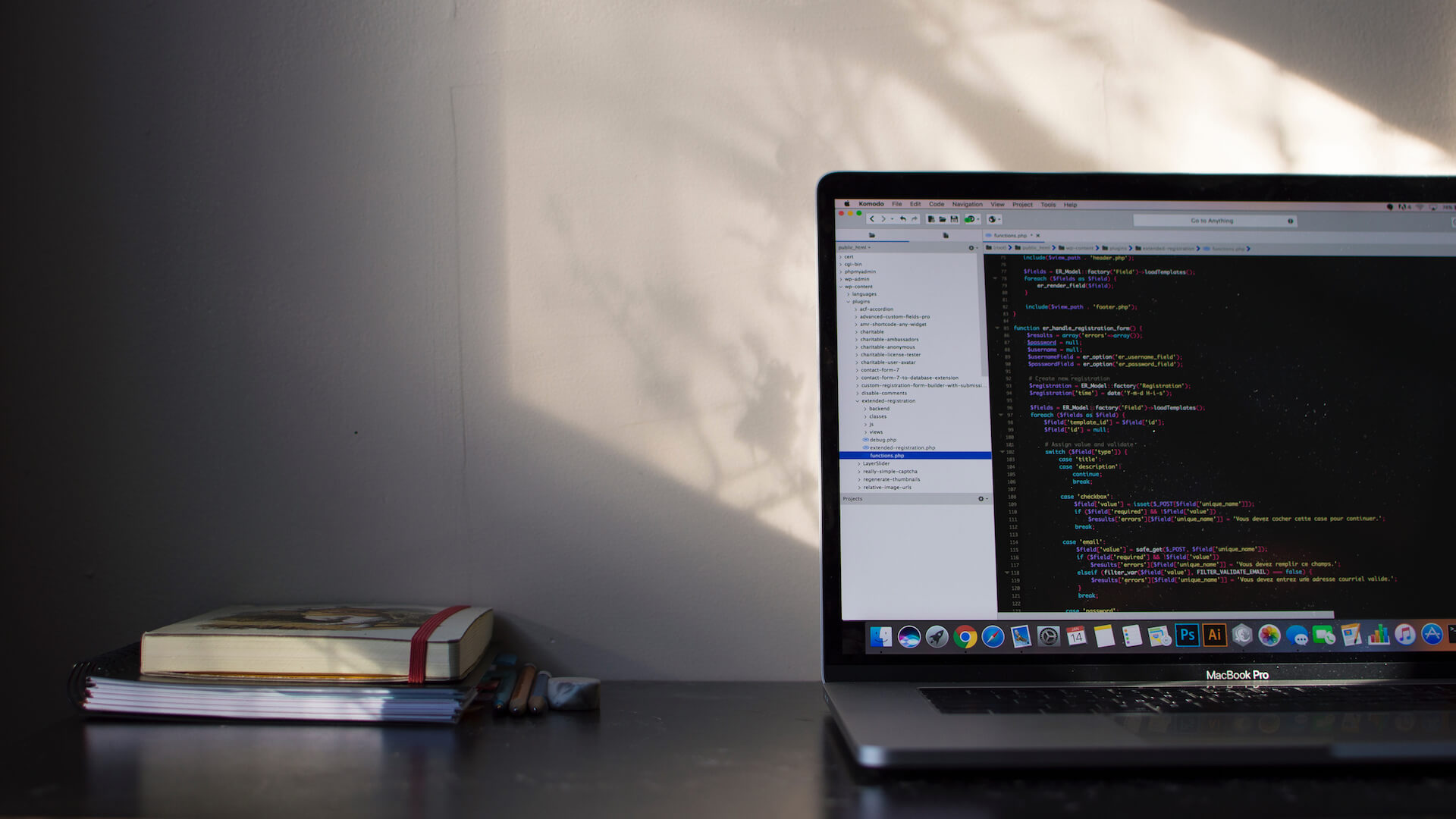
Welcome to Part 2 of our series on Swift Programming 101! In this installment, we will delve into the fascinating world of protocols and delegates. Understanding these concepts is crucial for mastering Swift and developing powerful mobile applications. Protocols allow us to define a set of methods and properties that classes or structs can adopt, while delegates provide a way for one object to communicate with another object. By mastering protocols and delegates, you will gain the ability to create flexible and modular code, enabling better code organization, code reuse, and communication between different parts of your app. In this article, we will explore the fundamentals of protocols and delegates, how to implement them in Swift, and how they can greatly enhance the functionality and extensibility of your iOS or macOS apps.
