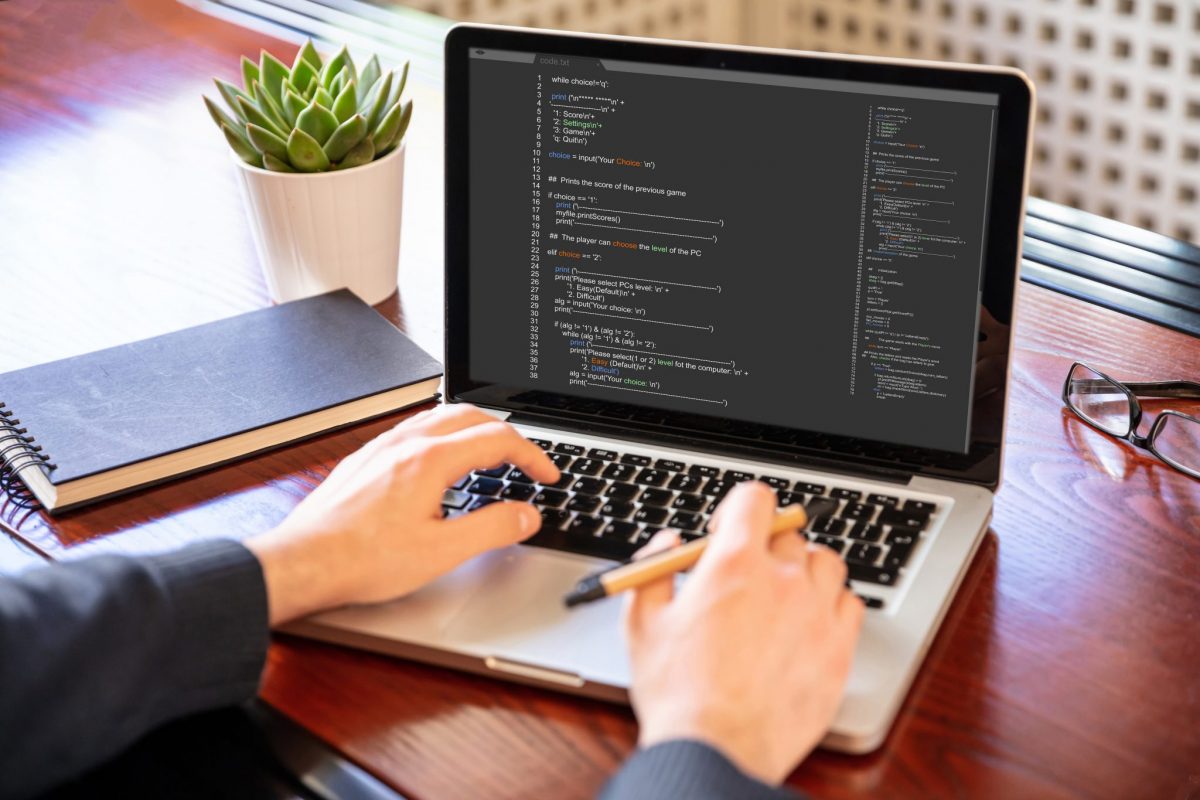
Are you ready to dive into the world of iOS app development? If so, one of the essential aspects you need to understand is the use of Swift programming and its various features. In this comprehensive guide, we will explore one such feature – Generics. Generics allow developers to write flexible and reusable code, providing a practical way to create functions and structures that can work with any type. Whether you are a beginner looking to expand your programming skills, or an experienced developer seeking to brush up on the essentials, this article will provide you with a solid foundation in using Generics within Swift programming. So, let’s get started and unlock the power of Generics in your iOS app development journey!
Inside This Article
- The Basics of Generics in Swift
- Understanding Generic Functions and Methods
- Working with Generic Types and Associated Types
- Applying Constraints to Generics in Swift
- Conclusion
- FAQs
The Basics of Generics in Swift
Generics are a powerful feature in Swift that allow you to write flexible and reusable code. They enable you to define functions, methods, and types that can work with different types of data without sacrificing type safety. In this section, we will explore the basics of generics in Swift and how they can enhance the efficiency and readability of your code.
In simple terms, generics allow you to write code that can be used with different types, rather than being restricted to just one specific type. By using placeholders known as “type parameters,” you can create generic code that can be used with various data types without duplicating code or sacrificing safety.
Let’s consider a practical example. Say you need to write a function to find the maximum value in an array. Without generics, you would have to write separate functions for different types such as integers, floats, and strings.
Fortunately, with generics, you can define a single function that can work with any type that conforms to the Comparable protocol. You can declare the function as follows:
func findMax(array: [T]) -> T {
var max = array[0]
for element in array {
if element > max {
max = element
}
}
return max
}
In the above code, the type parameter T is used to represent the type of elements in the array. The function states that the type T must conform to the Comparable protocol, which ensures that the elements can be compared using the > operator.
