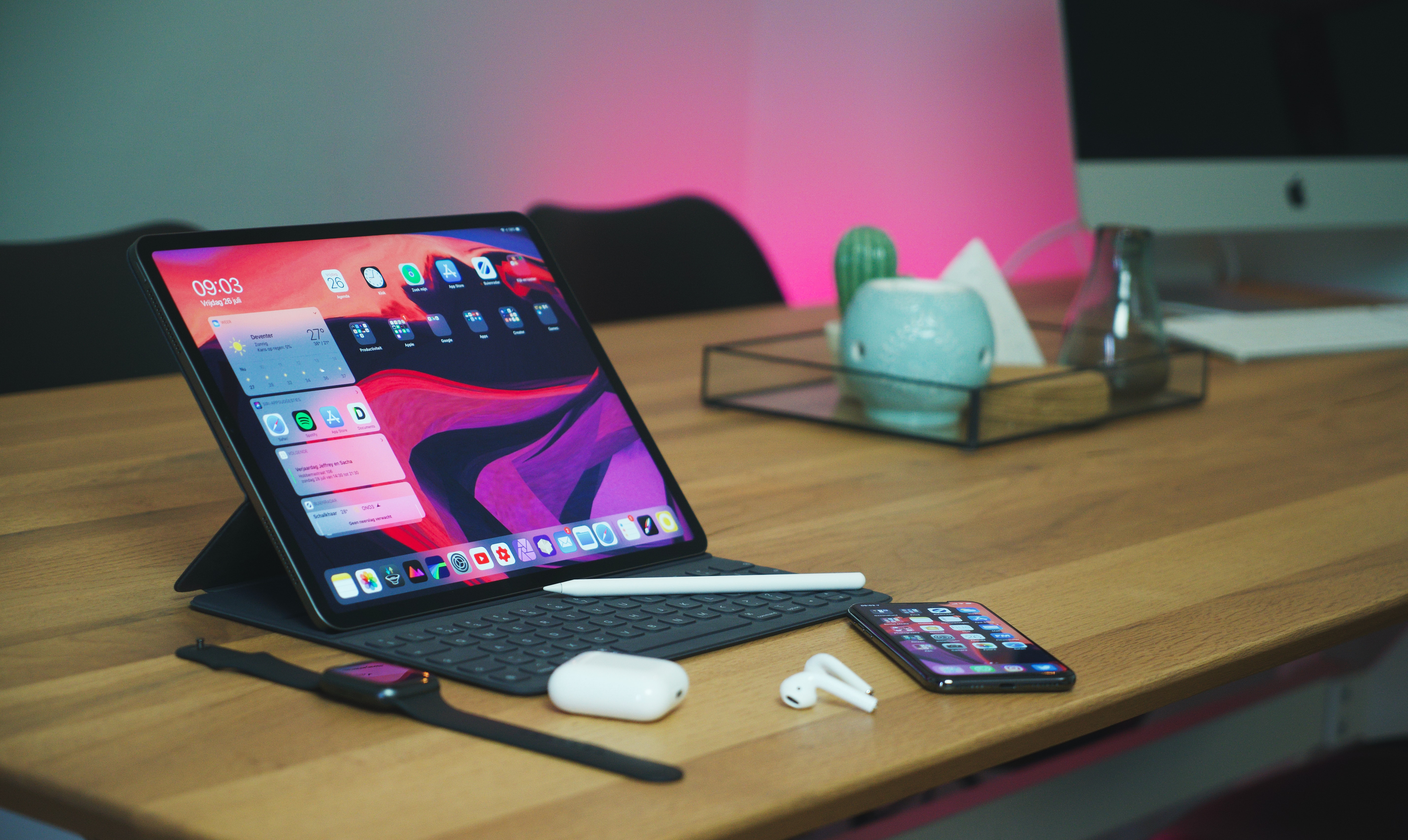
Developing mobile apps has become an essential skill in today’s tech-savvy world, and there’s no better platform to showcase your talent than iOS. With its sleek design, seamless user experience, and a massive user base, iOS has emerged as a dominant force in the mobile app market.
However, coding an iOS app may seem like a daunting task for beginners. Fear not! In this comprehensive guide, we will walk you through the process of coding an iOS app, from setting up the development environment to implementing key features and functionalities.
Whether you are a seasoned developer looking to expand your skillset or a newbie taking your first step into the app development realm, this article will provide you with the necessary knowledge and resources to dive into iOS app coding with confidence.
Inside This Article
- Choosing the Right Development Environment
- Setting Up Xcode and iOS Simulator
- Basic Swift Programming Concepts
- Understanding iOS App Architecture
- Conclusion
- FAQs
Choosing the Right Development Environment
When it comes to developing iOS apps, choosing the right development environment is crucial for a successful and efficient development process. The development environment you choose plays a significant role in determining your productivity, code quality, and overall experience. Here are a few factors to consider when selecting the right development environment for iOS app development:
- IDE (Integrated Development Environment): The IDE is a software application that provides a comprehensive set of tools for app development. One of the most widely used IDEs for iOS app development is Xcode. Xcode offers a wide range of features including code editing, debugging, and interface design. It is specifically designed for developing iOS and macOS apps and provides seamless integration with Apple’s frameworks and APIs.
