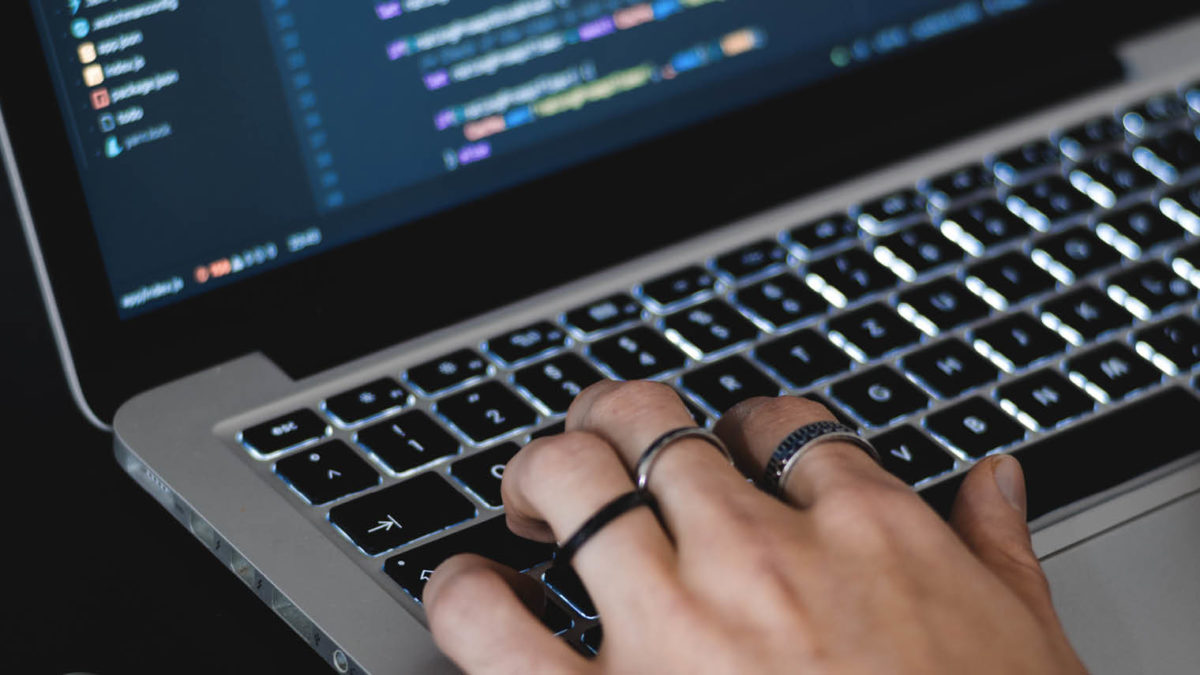
In the exciting world of Swift programming, understanding protocols and delegates is essential to becoming a master developer. Protocols provide a blueprint for defining methods, properties, and other requirements that a conforming class must implement. Delegates, on the other hand, allow objects to communicate with each other, enabling powerful encapsulation and separation of concerns.
In this comprehensive guide, we will dive deep into the world of protocols and delegates, exploring their roles, features, and best practices. Whether you are an experienced Swift programmer looking to solidify your understanding or a beginner eager to learn the fundamentals, this article is for you. Get ready to unlock the full potential of Swift by mastering protocols and delegates!
Inside This Article
- Understanding Protocols in Swift
- Implementing Protocols in Swift
- Working with Delegates in Swift
- Conclusion
- FAQs
Understanding Protocols in Swift
In the world of Swift programming, protocols play a vital role in defining a set of rules or guidelines that custom types can adopt. Protocols act as blueprints for what properties, methods, and requirements a conforming type should have. Think of protocols as contracts that specify the behavior and abilities that a type should exhibit.
Protocols define a list of methods, properties, and other requirements that a conforming type must adhere to. By adopting a protocol, a type promises to provide implementations for all the required elements declared within the protocol.
