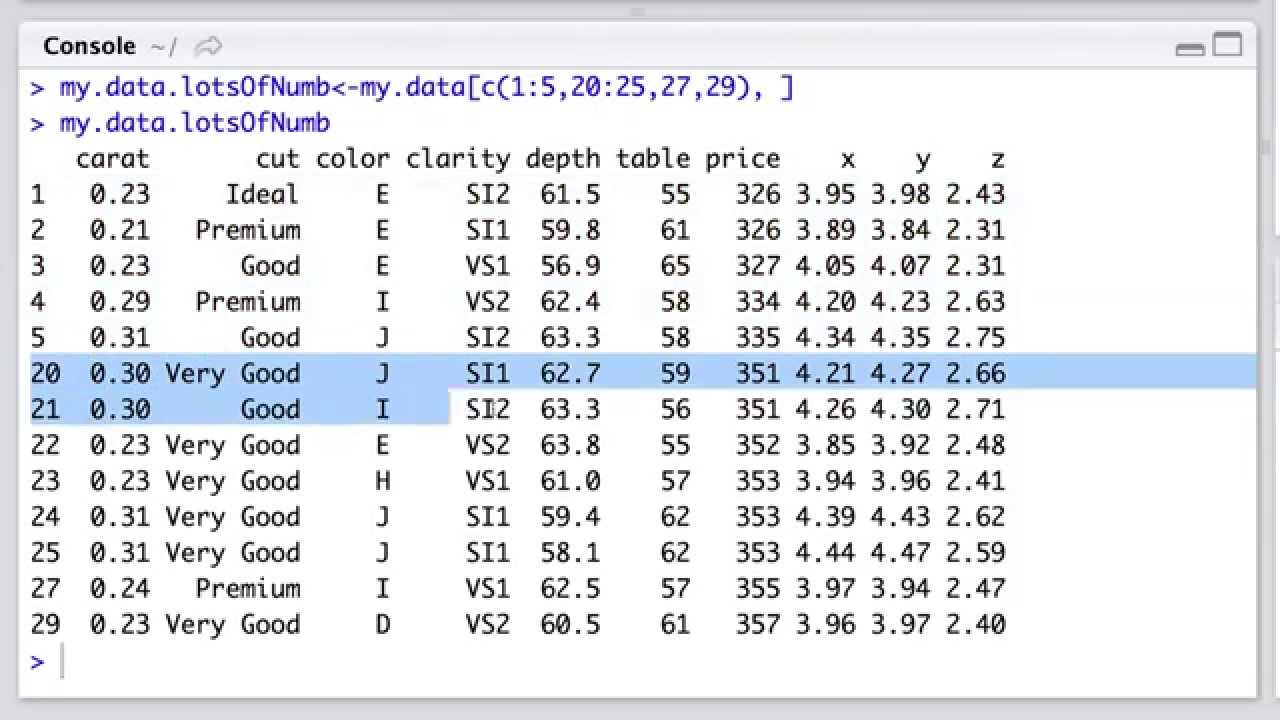
When working with data in R, it is common to encounter situations where you need to remove a row of data from a data frame. Whether it’s due to errors, duplicates, or irrelevant information, removing a row can help you clean and manipulate your data effectively. In this article, we will explore different methods to remove a row of data in R, providing you with the tools you need to streamline your data analysis and make informed decisions. From using indexing and subsetting techniques to leveraging functions like subset() and filter(), we will walk you through step-by-step instructions and examples, enabling you to confidently remove rows from your data frame. So, let’s dive in and learn how to remove a row of data in R!
Inside This Article
- Overview of Removing a Row of Data in R
- Method 1: Using the subset() Function- Syntax of the subset() function in R- Examples of removing a row of data using subset()
- Method 2: Using the filter() Function from the dplyr Package
- Method 3: Using the remove() Function from the data.table Package
- Method 4: Using the – Operator- Explanation of the – operator for row removal in R- Examples of removing a row of data using the – operator
- Conclusion
- FAQs
Overview of Removing a Row of Data in R
When working with data in R, there may be instances where you need to remove a particular row of data from a dataset. Removing a row of data is a common task in data analysis and can be useful when dealing with outliers, duplicate records, or irrelevant information.