- Compatibility: Ensure that the development environment you choose is compatible with the version of iOS you are targeting. This ensures that you have access to the latest features and tools provided by Apple and allows you to take full advantage of the platform’s capabilities.
- Community and Support: It is important to choose a development environment that has a strong and active community. This ensures that you have access to a wealth of resources, tutorials, and forums where you can seek help and guidance. Additionally, a strong community indicates that the development environment is widely adopted and trusted by developers.
- Integration with Version Control: Version control is essential for collaborative development and managing code changes. Ensure that the development environment you choose seamlessly integrates with popular version control systems such as Git. This allows you to track changes, collaborate with other developers, and easily roll back to previous versions if needed.
- Performance and Efficiency: Consider the performance and efficiency of the development environment. A laggy and slow development environment can significantly impact your productivity and hamper the development process. Look for an environment that offers fast build times, responsive debugging tools, and efficient code navigation.
Ultimately, the choice of a development environment depends on your personal preference, the complexity of your app, and the specific requirements of your project. While Xcode is the default and most widely used environment for iOS app development, there are alternative options available, such as AppCode and Visual Studio for Mac. Evaluate your options carefully and choose the development environment that best suits your needs and enhances your productivity as an iOS app developer.
Setting Up Xcode and iOS Simulator
Setting up Xcode and iOS Simulator is an essential step in coding iOS apps. Xcode is the primary integrated development environment (IDE) for iOS app development, while iOS Simulator allows you to test your app on virtual iOS devices. Here’s how to get started:
- Download Xcode: Xcode is available for free on the Mac App Store. Simply search for “Xcode” and click on the download button to install it on your Mac.
- Update macOS:Xcode requires the latest version of macOS to run smoothly. Make sure your Mac is running the latest version by going to the Apple menu, selecting “System Preferences,” and clicking on “Software Update.” Follow the instructions to update your macOS if needed.
- Launch Xcode: Once the installation is complete, you can launch Xcode from the Applications folder or by searching for it in Spotlight.
- Agree to Terms and Conditions: When you launch Xcode for the first time, you will need to agree to the terms and conditions to continue.
- Install Additional Components: Xcode may prompt you to install additional components necessary for iOS development. Follow the on-screen instructions to complete the installation of these components.
- Open iOS Simulator: With Xcode open, go to the “Xcode” menu and select “Preferences.” In the Preferences window, click on the “Components” tab and locate “iOS Simulator.” Click on the “Install” button next to it to download and install iOS Simulator.
- Choose the Device: Once iOS Simulator is installed, you can choose the virtual device on which you want to test your app. In Xcode, go to the toolbar and click on the device selection dropdown. From the list of available devices, select the one that matches your target device.
- Run Your App: With the iOS Simulator running and the desired device selected, you can now build and run your app on the virtual device. Use the “Product” menu in Xcode to build and run your app, or simply press Command + R on your keyboard.
Setting up Xcode and iOS Simulator is critical for iOS app development. It provides you with the necessary tools and environment to create and test your apps. By following these steps, you’ll be on your way to building and running your iOS apps in no time.
Basic Swift Programming Concepts
When it comes to developing iOS apps, having a solid understanding of Swift programming is essential. Swift is a powerful and intuitive programming language that has become the language of choice for iOS app development. In this section, we will explore some of the basic concepts of Swift programming to get you started on your journey as an iOS app developer.
Data Types and Variables: In Swift, you can work with different data types such as integers, strings, booleans, and more. You can declare variables using the var
keyword and constants using the let
keyword. For example:
var age: Int = 25
let name: String = "John Smith"
var isStudent: Bool = true
Control Flow: Control flow statements allow you to control the execution flow of your code. Swift provides various control flow statements like if
, switch
, for-in
, and while
. For example:
if age < 18 {
print("You are a minor")
} else {
print("You are an adult")
}
for i in 1...5 {
print(i)
}
while count <= 10 {
print(count)
count += 1
}
Functions: Functions allow you to encapsulate a piece of code that performs a specific task. You can define functions using the func
keyword. For example:
func greet(name: String) {
print("Hello, \(name)!")
}
greet(name: "Sarah") // Output: Hello, Sarah!
Optionals: Optionals are a unique feature in Swift that allows you to represent the absence of a value. You can declare an optional variable by adding a question mark (?
) after the data type. For example:
var age: Int?
age = 25
if let userAge = age {
print("The user's age is \(userAge)")
} else {
print("No age value found")
}
Classes and Objects: Object-oriented programming is an important aspect of Swift. You can define classes, create objects, and access their properties and methods. For example:
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func greet() {
print("Hello, my name is \(name) and I am \(age) years old.")
