followed by the variable name and its type. For example, to declare an integer variable called “age”, you would write: var age: Int.
Q: What is the difference between a method and a function in Swift?
A: In Swift, methods and functions are both blocks of code that can be executed. However, methods are associated with a specific class, structure, or enumeration, and are called on instances of those types. Functions, on the other hand, are standalone blocks of code that can be called independently.
Q: How do I access properties and call methods in Swift?
A: To access properties and call methods in Swift, you use the dot notation. For example, if you have an instance of a class called “person”, and it has a property called “name”, you can access it with person.name. Similarly, if the class has a method called “sayHello”, you can call it using person.sayHello().