R provides several methods to remove a row of data from a dataframe or a matrix. In this article, we will explore different approaches to achieve this goal. Whether you are a beginner or an experienced R programmer, understanding these methods will expand your toolkit and help you efficiently manipulate and clean your data.
Below, we will delve into four popular methods for removing a row of data in R:
- Using the subset() function
- Using the filter() function from the dplyr package
- Using the remove() function from the data.table package
- Using the – operator
Method 1: Using the subset() Function- Syntax of the subset() function in R- Examples of removing a row of data using subset()
When working with data in R, you may come across scenarios where you need to remove a specific row from a dataset. One approach to achieve this is by using the subset() function. This function allows you to filter and subset your data based on specific conditions.
The syntax of the subset() function in R is as follows:
- subset(data, condition)
The “data” parameter represents the dataset from which you want to remove a row, and the “condition” parameter specifies the condition that needs to be met for a row to be removed.
To remove a row using the subset() function, you can define the condition based on the values in a specific column. For example, let’s say you have a dataset named “mydata” with a column called “age”. If you want to remove all rows where the age is less than 18, you can use the following code:
- mydata_subset <- subset(mydata, age >= 18)
This code creates a new dataset, “mydata_subset”, that excludes all rows where the age is less than 18. The original dataset, “mydata”, remains unchanged.
Here’s another example. Let’s say you have a dataset named “students” with columns for “name”, “age”, and “grade”. You want to remove all rows where the grade is below C (grade < "C"). The code to achieve this would be:
- students_subset <- subset(students, grade >= “C”)
This code creates a new dataset, “students_subset”, that excludes all rows where the grade is below C.
Using the subset() function gives you the flexibility to remove rows based on specific conditions, making it a powerful tool in data manipulation and analysis in R.
Method 2: Using the filter() Function from the dplyr Package
One popular method for removing a row of data in R is by using the filter()
function from the dplyr package. This package is a powerful toolkit for data manipulation, providing intuitive and efficient functions for data cleaning and transformation.
To use the filter()
function, you first need to install and load the dplyr package in R. You can do this by running the following commands:
install.packages("dplyr")
library(dplyr)
Once the package is installed and loaded, you can start using the filter()
function to remove a row of data based on specific criteria. The syntax of the filter()
function is as follows:
filtered_data <- filter(data, condition)
Here, data
represents the name of your dataframe or dataset, and condition
specifies the filtering criteria. The condition
can be any logical expression that evaluates to either TRUE
or FALSE
.
Let’s take a look at some examples of removing a row of data using the filter()
function:
Example 1:
# Create a dataframe
data <- data.frame(
name = c("John", "Emily", "Michael", "Sarah"),
age = c(25, 30, 28, 35),
city = c("New York", "Chicago", "Los Angeles", "Boston")
)
# Remove the row where name is "Emily"
filtered_data <- filter(data, name != "Emily")
# Print the filtered data
print(filtered_data)
In this example, we have a dataframe with columns for “name”, “age”, and “city”. We use the filter()
function to remove the row where the name is “Emily”. The resulting filtered data will contain all rows except the one where the name is “Emily”.
Example 2:
# Create a dataframe
data <- data.frame(
name = c("John", "Emily", "Michael", "Sarah"),
age = c(25, 30, 28, 35),
city = c("New York", "Chicago", "Los Angeles", "Boston")
)
# Remove the row where age is greater than or equal to 30
filtered_data <- filter(data, age < 30)
# Print the filtered data
print(filtered_data)
In this example, we remove the row where the age is greater than or equal to 30. The resulting filtered data will only include rows where the age is less than 30.
The filter()
function offers flexibility in specifying the conditions for row removal, making it a handy tool for data manipulation in R.
Method 3: Using the remove() Function from the data.table Package
When it comes to removing a row of data in R, the remove()
function from the data.table
package provides a convenient way to accomplish this task. Before using the remove()
function, you need to make sure that the data.table
package is installed and loaded in your R environment.
To install the data.table
package, you can use the following command:
install.packages("data.table")
Once the package is installed, you can load it into your R session using the library()
function:
library(data.table)
Now that you have the data.table
package ready, let’s dive into the syntax of the remove()
function and explore some examples.
Syntax of the remove() function in data.table
The remove()
function in the data.table
package allows you to remove rows from a data table based on a specified condition. The basic syntax of the remove()
function is as follows:
remove(data.table, condition)
Here, data.table
refers to the name of your data table, and condition
is the condition that determines which rows should be removed.
