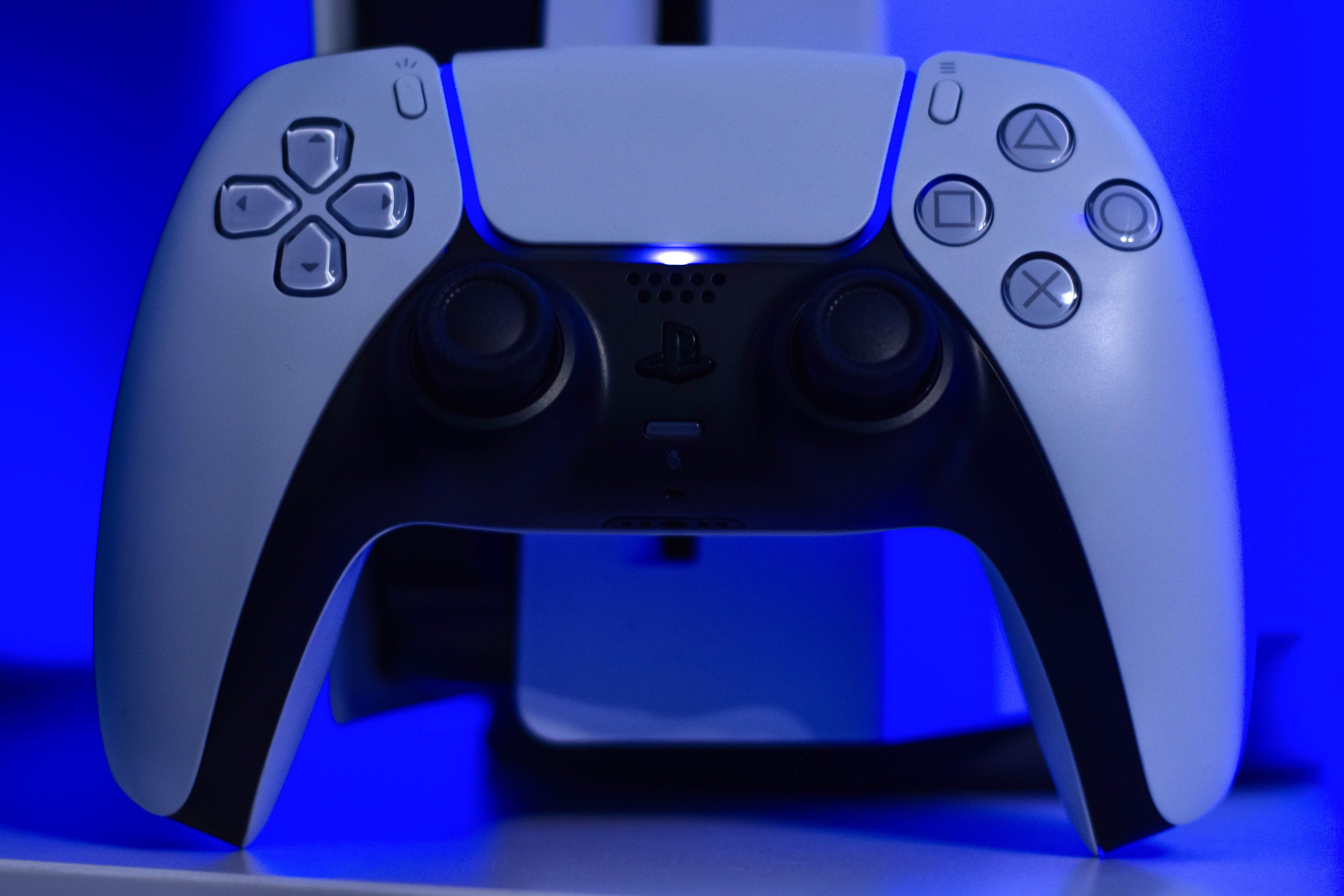
When it comes to game development, creating immersive and interactive experiences is key. One essential aspect of game mechanics is the ability to make objects face the direction the joystick is pointing. This feature adds an extra layer of realism and engagement to the gameplay, allowing players to control their character’s movements with precision.
In this article, we will explore how to implement this functionality using Unity, a powerful game development engine. We will delve into the step-by-step process of making an object face the same direction as the joystick in a user-friendly and efficient manner. Whether you’re a beginner or an experienced game developer, this guide will equip you with the knowledge and skills required to enhance your game with this dynamic feature.
Inside This Article
- Method 1: Using Transform.LookAt
- Method 2: Calculating Angle and Rotating
- Method 3: Applying Quaternion Rotation
- Conclusion
- FAQs
Method 1: Using Transform.LookAt
One of the most straightforward ways to make an object face the way the joystick is facing in Unity is by utilizing the Transform.LookAt()
method. This method allows you to rotate an object to face a specified target point. In this case, the target point will be the direction that the joystick is indicating.
To implement this method, you need to follow a few steps. First, you’ll need to have a reference to the joystick’s direction vector. This vector will represent the direction in which the joystick is pointing. You can obtain this vector by accessing the joystick’s input values from your input system.
Once you have the joystick’s direction vector, you can use it to calculate the target point to face. You can do this by adding the joystick’s direction vector to the object’s current position, resulting in a target point that is in front of the object.