In Swift, protocol adoption is not limited to just classes. Structs, enums, and even other protocols can conform to a protocol. This flexibility makes protocols extremely powerful in promoting code reusability and enabling polymorphic behavior among different types.
Protocols can also include optional requirements, indicated by the `optional` keyword. This means that conforming types are not mandated to implement those optional requirements. It is up to the conforming type to decide whether or not to provide an implementation for them.
One of the key benefits of using protocols is that they allow for protocol-oriented programming, which is a design paradigm that focuses on creating flexible, reusable, and modular code. By defining protocols and structuring your code around them, you can easily swap and interchange different types that conform to the same protocol, without affecting the overall functionality of your code.
To define a protocol in Swift, you use the `protocol` keyword followed by the name of the protocol. Inside the protocol, you can declare properties, methods, and other requirements that conforming types must implement.
For example, let’s say we want to define a protocol called `Drawable` that represents an object that can be drawn. We can define this protocol with a `draw()` method requirement:
protocol Drawable {
func draw()
}
Any type that adopts the `Drawable` protocol must provide an implementation for the `draw()` method. This ensures that all conforming types can be drawn in a consistent way.
To adopt and conform to a protocol, a type must explicitly declare its intention by using the `:` symbol followed by the protocol name while declaring the type. For example:
struct Circle: Drawable {
func draw() {
// Implementation of draw method for Circle
}
}
In this example, the `Circle` struct adopts the `Drawable` protocol and provides an implementation for the `draw()` method. This allows an instance of `Circle` to be treated as a `Drawable` object, enabling it to be drawn as per the protocol’s requirements.
Understanding protocols in Swift is essential for building modular and reusable code. By adopting protocols, you can define a contract that different types can adhere to, promoting code flexibility and facilitating code reuse.
Implementing Protocols in Swift
In Swift, protocols play a crucial role in defining the blueprint for methods, properties, and other requirements that a class or structure must adhere to. To implement a protocol in Swift, you simply need to adopt it in your class or structure declaration.
Let’s say we have a protocol called ‘Animal’ with a method called ‘makeSound’. To implement this protocol, you would write:
swift
class Dog: Animal {
func makeSound() {
// Implement the sound behavior for a dog
print(“Woof!”)
}
}
In the example above, the ‘Dog’ class adopts the ‘Animal’ protocol by using the colon ‘:’ notation. It then provides the implementation for the ‘makeSound’ method by printing “Woof!”.
Note that a class or structure can adopt multiple protocols at the same time. This allows for a flexible and modular design, as you can mix and match protocols based on the specific requirements of your application.
When implementing protocols, it’s important to ensure that all required methods and properties are implemented in the conforming class or structure. Failure to do so will result in a compilation error.
Additionally, Swift provides the ‘optional’ keyword for marking methods or properties in a protocol as optional. This means that conforming classes or structures can choose whether or not to implement these optional requirements.
For example, let’s extend our ‘Animal’ protocol with an optional method called ‘eatFood’:
swift
@objc protocol Animal {
func makeSound()
@objc optional func eatFood()
}
class Dog: Animal {
func makeSound() {
print(“Woof!”)
}
// ‘eatFood’ method can be implemented optionally
}
In the example above, the ‘eatFood’ method is marked as optional using the ‘@objc optional’ syntax. The ‘Dog’ class then chooses not to implement this optional requirement.
Overall, implementing protocols in Swift allows for greater code reuse, modularity, and flexibility. By defining a set of requirements through protocols, you can create classes and structures that can seamlessly work together in your Swift applications.
Working with Delegates in Swift
Delegation is a key concept in Swift programming, allowing one object to communicate and pass data to another object. Delegates act as intermediaries, handling tasks and callbacks on behalf of their delegating objects. They provide a mechanism for objects to interact and respond to events in a flexible and modular way.