With this generic function, you can find the maximum value of any array that contains elements conforming to the Comparable protocol. For example:
let numbers = [5, 2, 8, 1, 9]
let maxNumber = findMax(array: numbers)
print(maxNumber) // Output: 9
let words = ["apple", "banana", "cherry"]
let maxWord = findMax(array: words)
print(maxWord) // Output: cherry
As you can see, by using generics, we were able to write a single function that works with both integer and string arrays, without sacrificing type safety. This is the power of generics in Swift.
Another advantage of generics is code reusability. By abstracting the implementation details of the function or type using generics, you can write code that can be used with different types, reducing code duplication and improving maintainability.
Understanding the basics of generics in Swift is essential for writing efficient and reusable code. By leveraging the power of generics, you can write flexible code that can work with various data types, enhancing both the efficiency and readability of your code.
Understanding Generic Functions and Methods
When it comes to programming in Swift, understanding generic functions and methods is essential. Generics provide a powerful way to write flexible and reusable code that can work with different types. In this section, we will delve into the concept of generic functions and methods and explore how they can benefit your Swift programming.
A generic function is a function that can operate on a variety of types. Instead of specifying a specific type for the function’s parameters or return value, you can use a placeholder type called a “type parameter”. These type parameters act as variables for types and allow you to write code that can work with different types without sacrificing type safety.
Let’s take a look at an example. Suppose you have a function called swap
that swaps the values of two variables. Traditionally, you would need to write a separate function for each type you want to swap. However, with generics, you can write a single function that works with any type.
func swap<T>(a: inout T, b: inout T) {
let temp = a
a = b
b = temp
}
In the example above, the T
in func swap<T>
is the type parameter. It represents any type that you pass in when calling the function. The inout
keyword is used to indicate that the parameters are passed by reference and can be modified inside the function.
With this generic function, you can swap the values of variables of any type. For instance, you can call swap(&a, &b)
where a
and b
are integers, or even call swap(&x, &y)
where x
and y
are strings. The same function can handle different types seamlessly.
In addition to generic functions, Swift also supports generic methods. A generic method is a method that is part of a generic type. Similar to generic functions, generic methods can operate on various types.
To define a generic method, you need to add a type parameter to the method’s declaration, just like you would with a generic function. The type parameter can then be used within the method’s body to perform operations on different types.
Here’s an example where we have a generic class called Stack<T>
that represents a stack data structure:
class Stack<T> {
var elements: [T] = []
func push(_ element: T) {
elements.append(element)
}
func pop() -> T? {
return elements.popLast()
}
}
In the code above, the T
in class Stack<T>
is the type parameter. It allows us to create a stack that can hold elements of any type. The push
and pop
methods can then be used to add and remove elements from the stack, regardless of the specific type.
Generic functions and methods in Swift bring flexibility and reusability to your code. They allow you to write concise and generic code that can handle different types, making your programs more robust and adaptable. By understanding and utilizing generics effectively, you can take your Swift programming skills to the next level.
Working with Generic Types and Associated Types
When it comes to working with generic types and associated types in Swift, it’s essential to understand how they function and how they can be applied in your code. Generic types allow you to define a structure, class, or function that can work with any type, providing flexibility and reusability.
One of the primary benefits of using generic types is the ability to write code that is not tied to a specific data type. This makes your code more flexible and reusable across different data types, saving you time and effort in writing duplicate code for each type.
In Swift, you can create your own generic types by using angle brackets ‘<>‘ to define placeholder types, which can then be replaced with any specific type when you use the generic type. For example, you could create a generic LinkedList type that can hold values of any type by defining it as follows:
class LinkedList<T> {
var head: Node<T>?
// Rest of the implementation goes here
}
Here, the ‘
In addition to generic types, Swift also supports associated types. Associated types allow you to define a placeholder type within a protocol that is associated with a specific implementation. They provide a way to define a set of requirements that must be satisfied by any type implementing the protocol. This allows for more flexible and customizable implementations of protocols.
For example, imagine you have a protocol called Container that represents a generic container type. You could define an associated type called ItemType to represent the type of items that the container can hold:
protocol Container {
associatedtype ItemType
func insert(item: ItemType)
func remove() -> ItemType?
}
By using an associated type, you can now define different types of containers that hold different types of items. For example, you could create a Container implementation for an array, where the associated type ItemType represents the type of elements in the array. Similarly, you could create a Container implementation for a linked list, where the ItemType represents the type of nodes in the list.
