Usually, Android developers use Java to create their apps. However, this doesn’t make Java the best choice by default. If you’re new to making Android apps and don’t know much about programming, we’ve found you an alternative to Java. It’s called Android Kotlin, and it’s a lot easier to work with.
What Is Android Kotlin?
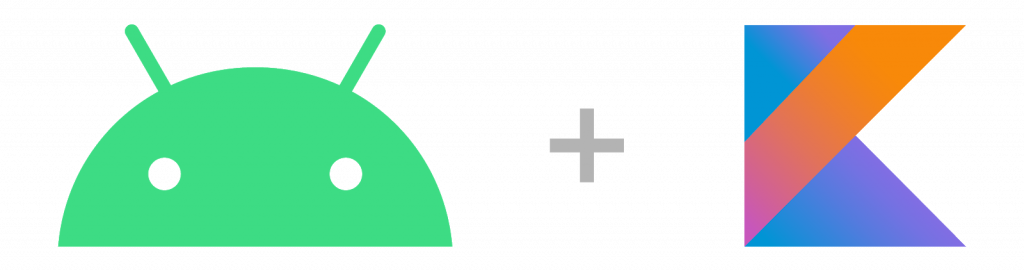
Android Kotlin is one of many Android programming languages that people use, allowing you to create your own apps using code. Kotlin is a versatile and open-source programming language, usually used as one of the Android developer tools. It was designed specifically for Android, and the best part is that it’s free.
JetBrains is the company behind Kotlin. Since its release in 2012, it has remained open-source. This has led to the creation of the Kotlin project on GitHub, which currently has over 300 contributions. The team behind Android Kotlin now has approximately 100 full-time members from its mother company.
Android Kotlin vs Java
People see Android Kotlin as a simpler version of Java. This is mostly true, as most Java users will probably be able to translate their Java knowledge to Kotlin fairly easily. However, there are some differences between Kotlin and Java.
Unlike Java, Android Kotlin focuses on backward compatibility, since it still supports codes from older Java versions that some phones rely on. Additionally, it doesn’t have its own build system and instead chooses to support existing build systems such as Gradle and Maven.
Features of Android Kotlin
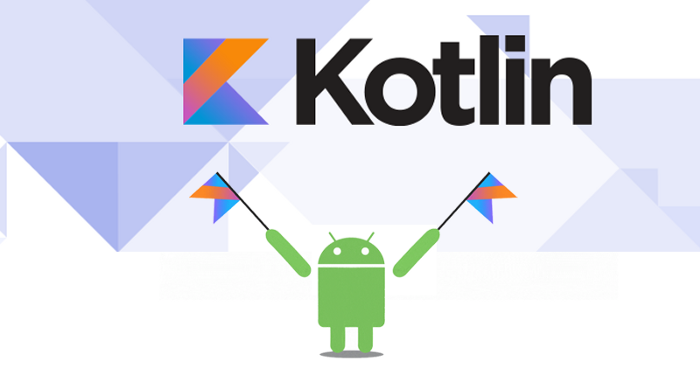
Kotlin for Android developers is quite popular. In fact, over 60% of professional developers use Kotlin to make their apps. Why do people love Kotlin so much? In this section, we talk about some of Kotlin’s best features and why you should use it.
Easy to Learn and Use
First things first, Android Kotlin is extremely easy to learn, and once you understand how it works, it is really easy to use. As we’ve mentioned earlier, it’s like a simpler and more streamlined version of Java. Java is already friendly to beginners, but Kotlin takes a step further. It will make you write less code than many other languages, which in turn reduces file sizes.
Using Kotlin will reduce all of the redundant code that you use in other programming languages. It’s more concise and even gives you beginner tips from time to time. There are also templates for apps, making it infinitely simpler to create apps.
Safe Code
In Java, there are what we call NullPointerExceptions. This is a direct result of nullable variables not being handled properly, which leads to crashes. But when you use Android Kotlin, you reduce the risk of crashing, because NullPointerExceptions are avoided. Apps that utilize Android Kotlin are about 20% less likely to crash than apps that use Java or other programming languages.
Interoperable
If you’re too used to Java, don’t worry. As Kotlin runs much like Java, it has interoperability with it. This is because Kotlin compiles down to the JVM bytecode, the same bytecode that Java runs on.
Kotlin codes can call directly to Java codes, so if you wrote a code in Java and want to transfer it onto Kotlin, there will be little to no problem. You can even use existing Java libraries with Android Kotlin. Additionally, a lot of Android APIs are written using Java, which means you have the ability to call them using Kotlin.
As an example, if you’ve built a huge project with Java that has thousands of lines of code, you can easily convert it to Kotlin. What most app developers do is create their new apps on Kotlin and maintain their old projects using Java.