Next, you can use the Transform.LookAt()
method to rotate the object to face the target point. The method takes the target point as its parameter and adjusts the object’s rotation accordingly. This will make the object face the direction indicated by the joystick.
It’s important to note that using Transform.LookAt()
will align the object’s forward vector directly with the direction of the joystick. This means the object will face the same direction as the joystick, regardless of its initial rotation or orientation.
This method is useful when you want an object to always face the direction indicated by the joystick, regardless of other factors or constraints. It provides a simple and effective way to achieve the desired behavior.
Method 2: Calculating Angle and Rotating
In this method, we will calculate the angle between the direction the joystick is pointing and the direction the object is currently facing. Then, we will rotate the object to face the same direction as the joystick.
Here are the steps to implement this method:
- Get the direction the joystick is pointing by accessing the joystick input values. In Unity, this can be obtained using input.GetAxis() or input.GetVector() function.
- Normalize the joystick direction vector to ensure consistent behavior.
- Calculate the angle between the joystick direction and the object’s current forward direction using the Vector3.Angle() function. This will give us the rotation angle needed to face the joystick direction.
- Create a Quaternion rotation using the calculated angle and the object’s up direction. This rotation will align the object with the joystick direction.
- Apply the rotation to the object by assigning the calculated Quaternion to the object’s transform.rotation property.
By using this method, the object will smoothly rotate and face the same direction as the joystick input. This can be helpful in various game scenarios where the player’s control is linked to the object’s orientation.
Method 3: Applying Quaternion Rotation
Another method to make an object face the way the joystick is facing in Unity is by using Quaternion rotation. Quaternion rotation is a mathematical representation of rotation in three-dimensional space.
The first step is to get the direction vector from the joystick input. This can be done by accessing the joystick input values and creating a new Vector3 with those values as its x and y coordinates.
Next, we normalize the direction vector to have a magnitude of 1. This is necessary to ensure consistent and accurate rotation.
Now, we can calculate the rotation using Quaternion.LookRotation() function. This function takes two parameters – the direction vector and the up direction. The up direction is usually Vector3.up, which represents the positive y-axis.
The Quaternion.LookRotation() function returns a Quaternion that represents the rotation needed to face the specified direction. We can then apply this rotation to our object by setting its transform.rotation to the calculated Quaternion.
Here’s an example code snippet:
Vector3 direction = new Vector3(joystick.Horizontal, 0, joystick.Vertical);
direction.Normalize();
Quaternion rotation = Quaternion.LookRotation(direction, Vector3.up);
transform.rotation = rotation;
This code snippet assumes that you have a joystick object named “joystick” and you want to apply the rotation to a transform component named “transform”.
By using Quaternion rotation, you can easily make an object face the way the joystick is facing in Unity. This method provides a smooth and accurate rotation, which is essential for many games and applications.
Conclusion
In conclusion, learning how to make an object face the way the joystick is facing can greatly enhance the gameplay experience in Unity. By implementing the code discussed in this article, you can create dynamic and interactive environments where objects respond to the player’s input.
The use of Unity’s Transform and Input classes enables you to easily access and manipulate the rotation of objects, ensuring they align with the joystick’s direction. Additionally, utilizing normalized vectors and Quaternion.LookRotation provides a smooth and accurate rotation effect.
Whether you are creating a 2D or 3D game, this functionality can add realism and player immersion. Experiment with different scenarios and tweak the code to suit your specific needs and game mechanics. With practice, you will become proficient in making objects face the joystick direction with ease.
So why wait? Apply this knowledge to your Unity projects and take your gameplay to the next level by making objects seamlessly face the way the joystick is facing.
FAQs
Q: How do I make an object face the way the joystick is facing in Unity?
A: To make an object face the way the joystick is facing in Unity, you can use the LookRotation method combined with the joystick input. This method calculates the rotation needed for the object to face a specified direction. By passing the joystick input vector as the direction parameter, you can make the object rotate accordingly.
Q: What is the LookRotation method in Unity?
A: The LookRotation method in Unity is a built-in function that calculates the rotation needed for an object to face a specified direction. It takes a direction vector as a parameter and returns a Quaternion representing the rotation. This method is often used in games to make objects face a certain point or follow a specific path.
Q: How can I access the joystick input in Unity?
A: To access the joystick input in Unity, you can use the Input class. Specifically, you can use the GetAxis method to retrieve the horizontal and vertical axis values of the joystick. These values represent the position of the joystick along the respective axes. By combining these values, you can determine the direction in which the joystick is being moved.
Q: Can I use the joystick input to control other aspects of my game besides object rotation?
A: Absolutely! The joystick input in Unity is versatile and can be used to control various aspects of your game. Apart from object rotation, you can use the joystick input to move characters, control camera angles, initiate actions, and more. The input values retrieved from the joystick can be used creatively to create immersive and interactive gameplay experiences.
Q: Are there any alternative methods to make an object face the joystick direction in Unity?
A: Yes, there are alternative methods to make an object face the joystick direction in Unity. One popular approach is to use the Quaternion.LookRotation method along with the Vector3.RotateTowards method. The LookRotation method calculates the rotation needed to face the joystick direction, while the RotateTowards method smoothly rotates the object towards the desired direction. This combination allows for more precise control over the object’s rotation.