Working with generic types and associated types in Swift allows you to write flexible, reusable, and customizable code. By leveraging the power of generics and associated types, you can create code that is not tied to specific types, making your code more versatile and adaptable to different scenarios. So, embrace the power of generics and associated types in Swift, and unlock new possibilities in your programming journey.
Applying Constraints to Generics in Swift
In Swift, generics allow us to write flexible and reusable code. We can create generic functions, methods, and types that can work with a variety of data types. However, sometimes we want to apply constraints to our generics to ensure they only accept specific types that meet certain requirements. This is where applying constraints to generics in Swift becomes essential.
By applying constraints to generics, we can enforce that the generic parameter must adhere to specific protocols, conform to certain classes, or have specific properties or capabilities. This allows us to write more robust code by ensuring that the generic type meets our expectations. Let’s explore some ways to apply constraints to generics in Swift.
1. Using Type Constraints
Swift allows us to use type constraints to restrict the types that can be used with a generic function or type. We can use the where
keyword followed by a type constraint to specify the requirements that the generic type must fulfill.
For example, if we have a generic function that needs to work with types that conform to a specific protocol:
func process(value: T) {
// Code implementation here
}
Here, T
must be a type that conforms to the SomeProtocol
protocol. If we try to pass a type that doesn’t conform to the protocol, the compiler will throw an error.
2. Specifying Class Constraints
In addition to protocols, we can also apply constraints to generics to ensure that the type parameter is a subclass of a specific class. This is useful when we want to restrict the generic type to a specific class hierarchy.
We can use the class
keyword to specify that the generic type must be a subclass of a particular class:
func process(value: T) {
// Code implementation here
}
In this case, the T
type must be a subclass of the SomeClass
class. Any attempt to use a type that is not a subclass of SomeClass
will result in a compilation error.
3. Using Multiple Constraints
Swift also allows us to apply multiple constraints to a generic type parameter using the where
keyword. This allows us to specify a combination of type constraints and class constraints.
For example:
func process(value: T) {
// Code implementation here
}
In this case, the T
type must both conform to the SomeProtocol
protocol and be a subclass of the SomeClass
class. The type parameter must meet both requirements to be used with this function.
4. Using Generic Type Constraints
Swift also allows us to apply constraints to the generic type itself, not just the generic parameter. This is useful when we want to specify that the generic type must have specific properties or capabilities.
We can use the Self
keyword to refer to the generic type itself and apply constraints to it. For example:
protocol SomeProtocol {
init()
func someFunction()
}
func process(value: T.Type) {
let instance = T()
instance.someFunction()
}
In this case, the T
type must conform to the SomeProtocol
protocol and must have an initializer. We can then create an instance of T
and call its someFunction()
method.
By applying constraints to generics in Swift, we can ensure that our code is more reliable and robust. We can restrict the generic types to specific protocols, classes, or requirements, allowing us to write code that is more precise and less prone to errors.
So, the next time you find yourself working with generics in Swift, remember to consider applying constraints to make your code even more powerful and efficient.
Conclusion
In conclusion, Swift programming provides developers with a powerful tool for creating efficient and flexible code. Understanding generics is essential for maximizing the benefits of Swift’s type system and simplifying code implementation. By utilizing generics, developers can create reusable and type-safe code, leading to increased productivity and maintainability.
Throughout this guide, we have covered the fundamental concepts of generics in Swift, exploring their syntax, benefits, and practical use cases. From creating generic functions to defining generic types and protocols, you now have a solid foundation to apply generics in your own projects.
Remember, generics can greatly enhance the flexibility and reusability of your code, allowing you to write efficient and scalable iOS applications. So, don’t hesitate to leverage the power of generics and unlock their potential in your Swift programming journey.
FAQs
1. What are generics in Swift programming?
Generics in Swift programming allow you to write reusable and flexible code that can work with different types. It enables you to define functions, structures, and classes in a way that can operate on various data types without sacrificing type safety or performance.
2. Why should I use generics in my Swift code?
Using generics in your Swift code promotes code reusability and encapsulation. It allows you to write generic algorithms and data structures that can be used with multiple data types. This reduces code duplication and makes it easier to maintain and expand your codebase.
3. How do generics improve performance in Swift?
Generics in Swift provide type safety at compile time, which eliminates the need for runtime type casting and improves performance. By using generics, the compiler can generate specialized code for each specific type, resulting in more efficient execution and reduced memory overhead.
4. Can I create my own generic types in Swift?
Absolutely! In Swift, you can define your own generic types, such as generic structures, classes, and enumerations. This allows you to create flexible and reusable components that can adapt to different types as needed.
5. Are there any limitations to using generics in Swift?
While generics in Swift are powerful and versatile, there are a few limitations to keep in mind. For example, Swift doesn’t support the use of generic protocols directly. However, you can work around this limitation by using associated types or type erasure techniques.