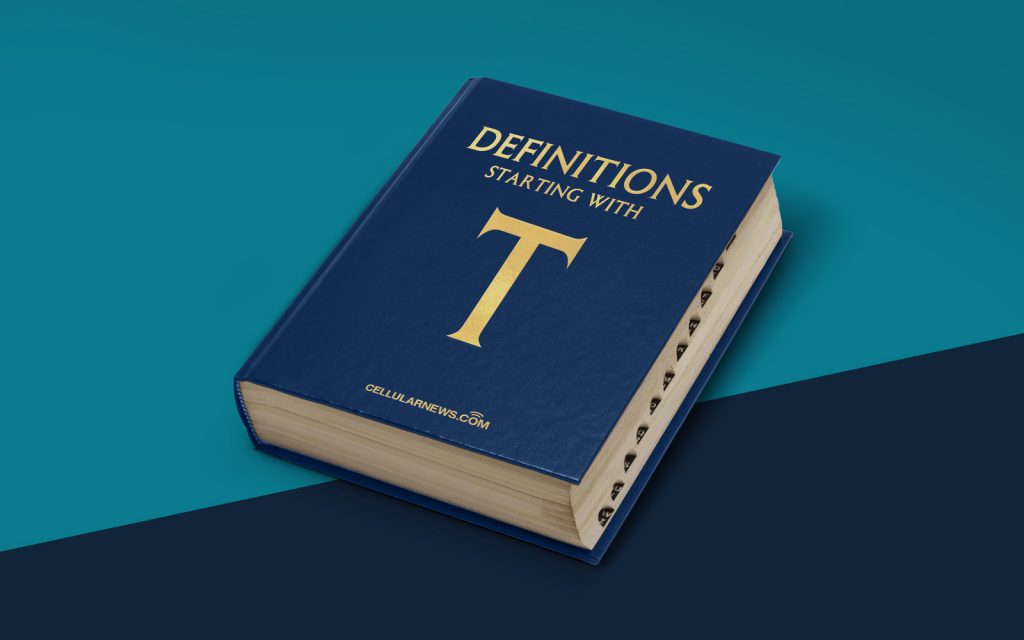
What is a Try/Catch Block?
Welcome to our “Definitions” blog series, where we break down complex programming concepts and deliver them in a way that’s easy to understand. In today’s article, we’re diving into the world of try/catch blocks, a fundamental component of error handling in many programming languages.
Have you ever encountered an error while running your code and wondered how to handle it? That’s where try/catch blocks come in handy! They allow developers to identify and manage errors gracefully, ensuring that the program can continue running smoothly even when unexpected issues arise.
Key Takeaways:
- A try/catch block is a section of code that is used for exception handling in programming.
- The try block contains the code that might throw an exception, while the catch block handles the exception and performs any necessary error handling.
How Does a Try/Catch Block Work?
When a try/catch block is implemented, the code contained within the try block is executed. If an exception occurs during this execution, the catch block immediately takes over and executes any necessary error handling code, preventing the program from crashing.
Let’s break down the components of a try/catch block:
- Try Block: This is where the code that could potentially throw an exception resides. It is surrounded by the “try” keyword.
- Catch Block: This block is executed when an exception occurs in the try block. It is preceded by the “catch” keyword, followed by the specific exception type that it can handle. Multiple catch blocks can be used to handle different types of exceptions.
- Exception: An exception is an event that occurs during the execution of a program and disrupts its normal flow. It can be caused by various factors such as invalid input, a network error, or a system failure.
Here’s an example of a try/catch block in JavaScript:
try {
// Code that might throw an exception
} catch (error) {
// Error handling code
}
Within the catch block, you can include code to display an error message, log the error, or take any necessary actions to correct the issue. By properly handling exceptions, you can enhance the stability and reliability of your code.
Conclusion
A try/catch block is a vital tool for handling exceptions in programming. It allows developers to anticipate and respond to errors, ensuring that their code can continue running smoothly even when unexpected issues arise. By implementing try/catch blocks effectively, you can provide a better user experience and improve the overall stability of your applications.
So the next time you encounter an error in your code, remember the power of the try/catch block and let it help you navigate the challenges of programming!