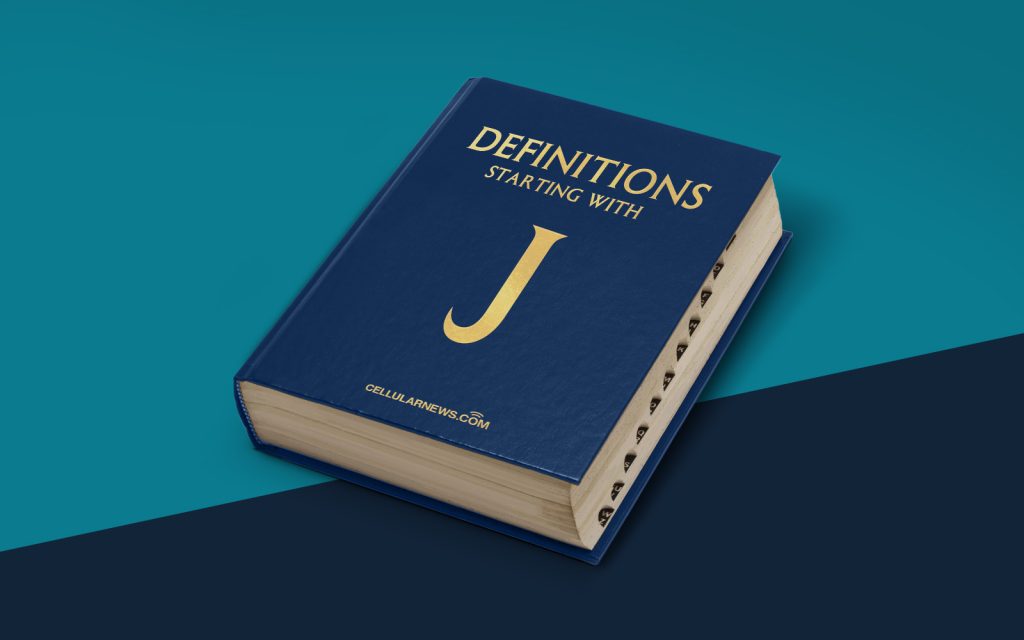
Understanding Java Objects
Welcome to another installment of our “DEFINITIONS” series, where we dive deep into the world of programming and explore the fundamental concepts that every developer should know. In this post, we’ll be shedding light on the ever-important topic of Java objects. So, grab your coding hats and let’s embark on this exciting journey!
Key Takeaways:
- A Java object is an instance of a class that encapsulates both data and behavior.
- Objects can be created using the ‘new’ keyword and can communicate with each other through methods.
In the world of Java programming, objects are the building blocks of applications. But what exactly is a Java object? In simple terms, an object is an instance of a class that encapsulates both data and behavior. It can be thought of as a real-world entity that has attributes (data) and actions (methods) associated with it.
Imagine you have a class called ‘Person’ that represents a person’s information such as name, age, and occupation. An object of the ‘Person’ class would then represent an actual person with specific values for those attributes.
In Java, objects are created using the ‘new’ keyword followed by the class name, like this: Person person = new Person(); This line of code instantiates a new object of the ‘Person’ class and assigns it to the variable ‘person’.
Once an object is created, you can access its attributes and behavior using dot notation. For example, if the ‘Person’ class has a method called ‘getAge()’, you can call it on the ‘person’ object like this: int age = person.getAge(); This allows you to retrieve the age value from the ‘person’ object.
Objects in Java can also communicate with each other through methods. Let’s say you have another class called ‘Friend’ that has a method called ‘greet()’. The ‘Person’ object can call this ‘greet()’ method on the ‘Friend’ object to initiate a greeting. This allows for interactions and collaborations between different objects in your code.
It’s important to understand that objects in Java have a state and behavior. The state is represented by the data stored in the object’s attributes, while the behavior is defined by the methods that operate on that data. This combination of state and behavior makes Java objects versatile and powerful tools for building complex applications.
So, the next time you’re coding in Java, remember that objects are at the heart of it all. They bring your code to life, allowing you to create dynamic and interactive applications that can solve real-world problems.
Key Takeaways:
- A Java object is an instance of a class that encapsulates both data and behavior.
- Objects can be created using the ‘new’ keyword and can communicate with each other through methods.