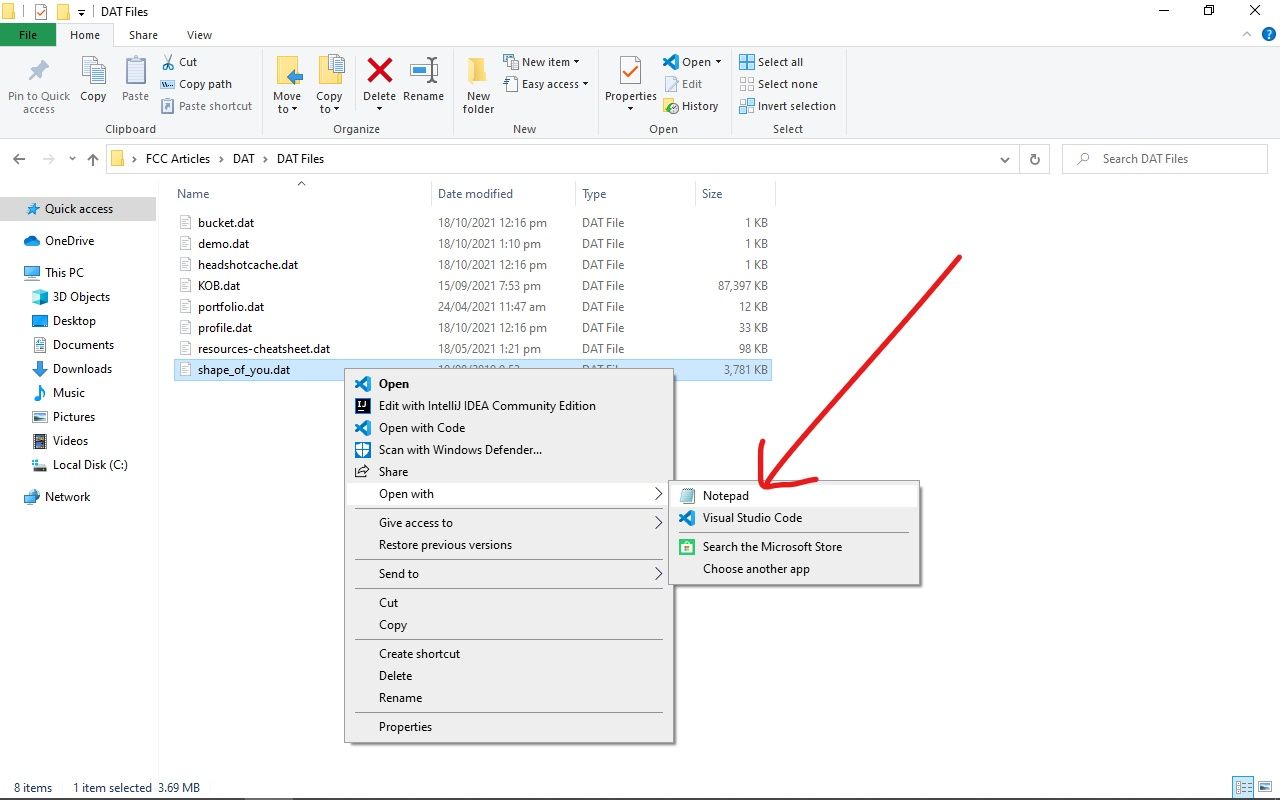
Are you a Java developer looking to read data from a .dat file? You’ve come to the right place! In this article, we’ll dive into the world of Java I/O operations and show you how to efficiently read data from a .dat file using Java. Reading data from a .dat file can be quite useful when working with structured data, like records or serialized objects. We’ll walk you through the step-by-step process of opening a .dat file, reading the data, and performing any necessary data conversion or manipulation along the way. So, whether you’re a beginner or an experienced programmer, let’s get started on this Java journey and unlock the secrets of reading data from a .dat file!