Multi-Platform Development
You have the ability to use Android Kotlin for something else other than app development. Since it’s interoperable with Java, you can use it for other purposes. Projects outside of app development are easily done using Kotlin.
Moreover, JetBrains has made it so that you have support for Gradle in Android Kotlin. This means that you can write Gradle files in Kotlin. Additionally, Kotlin has been developing support for iOS applications. These factors make Kotlin not just ideal for making apps on Android, but for other purposes as well.
Getting Started With Android Kotlin: What Do You Need?
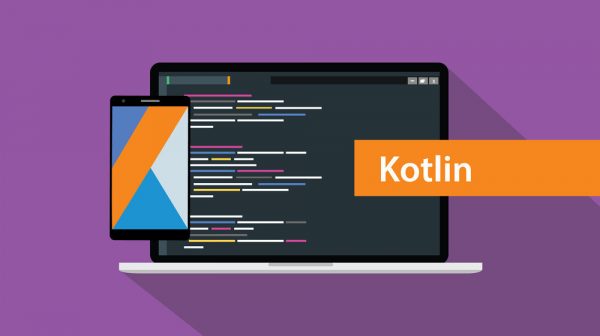
While Kotlin itself is easy enough to learn, there are some prerequisites you must first fulfill before you start. In this section, we explain some of the things that you require before you can start using Android Kotlin.
Computer Literacy
Computer literacy is crucial, as Kotlin entails learning layouts and being familiar with editors. Not being technologically literate will make things a lot harder for you.
How good with computers should you be to understand Kotlin? You should at least have a basic background in programming. You should also know how to navigate through apps.
Good Internet Connection
Kotlin won’t take up too much bandwidth, so your internet connection doesn’t need to be super fast. Nevertheless, you should at least have a stable internet connection.
Android Studio
Android Studio is a studio app that gives you an advanced code editor and IDE. It also provides you with app templates that are excellent for beginners. You’ll have additional access to functions for development, debugging, and testing to make it easier to develop apps.
After you’ve created your apps, Android Studio allows you to test them. You can either do this by downloading emulators or downloading your created app on your Android phone. It’s the premier application for creating and publishing apps to the Google Play Store.
Android Studio is available on Windows, Linux, and Mac—there are no OS restrictions to help you get started with it! It is also frequently updated by its developers, so make sure to keep the program up to date.
Java Development Kit
The Java Development Kit (JDK) is a requirement for anyone who’s using Kotlin to create programs. You’ll need it to run and test any program that you create through Android Kotlin. JDK includes the Java Runtime Environment (JRE), which is another essential component for running Kotlin programs. So you won’t need to install JRE if you already have JDK.
Unlike Android Studio, JDK doesn’t have any inherent functions. Think of it as a launcher to a game, except it’s only launching Android Kotlin. After downloading JDK, you’ll get an installer in your Program Files. Just follow what the installer says and download JDK.
After installing JDK, up next is an installation path. This is where all of your variable files will go for the executable programs of apps you’ll be creating. The proper installation of JDK will then need to be verified. This is because some older versions of JDK are incompatible with Kotlin.
Android Virtual Device Emulator
Android Virtual Device Emulator (AVDE) is something that mimics how an Android device works. If you’ve ever heard of DS emulators or PS4 emulators, AVDE works much the same way. It functions similarly to your average Android phone.
AVDE also speeds up the development process. With it, you won’t have to pair Android Studio with your phone and run your app on it all the time. You can simply run the apps that you create on AVDE, and the results will still be strikingly similar to how they will appear on your Android phone.
Using Android Kotlin: The Basics
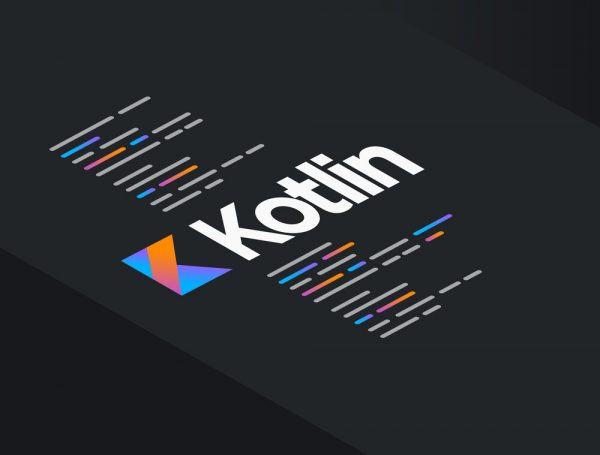
You now have all of the things you need to get started with Android Kotlin. Let’s now move on to the basics of using Android Kotlin for development.
