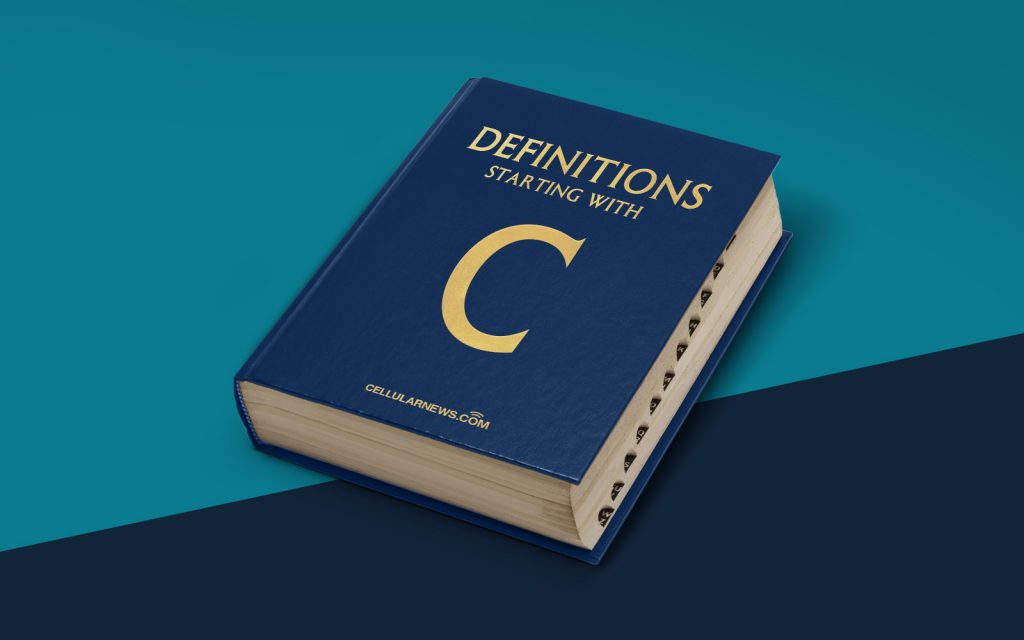
What is a Conditional Operator?
Welcome to the “DEFINITIONS” category of our blog! Today, we’re going to dive deep into one of the often misunderstood concepts in programming: the Conditional Operator. If you’re a developer or someone interested in learning about coding, you might have come across the term but aren’t quite sure what it means. In this blog post, we’ll demystify the Conditional Operator and explain its significance in programming.
Key Takeaways:
- The Conditional Operator, also known as the ternary operator, is a shorthand notation for an if-else statement in programming.
- It evaluates a condition and returns one value if the condition is true, and another value if the condition is false.
Imagine you are writing code and need to perform different actions based on a certain condition. Instead of using an if-else statement that spans multiple lines and adds complexity to your code, you can use the Conditional Operator to achieve the same result in a concise and efficient manner. It allows you to make decisions based on a condition in a single line of code, making your program more readable and reducing unnecessary clutter.
The Conditional Operator takes the form of condition ? value_if_true : value_if_false, where:
- condition is the expression being evaluated. It can be anything that returns a boolean value (true or false).
- value_if_true is the value that will be returned if the condition evaluates to true.
- value_if_false is the value that will be returned if the condition evaluates to false.
Let’s look at an example to solidify our understanding:
int age = 18;
string message = (age >= 18) ? "You are an adult" : "You are a minor";
In the above code snippet, we assign the value of “You are an adult” to the variable “message” if the condition “age >= 18” is true. Otherwise, the value “You are a minor” will be assigned.
Now, you might be wondering why use the Conditional Operator when we can accomplish the same thing with an if-else statement? The answer lies in the simplicity and compactness that the Conditional Operator provides. It condenses multiple lines of code into a single concise line, making it easier to understand and maintain.
Here are two key takeaways to remember about the Conditional Operator:
- It is a shorthand notation for if-else statements, reducing code complexity and enhancing readability.
- Keep in mind that the use of the Conditional Operator should not sacrifice code clarity. It is best suited for simple conditions rather than complex logical evaluations.
In conclusion, the Conditional Operator is a valuable tool in programming that simplifies decision-making processes. It allows you to write cleaner and more efficient code, enhancing both your productivity and the readability of your programs. So the next time you encounter a situation where a simple decision needs to be made based on a condition, give the Conditional Operator a try!