Examples of removing a row of data using remove()
Let’s consider an example where we have a data table called myDT
with three columns: name
, age
, and gender
. We want to remove the rows where the age is equal to 30.
# Create a data table
myDT <- data.table(name = c("John", "Amy", "Mike", "Lisa"),
age = c(25, 30, 30, 35),
gender = c("Male", "Female", "Male", "Female"))
# Remove rows where age is equal to 30
myDT <- remove(myDT, age == 30)
# Print the updated data table
print(myDT)
By executing the above code, the rows where the age is equal to 30 will be removed from the myDT
data table. The resulting data table will only contain the rows where the age is either 25 or 35.
The remove()
function can handle more complex conditions as well. For example, you can add multiple conditions using logical operators like &
(AND) or |
(OR) to specify which rows should be removed.
Now that you are familiar with the remove()
function from the data.table
package, you can efficiently remove specific rows of data from your data tables in R.
Method 4: Using the - Operator- Explanation of the - operator for row removal in R- Examples of removing a row of data using the - operator
Another method to remove a row of data in R is by using the - operator. This operator allows you to exclude specific rows from a dataframe. It can be a concise and straightforward approach, especially when dealing with smaller datasets.
The - operator works by specifying the row numbers you want to remove after the dataframe name. This is similar to subsetting the dataframe but selecting all rows except the ones you want to remove.
Here's an example to illustrate how to remove a specific row using the - operator:
R
# Create a sample dataframe
df <- data.frame(
name = c("John", "Jane", "Mark", "Sara"),
age = c(25, 30, 35, 40),
city = c("New York", "London", "Paris", "Tokyo")
)
# Remove the second row
df <- df[-2, ]
# Print the updated dataframe
print(df)
In this example, we have a dataframe called "df" with three columns: name, age, and city. By using the - operator along with the row number, we exclude the second row (Jane's row) from the dataframe. The updated dataframe is then printed, showing that the second row has been successfully removed.
By adjusting the row number in the square brackets, you can remove any specific row from your dataframe. If you want to remove multiple rows, you can simply provide a vector of row numbers. For example, to remove the second and fourth rows, you can use:
R
df <- df[-c(2, 4), ]
This will remove both the second and fourth rows from the dataframe.
It's worth noting that using the - operator may not be the most efficient method for removing rows from large datasets. In such cases, the other methods mentioned earlier, like subset(), filter(), or remove(), might be more suitable. However, for smaller datasets or quick row removal tasks, the - operator can be a handy and straightforward option.
Always remember to assign the modified dataframe back to the original variable name to ensure that the changes are applied and saved.
Conclusion
In conclusion, removing a row of data in R is a straightforward process that can be accomplished using various methods. Whether you choose to use the subset() function, the negative index method, or the filter() function from the dplyr package, you have the flexibility to customize your data cleaning process to suit your specific needs.
It is important to carefully consider the impact of removing a row of data and ensure that it aligns with the goals of your data analysis. Additionally, always remember to make a backup of your dataset before making any modifications to avoid accidental data loss.
By mastering the art of removing rows in R, you will be equipped to confidently clean and manipulate your data, empowering you to derive valuable insights and make informed decisions.
FAQs
1. Can I remove a single row of data in R?
Yes, you can remove a single row of data in R. You can specify the row index using the square brackets notation and assign an empty value to that row.
2. How can I remove multiple rows of data in R?
To remove multiple rows of data in R, you can create a new data frame without the rows you want to remove. You can use the `subset()` function with a logical condition to filter out the rows you don't need.
3. Can I remove a row based on a specific condition in R?
Yes, you can remove a row based on a specific condition in R. You can use the `subset()` function with a logical condition to filter out the rows that satisfy the condition you specify.
4. What if I want to remove a row by its row name or row label in R?
If you want to remove a row by its row name or row label in R, you can use the `filter()` function from the `dplyr` package. You can specify the row name or label as the filter condition to exclude that specific row.
5. Can I remove a row without creating a new data frame in R?
Yes, you can remove a row without creating a new data frame in R. You can use the `subset()` function with the negation (`!`) operator to exclude the row you want to remove from the original data frame.