Android Studio
Android Studio houses your project and its corresponding files. There are three main components to the program: the Project, Editing, and Suggestions windows.
The Project window will show all of the things that you currently have in your project. This includes all of the files you’re using and all of the folders that house these files. It’s essentially the master list that helps you keep track of what you’re doing.
The Editor/Editing window is where all the magic happens. This is where you place all of your lines of code and shows which file(s) you’re editing. It will also keep track of errors that you’ve committed.
The Suggestions window tells you all the news about Android Studio. It keeps you notified of what’s new and also gives you some tips based on the code you’re writing.
Functions
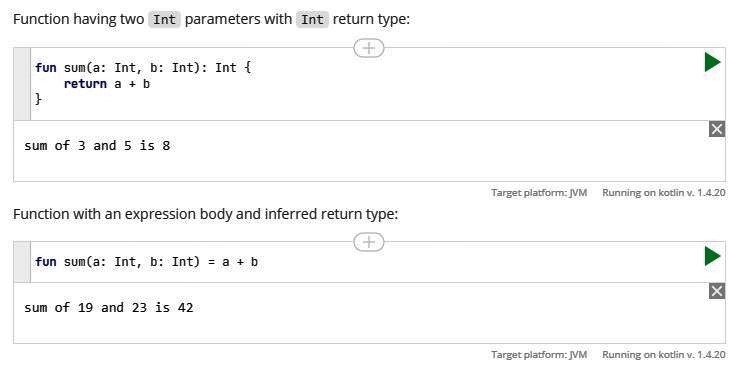
Functions are some of the most basic things that people learn. The term “fun” denotes a function. They represent a value of a functional type, such as a lambda, an anonymous function, or a function reference. There are many types of functions, such as “sum,” or “main.”
Variables
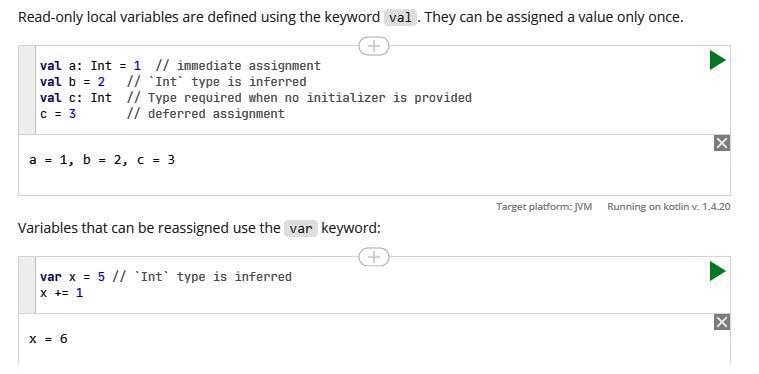
There are two main types of variables: variables that can be defined and variables whose values can be reassigned. Defined variables are called “val,” and can only be assigned a value once. Once you assign a variable to val, you can no longer change its value. They’re like a constant in a formula. Conversely, you can reassign “var” variables.
Conditional Expressions
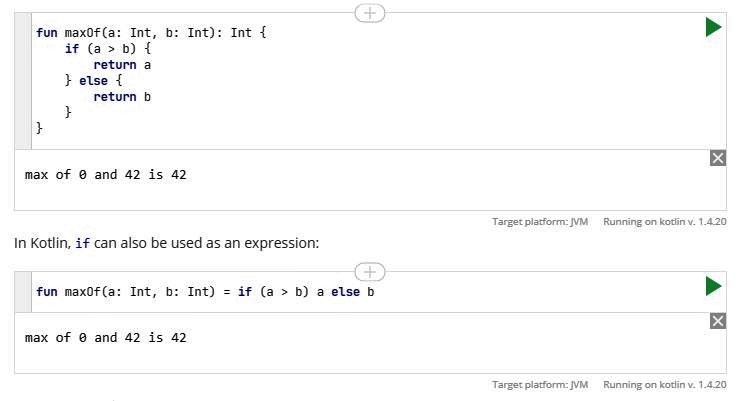
In Android Kotlin, conditional expressions are usually defined by “if,” followed by “else.” For instance, you can have a function do something, and then if it returns a specific value, then you can assign it to a label. But if it doesn’t meet that, then it will be assigned to a different one. This is where “else” comes in.