}
}
let person = Person(name: "John", age: 30)
person.greet() // Output: Hello, my name is John and I am 30 years old.
These are just a few of the basic concepts of Swift programming. By mastering these fundamentals, you will have a solid foundation to build upon as you delve deeper into the world of iOS app development.
Understanding iOS App Architecture
When it comes to developing an iOS app, it is crucial to have a clear understanding of the underlying architecture. iOS app architecture is the foundation upon which your app is built, determining its structure, organization, and behavior. By understanding the different components of iOS app architecture, you can design and develop robust and scalable apps that provide a seamless user experience.
The key components of iOS app architecture include:
- Model: The model represents the data and the logic behind it. It encapsulates the business logic of your app, storing and manipulating the data.
- View: The view is responsible for presenting the app’s user interface to the user. It defines the visual elements and controls that the user interacts with.
- Controller: The controller acts as an intermediary between the model and the view. It receives input from the user and manipulates the model accordingly. It also updates the view to reflect the changes in the model.
In iOS app development, the most commonly used architectural pattern is the Model-View-Controller (MVC) pattern. This pattern separates the different components of an app, allowing for better organization and maintainability.
With the MVC pattern, the model, view, and controller each have their own responsibilities:
- The model is responsible for managing the data and maintaining the app’s state. It handles tasks such as data validation, storage, and retrieval.
- The view is responsible for displaying the user interface elements to the user. It receives updates from the controller and renders the appropriate content.
- The controller acts as the intermediary between the model and the view. It handles user input, updates the model, and updates the view accordingly.
By separating the responsibilities of the model, view, and controller, the MVC pattern enables efficient development, code reusability, and easier maintenance. It allows for better scalability as the app grows in complexity.
However, it is important to note that the MVC pattern is not the only architectural pattern for iOS app development. There are other patterns such as MVVM (Model-View-ViewModel), VIPER (View-Interactor-Presenter-Entity-Router), and Clean Architecture, each with its own advantages and use cases.
Understanding iOS app architecture is essential for building high-quality, maintainable apps. By choosing the right architectural pattern and appropriately structuring your code, you can create apps that are robust, scalable, and easy to maintain.
Conclusion
In conclusion, coding iOS apps can seem like a daunting task, but with the right knowledge and resources, it becomes an achievable feat. Throughout this article, we have explored the essential steps and considerations involved in coding an iOS app. From setting up the development environment to writing code and testing the app, each phase contributes to the overall success of the project.
Remember, the key to coding iOS apps effectively lies in having a strong foundation in Objective-C or Swift programming languages, understanding the iOS SDK, and keeping up with the latest app development best practices. Furthermore, leveraging the power of popular mobile app development frameworks, such as SwiftUI and React Native, can significantly speed up the development process and enhance the user experience.
By following a systematic approach, continuously learning and adapting to the evolving iOS ecosystem, and staying updated with the latest trends and technologies, you can create engaging and innovative iOS apps.
So, take the plunge, embark on your iOS app development journey, and let your creativity and coding skills shape the future of mobile applications. Good luck, and happy coding!
FAQs
1. What programming language do I need to code iOS apps?
To code iOS apps, you primarily need to use the Swift programming language. Swift is a powerful and intuitive language developed by Apple specifically for iOS, macOS, watchOS, and tvOS app development. It offers modern syntax, extensive libraries, and tools that make it easier for developers to build robust and efficient iOS applications.
2. Do I need a Mac to code iOS apps?
Yes, a Mac is required to develop iOS apps. The entire iOS development ecosystem, including Xcode (the Integrated Development Environment for iOS apps) and the iOS Simulator, is only available for macOS. You can either use a physical Mac computer or set up a virtual macOS environment using tools like VMWare or VirtualBox. Without a Mac, it is not possible to compile or test iOS apps.
3. Can I develop iOS apps without prior coding experience?
It is certainly possible to develop iOS apps without prior coding experience, but it may require some learning and practice. There are various resources available to help beginners get started, such as online tutorials, coding bootcamps, and official Apple documentation. It is recommended to start with learning the basics of programming concepts and then gradually move on to iOS app development. Persistence and a willingness to learn are key to becoming proficient in coding iOS apps.
4. What tools do I need to code iOS apps?
The main tool you need to code iOS apps is Xcode. Xcode is a comprehensive development environment that includes the necessary compilers, editors, and debugging tools for iOS app development. It also provides an interface builder for designing app layouts visually. Xcode is available for free on the Mac App Store. Additionally, you may need to use other tools like git for version control, and design software like Sketch or Adobe XD for creating app interfaces.
5. Can I deploy my iOS app on the App Store?
Yes, once you have developed and tested your iOS app, you can submit it to the App Store for distribution. However, there are certain guidelines and requirements set by Apple that you need to adhere to. This includes obtaining a developer account, complying with Apple’s App Store Review Guidelines, and paying an annual fee. Apple reviews all submissions to ensure quality and adherence to their guidelines before approving apps for the App Store.