Inside This Article
- Protocols and Delegates Recap
- Implementing Protocols
- Adopting Multiple Protocols
- Understanding Delegates and Delegation
- Conclusion
- FAQs
Protocols and Delegates Recap
In the world of Swift programming, protocols and delegates play a crucial role in establishing communication between different components of an application. They provide a way to define a set of methods that should be implemented by a class or a structure. This allows for code reusability, modularity, and customization.
A protocol acts as a blueprint for a group of related properties and methods. It defines a set of requirements that a class, structure, or enumeration must conform to in order to be considered as adopting that protocol. By adopting a protocol, a type can inherit its properties and methods, enabling it to fulfill specific functionality.
Delegates, on the other hand, are a design pattern that utilizes protocols to handle communication between objects. A delegate is an object that is assigned to handle specific tasks or provide certain functionality on behalf of another object. By assigning a delegate, an object can delegate the responsibility of implementing certain methods to another object that conforms to the corresponding protocol.
One of the key advantages of using protocols and delegates is to achieve separation of concerns. This means that each component of the application can focus on its specific functionality without worrying about the implementation details of other components. The protocols define a common set of methods that need to be implemented in a standardized manner, allowing for easy integration and collaboration between different parts of the application.
Additionally, protocols and delegates promote code flexibility and extensibility. By adhering to protocols, different types can share the same behavior while implementing their own specific functionality. This allows for modular and reusable code, making it easier to maintain and update the application as requirements change.
Overall, protocols and delegates are powerful concepts in Swift programming that enable modularity, code reusability, and effective communication between different components of an application. By understanding the key concepts and advantages of protocols and delegates, you can harness their potential to write clean, organized, and efficient code.
Implementing Protocols
Implementing protocols is a crucial aspect of Swift programming. It allows you to define a set of methods, properties, and requirements that a class or structure must conform to. By adhering to protocols, you can ensure that your code is modular, flexible, and reusable.
To implement a protocol, you need to declare conformance in the class or structure definition. This is done by adding a colon after the class or structure name, followed by the name of the protocol. For example:
class MyClass: MyProtocol {
Once a class or structure adopts a protocol, it must provide implementations for all the methods and properties defined in the protocol. This is accomplished by using the func
keyword for methods or the var
keyword for properties, followed by the method or property signature.
For example, let’s say you have a protocol named MyProtocol
with a method called myMethod()
. In your class that conforms to this protocol, you would provide an implementation for myMethod()
like this:
func myMethod() {
// Implementation goes here
}
It’s important to ensure that the implemented methods and properties match the requirements defined in the protocol. This includes parameter types, return types, and any other specifications outlined in the protocol’s declaration.
Implementing protocols also allows for protocol inheritance. This means that you can have a protocol inherit from one or more other protocols, forcing the adopting class or structure to conform to both the base protocol and any additional protocols.
By implementing protocols in your Swift code, you can achieve greater modularity, increase code reuse, and improve the overall structure and organization of your application.
Adopting Multiple Protocols
When it comes to Swift programming, protocols offer a powerful way to define a blueprint of properties and methods that a class, structure, or enumeration should implement. They allow for code reuse and ensure a consistent behavior across different types. One of the great advantages of protocols is that a single type can adopt multiple protocols, providing even more flexibility and modularity to your code.
To adopt multiple protocols, simply separate them with a comma when declaring your type’s conformance. For example, let’s say you have a class called “Person” and you want it to conform to both the “Singer” and “Dancer” protocols. You can do so by declaring:
class Person: Singer, Dancer {
// Class implementation goes here
}
By adopting multiple protocols, the “Person” class gains access to all the properties and methods defined within each protocol. This means that an instance of the “Person” class can both sing and dance, as it implements the requirements of both the “Singer” and “Dancer” protocols.
Adopting multiple protocols can be especially useful when you want to build complex, interconnected systems with different functionalities. By breaking down the behavior into smaller, modular protocols, you can mix and match them as needed to create more specialized types.
It’s worth mentioning that while Swift allows for adopting multiple protocols, it’s a good practice to keep the conformances manageable and focused. Adding too many protocols to a type can make the code harder to read and maintain. Therefore, it’s important to consider the purpose and responsibilities of your type when deciding which protocols to adopt.