Comments
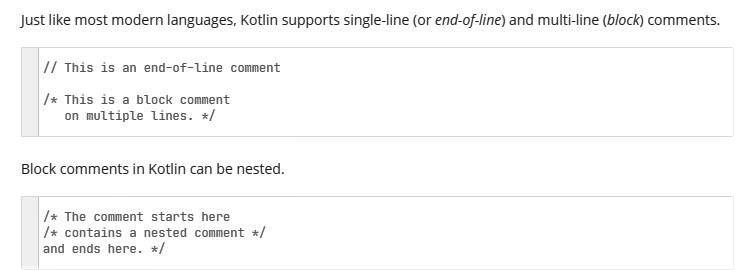
Just like many other programming languages, Kotlin supports comments. These can be in the middle of your code or at the end of it. They don’t really do anything, they’re just there as reminders.
Numbers
The most basic numbers in Kotlin are Int, Long, Float, and Double. Int and Long denote whole numbers. Meanwhile, Float and Double denote decimal numbers. If you’re writing a code using big numbers, use Float and Double instead of Int and Long.
Booleans
Booleans denote either a true value or a false one. To use a Boolean variable, type “= true” or “= false” in your code.
Making Your First Kotlin App on Android Studio
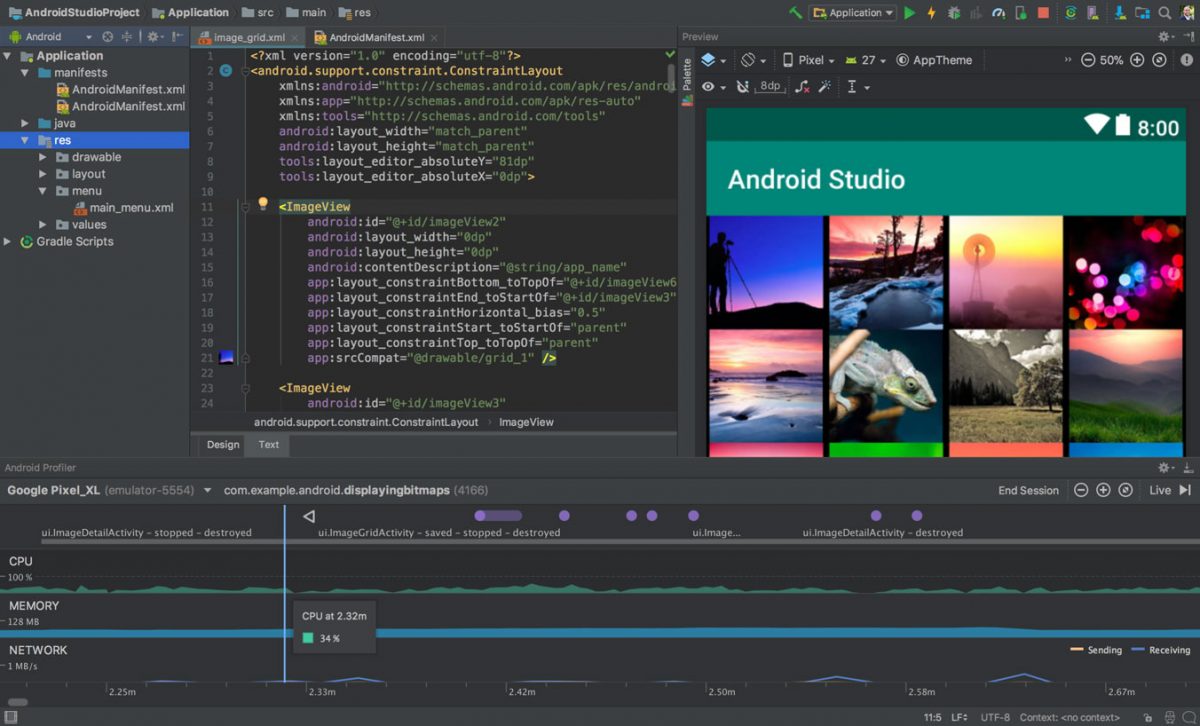
We’ve given you some of the basic building blocks for Kotlin. Now it’s time to learn how Kotlin integrates with Android Studio. First, open Android Studio and start a new project. It will suggest a few project templates, so choose any you like and play around with whichever catches your eye. After choosing a template, it will ask you to choose your language. Make sure you choose Kotlin.
In Android Studio, go to Tools, then click on AVD manager. Then, tap on +Create Virtual Device and just choose a phone model. Click on Finish, and the AVD manager now shows your added virtual device.
You can run your project on the emulator by clicking on Run and then Run app. Then, simply select the device that you just added. From here, you can see that your app is now running on the emulator.
Now that you can see how your project runs on the emulator, it’s time to make some modifications to your code. Every time you make changes to your code, be sure to run it on Android Studio to see how the changes affect your app.
Final Word
Now that we’ve given you the building blocks to make your own app using Android Kotlin, it’s time for you to make the app you want to make. It might be hard and full of trial and error, but with a willingness to learn and a lot of effort, you can make your dream app a reality.