Inside This Article
- File Handling in Java
- Reading a .dat File in Java
- Steps to Read Data from a .dat File in Java
- Example: Reading Data from a .dat File in Java
- Conclusion
- FAQs
File Handling in Java
File handling is an essential aspect of programming in Java. It allows you to read and write data to and from files on the local file system. Java provides several classes and methods that facilitate file handling operations, making it easier for developers to handle data stored in files.
With file handling in Java, you can create, open, read, write, and close files. This functionality is particularly useful when you need to store and retrieve data for your applications, such as configuration files, databases, or any other external data sources.
Java provides a rich set of classes in the java.io package for file handling. These classes enable you to perform various operations on files, including creating new files, checking file existence, reading from files, writing to files, and deleting files.
When working with file handling in Java, it is important to understand key concepts such as file streams, byte streams, and character streams. File streams are used to transfer data between the device and the file system. Byte streams are used to read or write binary data, while character streams are used for reading or writing text data.
File handling in Java follows a simple process: open the file, perform the necessary operations, and close the file. By following this process, you ensure that the resources associated with the file are properly released, preventing memory leaks and other issues.
Now that you have an understanding of file handling in Java, it’s time to learn how to read data from a .dat file in Java. Keep reading to find out the steps involved!
Reading a .dat File in Java
Reading a .dat file in Java involves retrieving data stored in a .dat file and processing it in your Java program. The .dat file format is commonly used to store binary data, such as serialized objects or other complex data structures.
Java provides various classes and methods to handle file input and output operations. To read data from a .dat file, you can utilize the FileInputStream and ObjectInputStream classes, which are part of the java.io package.
The FileInputStream class allows you to open a connection to a .dat file and read the contents as a stream of bytes. The ObjectInputStream class, on the other hand, enables you to read Java objects from the binary data in the .dat file.
Here are the steps to read data from a .dat file in Java:
- Create an instance of the FileInputStream class and pass the file path or file object as a parameter.
- Create an instance of the ObjectInputStream class and pass the FileInputStream object as a parameter.
- Use the ObjectInputStream’s
readObject()
method to read the objects from the .dat file. - Catch any exceptions that may occur during the reading process and handle them appropriately.
- Close the ObjectInputStream and FileInputStream objects to release system resources.
Here’s an example of how to read data from a .dat file in Java:
import java.io.FileInputStream;
import java.io.ObjectInputStream;
public class DataReader {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("data.dat");
ObjectInputStream ois = new ObjectInputStream(fis);
// Read data from the .dat file
Object data = ois.readObject();
// Process the data
ois.close();
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Make sure to replace “data.dat” with the actual path or name of your .dat file in the FileInputStream initialization.
By following these steps, you can successfully read the data stored in a .dat file using Java. Remember to handle exceptions and close the input streams properly to maintain good programming practices.
Steps to Read Data from a .dat File in Java
Reading data from a .dat file in Java involves several steps. By following these steps, you can easily read and process data stored in a .dat file using Java programming language.
Step 1: Create a File Object
The first step is to create a file object that represents the .dat file you want to read. You can do this by providing the file path as a parameter to the File class constructor.
Step 2: Create an InputStream Object
Next, you need to create an InputStream object to read the data from the .dat file. InputStream is an abstract class that provides a stream of bytes. You can use the FileInputStream class, which is a subclass of InputStream, to read data from a file.
Step 3: Create a DataInputStream Object
To read data in a specific format from the .dat file, you need to create a DataInputStream object. DataInputStream is a class that provides the necessary methods to read different data types, such as integers, floats, and strings, from the input stream.
Step 4: Open the .dat File
You need to open the .dat file for reading using the created InputStream object. This is typically done by calling the open() method on the InputStream object.
Step 5: Read and Process the Data
Now, you can start reading and processing the data from the .dat file. Use the methods provided by the DataInputStream class to read the data in the desired format. For example, you can use the readInt() method to read an integer from the input stream.
