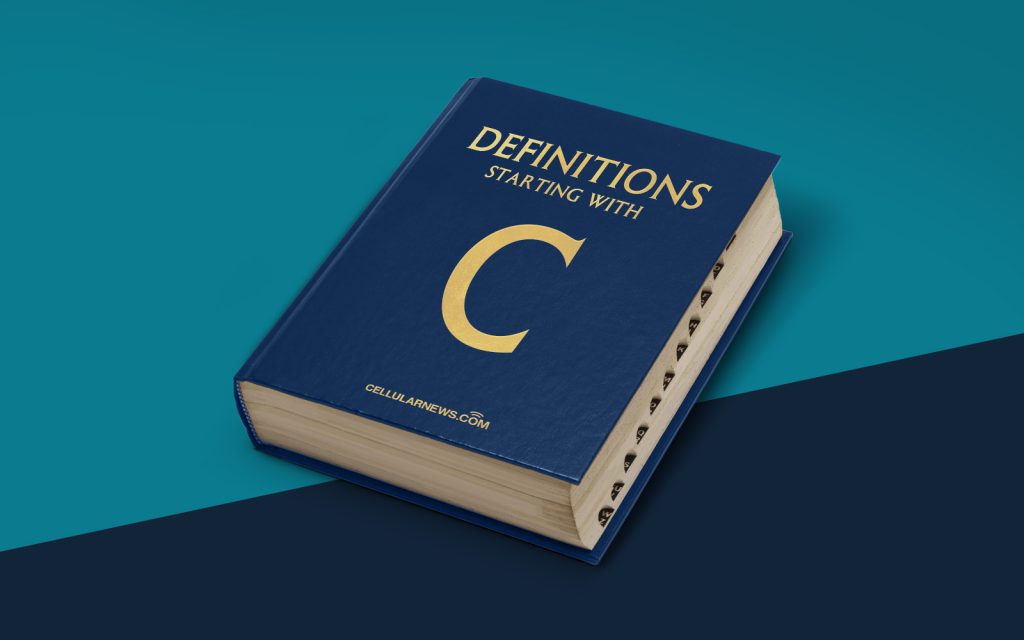
Welcome to the “DEFINITIONS” category on our page! In this blog post, we’ll be diving into the world of programming and taking a closer look at the concept of a Catch block. So, what exactly is a Catch block?
Essentially, a Catch block is a fundamental component of error handling in programming languages such as Java, C++, and C#. It is used to catch and handle exceptions that may occur during the execution of a program, allowing for a graceful way to deal with potential errors.
Key Takeaways:
- A Catch block is an essential part of error handling in programming languages.
- It is used to catch and handle exceptions that may occur during program execution.
Now, let’s take a closer look at how a Catch block works and its role in handling exceptions.
When a program encounters an exception, it throws an exception object. This object contains information about the exception, such as its type and message. The Catch block is responsible for catching this exception and executing the code within its scope to handle the specific exception.
Here’s a simplified example to illustrate how a Catch block functions:
try { // Code that may throw an exception } catch(ExceptionType exception) { // Code to handle the exception }
In the above code snippet, the try block contains the code that might cause an exception. If an exception occurs, the Catch block is triggered, and the code within the block is executed. The exception object is then passed to the Catch block, allowing the programmer to handle the exception accordingly.
It’s worth noting that Catch blocks can be used to handle specific exceptions or a broader range of exceptions. By specifying the type of exception within the parentheses after the “catch” keyword, you can define which exceptions will be caught by that particular Catch block.
Additionally, multiple Catch blocks can be used within a single code block to handle different types of exceptions. This allows for more precise error handling and enables programmers to handle various exceptions in different ways.
Key Takeaways:
- Catch blocks are used to catch exceptions that occur during program execution.
- They handle exceptions by executing code within their scope.
In conclusion, a Catch block is a vital component of error handling in programming languages. It plays a crucial role in catching and handling exceptions, allowing for more robust and reliable programs. By using Catch blocks effectively, programmers can gracefully handle errors and ensure the smooth execution of their applications.
We hope this article has provided you with a clearer understanding of what a Catch block is and how it functions. Stay tuned for more informative posts in our “DEFINITIONS” category!