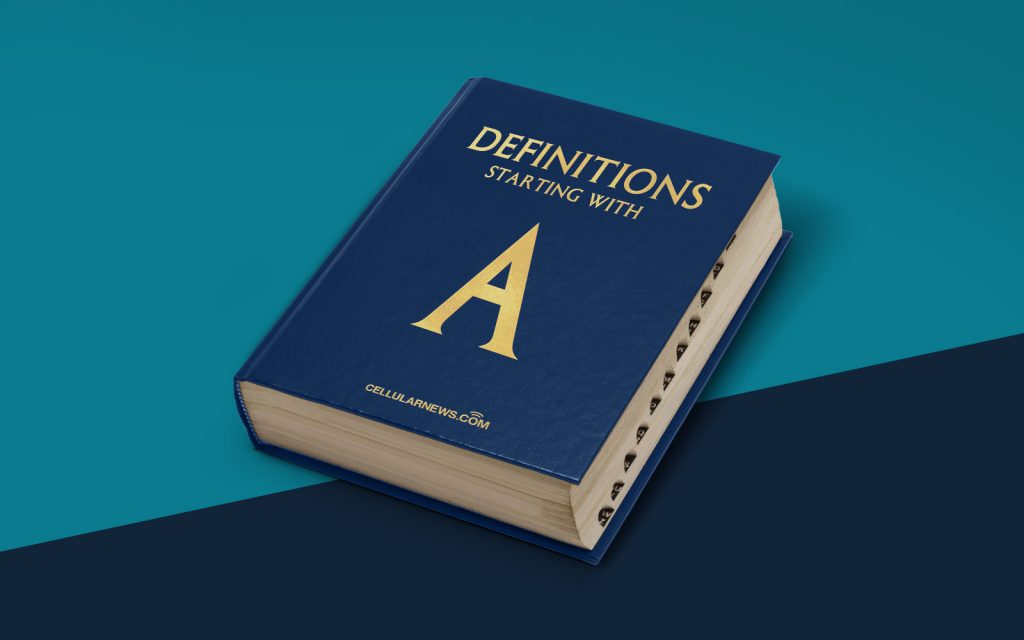
What is an Array in Java?
Welcome to another post in our “DEFINITIONS” category, where we explore important concepts and terms related to Java programming. In this blog post, we’ll be diving into the fascinating world of arrays in Java. If you’ve ever wondered how to store multiple values of the same type in a single variable, then you’re in the right place!
Key Takeaways:
- An array in Java is a collection of elements of the same type, stored in contiguous memory locations.
- Arrays have a fixed length, defined at the time of their creation, and can store values of primitive data types or objects.
Understanding Arrays
Imagine you have a collection of numbers and you want to store them together in a single variable. Without arrays, you would need to declare multiple variables, which quickly becomes cumbersome and inefficient. This is where arrays come to the rescue!
An array in Java is like a container that can hold multiple elements of the same type. It provides a way to store and access a group of related values in a more organized and efficient manner.
Arrays have a specific length, which is defined at the time of their creation and cannot be changed afterwards. The elements within an array are stored in contiguous memory locations, which means they can be accessed using their index.
Suppose you have an array named numbers that stores a series of integers. You can access each element of the array using its corresponding index. The first element has an index of 0, the second element has an index of 1, and so on. This allows you to perform operations on individual elements of the array or iterate over the entire collection.
Declaring and Initializing an Array
To declare an array in Java, you need to specify the data type of its elements, followed by square brackets ([]) to indicate that it is an array. Here’s an example of how to declare an array of integers:
int[] numbers;
After declaring an array, you need to initialize it by assigning values to its elements. There are two popular ways to initialize an array:
- Static Initialization: You can assign values to each element of the array at the time of its declaration. For example:
int[] numbers = {1, 2, 3, 4, 5};
- Dynamic Initialization: You can assign values to the array elements using a loop or any other mechanism during the program execution. This allows you to change the array’s content dynamically. For example:
int[] numbers = new int[5]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
It’s important to note that when you initialize an array, you must ensure that the number of elements matches the array’s declared length. Otherwise, you will encounter an ArrayIndexOutOfBoundsException at runtime.
Benefits of Using Arrays
Arrays offer several advantages in Java programming:
- Easy Access: Arrays allow for easy access to individual elements using their index values.
- Efficient Memory Usage: Arrays store elements in contiguous memory locations, making them highly memory-efficient.
- Iterating Over Elements: Arrays provide a convenient way to iterate over a collection of elements using loops.
- Performance Optimization: Arrays can be optimized for performance in certain scenarios, as they offer constant-time access to elements.
By familiarizing yourself with arrays in Java, you gain an essential tool for organizing and manipulating data in your programs. Whether you’re working with a small collection or a large dataset, arrays can help you streamline your code and make it more efficient.
We hope this blog post has provided you with a solid understanding of what arrays are in Java and why they are useful. Stay tuned for our next post in the “DEFINITIONS” category, where we’ll continue exploring important concepts in Java programming!