Understanding Delegates and Delegation
In the world of Swift programming, delegates and delegation play a crucial role in facilitating communication between different objects.
Delegates can be thought of as specialized objects that are responsible for handling certain tasks or events on behalf of another object. These tasks or events can vary from user interactions to data processing and more.
The concept of delegation revolves around the idea of one object delegating its responsibilities to another object. This allows for a clear separation of concerns, making the code more modular and maintainable.
Delegates are often defined using protocols, which specify a set of methods or functions that the delegate object must implement. By conforming to the protocol, the delegate object guarantees that it will be able to handle the required tasks or events.
When an object wants to delegate a task or event to a delegate, it simply calls a specific method or function defined in the protocol. The delegate object, which has adopted the protocol, then receives the message and executes the appropriate code to handle the task or event.
Delegation allows for a powerful form of customization and extensibility in Swift programming. By implementing different delegate objects, you can tailor the behavior of your objects based on the specific requirements of your application or user.
One common example of delegation is in UITableView, where the UITableViewDataSource and UITableViewDelegate protocols are used. The UITableView delegates certain tasks, such as providing data and handling selection events, to another object that conforms to these protocols.
To implement delegation in your code, you need to define a protocol that specifies the required methods or functions. Then, you need to create a delegate property in the delegating object and assign a delegate object to it. Finally, you can call the delegate methods or functions when appropriate.
It’s important to note that delegation is not limited to one-to-one relationships. In some cases, a single object can have multiple delegates, allowing for even more flexibility and customization.
Understanding delegates and delegation is essential for mastering Swift programming, as it enables you to create modular, reusable, and flexible code. By harnessing the power of delegates, you can enhance the functionality and responsiveness of your applications while keeping your codebase organized and maintainable.
Conclusion
In this article, we have delved into the world of protocols and delegates in Swift programming. We have explored their roles in establishing communication and defining behaviors between objects. By mastering protocols and delegates, you can unlock the power of modularity and extensibility in your iOS app development.
Protocols provide a blueprint for defining a set of methods and properties that a class, struct, or enum must adhere to. They enable you to create flexible and reusable code, promoting code encapsulation and separation of concerns.
Delegates, on the other hand, facilitate the communication between objects, allowing them to pass data and trigger actions. By implementing delegate methods, you can customize the behavior of your app and enhance user interactions.
By understanding the concepts and techniques presented in this article, you are now equipped to leverage the full potential of protocols and delegates in your Swift projects. So go ahead, experiment, and take your iOS app development to the next level!
FAQs
1. What is a protocol in Swift?
A protocol in Swift is a blueprint of methods, properties, and other requirements that a class or struct can conform to. It defines a set of rules for what functionality a type must provide, without specifying how that functionality is implemented. Protocols enable objects of different types to interact with each other through a common interface.
2. How do you declare a protocol in Swift?
To declare a protocol in Swift, you use the protocol
keyword followed by the name of the protocol. Inside the protocol, you define the required methods, properties, and other requirements that the conforming types must adhere to. Here’s an example:
protocol MyProtocol {
// Define requirements here
}
3. What is a delegate in Swift?
A delegate in Swift is a design pattern that allows one object to communicate with another object by defining a protocol. The delegate protocol declares methods that the delegating object can call to notify the delegate about certain events or to request information. Delegates are particularly useful in scenarios where an object needs to perform a task but doesn’t have enough information to complete it on its own.
4. How do you implement a delegate in Swift?
To implement a delegate in Swift, you first define a protocol that contains the required methods. Then, you declare a delegate property in the delegating class, typically with the weak
keyword to prevent retain cycles. The delegate object must conform to the protocol, and you can call the delegate methods to notify or request information from the delegate. Here’s an example:
protocol MyDelegate: class {
func didSomething()
}
class MyClass {
weak var delegate: MyDelegate?
func performAction() {
// Do something
delegate?.didSomething()
}
}
5. How can protocols and delegates be used together in Swift?
Protocols and delegates are often used together in Swift to enable communication between objects and to achieve loose coupling. By defining a protocol, you can create a common interface that multiple objects can conform to. Then, by implementing a delegate pattern, you can allow one object to delegate certain responsibilities or actions to another object that conforms to the protocol. This promotes modularity, reusability, and separation of concerns in your code.