In Swift, delegates are typically implemented using protocols. A protocol defines a set of methods and properties that a class or struct should adhere to. By conforming to a protocol, an object can become a delegate and provide the necessary implementations for the delegate methods.
To establish a delegate relationship, the delegating object needs a delegate property that conforms to the specified protocol. This property is usually weak to prevent strong reference cycles and memory leaks.
Once the delegate relationship is set up, the delegating object can call the delegate methods at appropriate times. These methods allow the delegate to perform custom logic, handle events, or retrieve data from the delegating object.
Delegates are commonly used in various scenarios, such as handling button taps, tableView or collectionView events, text input validation, and network request callbacks. They enable the separation of concerns and promote reusable and maintainable code.
When working with delegates, it’s important to follow the delegation pattern and adhere to the conventions set by the protocol. The delegating object should inform the delegate when an event occurs, but it should not dictate how the delegate handles the event. The delegate has the freedom to choose the appropriate action based on the specific context.
To implement delegates in Swift, start by defining a protocol that outlines the required methods and properties. Then, create a delegate property in the delegating object, and assign the delegate to another object that conforms to the protocol. Finally, call the delegate methods when needed.
Using delegates can greatly enhance the flexibility and extensibility of your Swift code. It allows for loose coupling between objects, making it easier to modify and extend functionality without directly modifying the existing codebase.
By leveraging the power of protocols and delegates, you can create highly modular and customizable applications in Swift, promoting code reuse and maintainability.
Conclusion
In conclusion, mastering protocols and delegates in Swift programming is essential for becoming a proficient iOS developer. Understanding how to use protocols allows you to define a set of methods and properties that can be adopted by different classes, promoting code reusability and flexibility. Delegates, on the other hand, enable the communication between objects by providing a way for one object to send messages to another.
By harnessing the power of protocols and delegates, you can build modular and decoupled code that is easier to maintain and extend. Additionally, protocols and delegates enable you to implement powerful design patterns such as the delegation, observer, and MVC patterns.
Continuing to explore and experiment with protocols and delegates will expand your horizons as a Swift developer and open up new possibilities for creating robust and scalable iOS applications. So don’t hesitate to dive into this powerful feature of the Swift language and take your programming skills to the next level!
FAQs
1. What is a protocol in Swift programming?
A protocol in Swift is a blueprint of methods, properties, and other requirements that can be adopted by a class, struct, or enum. It defines a set of rules or interface that a type must adhere to. By conforming to a protocol, a type promises to implement all the required methods and properties defined by that protocol.
2. What is a delegate in Swift programming?
A delegate is a design pattern used in Swift programming that allows one object to communicate and interact with another object. It defines a protocol with methods and properties that the delegate object can implement to provide its functionality. By adopting the delegate protocol, an object can delegate tasks or pass information to another object.
3. How do you declare and adopt a protocol in Swift?
To declare a protocol in Swift, use the `protocol` keyword followed by the protocol name. Inside the protocol, you can define required and optional methods, properties, and other requirements. To adopt a protocol, a class, struct, or enum must conform to it by implementing all the required methods and properties. Use the `class` keyword for class-only protocols, and use the `protocolName: DelegateProtocol` syntax to set the delegate property and assign the delegate object.
4. Can a class adopt multiple protocols in Swift?
Yes, a class in Swift can adopt multiple protocols by separating them with a comma. This allows the class to conform to the requirements of multiple protocols and inherit their functionality. This flexibility enables classes to incorporate various features and behaviors from different protocols, enhancing code modularity and reusability.
5. How are protocols different from inheritance in Swift?
Protocols and inheritance are both mechanisms to define a set of rules and requirements for a type. However, they serve different purposes. Inheritance is used to create a hierarchy of classes where subclass inherits the properties and methods of its superclass, while protocols are used to define a set of rules that any type can conform to. A class can inherit from only one superclass, but can adopt multiple protocols. Protocols provide a more flexible approach to code reuse and composition.