Step 6: Close the .dat File
After you have finished reading the data from the .dat file, it is important to close the file to free up system resources. You can do this by calling the close() method on the DataInputStream object.
By following these steps, you can successfully read data from a .dat file in Java. Remember to handle any exceptions that may occur during the file handling process to ensure a smooth execution of your program.
Example: Reading Data from a .dat File in Java
Now let’s take a look at an example of reading data from a .dat file in Java. Suppose we have a .dat file called “data.dat” that contains information about employees in a company. Each line in the file represents the details of an employee, with the data separated by commas.
Here’s a sample content of the “data.dat” file:
John Smith, Engineering, 5000 Jane Doe, Marketing, 4500 Michael Johnson, Sales, 4000 Emily Wilson, HR, 5500
Now, let’s write a Java program to read the data from this .dat file and display it on the console:
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ReadDataFromDatFile { public static void main(String[] args) { try { File file = new File("data.dat"); Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); String[] data = line.split(","); String name = data[0]; String department = data[1]; int salary = Integer.parseInt(data[2]); System.out.println("Name: " + name); System.out.println("Department: " + department); System.out.println("Salary: " + salary); System.out.println(); } scanner.close(); } catch (FileNotFoundException e) { System.out.println("File not found."); } } }
In this example, we first create a File object representing the “data.dat” file. We then create a Scanner object and pass the File object as an argument to the Scanner constructor to read data from the file. We use the hasNextLine() method to check if there is another line to read, and then use the nextLine() method to read the current line.
We split the line using the split() method, specifying “,” as the delimiter, which separates the data into an array. We then extract the relevant data from the array and display it on the console.
After reading each employee’s details, we print an empty line to create a separation between the different records. Finally, we close the Scanner object to release any resources associated with it.
By executing this program, we will be able to read and display the employee data from the “data.dat” file in a structured manner on the console.
Conclusion
In conclusion, learning how to read data from a .dat file in Java can be a valuable skill for any programmer. By using the appropriate classes and methods, you can easily retrieve and manipulate data stored in these files. The java.io package provides various classes like FileInputStream, DataInputStream, and ObjectInputStream that can be used to read data from a .dat file.
Throughout this article, we have covered the essential steps involved in reading data from a .dat file, including opening the file, creating the appropriate input stream, and using the read methods to extract the data. We have also discussed how to handle exceptions and properly close the file and streams to ensure efficient and error-free file handling.
By familiarizing yourself with the concepts and techniques discussed in this article, you can confidently work with .dat files in your Java applications. So, start exploring, experimenting, and applying this knowledge to enhance your coding skills and develop robust file handling capabilities in Java.
FAQs
1. Can I read data from a .dat file in Java?
Yes, you can read data from a .dat file in Java using various methods and classes available in the Java I/O package. These classes provide the necessary functionality to read and manipulate binary data from a .dat file.
2. How do I open and read a .dat file in Java?
To open and read a .dat file in Java, you can use the FileInputStream class to create an input stream and read the data byte by byte or using a buffer. You can also use the ObjectInputStream class to read serialized objects from a .dat file.
3. What is the difference between reading binary and text files in Java?
When reading binary files in Java, you work with the raw data stored in the file, which consists of bytes that directly represent the information. On the other hand, when reading text files, Java provides high-level abstractions like BufferedReader that handle character encoding, buffering, and line-by-line reading, making it easier to process text-based content.
4. How can I read data from a specific location in a .dat file?
To read data from a specific location in a .dat file, you can use the seek() method provided by the RandomAccessFile class. This method allows you to set the file pointer to a specific position in the file, enabling you to read data from that location.
5. Is it possible to modify the data in a .dat file while reading it?
Yes, it is possible to modify the data in a .dat file while reading it. By using classes such as RandomAccessFile, you can read and write data at a specific position in the file simultaneously. However, it is important to be cautious when making modifications to ensure data integrity and avoid unintended consequences.