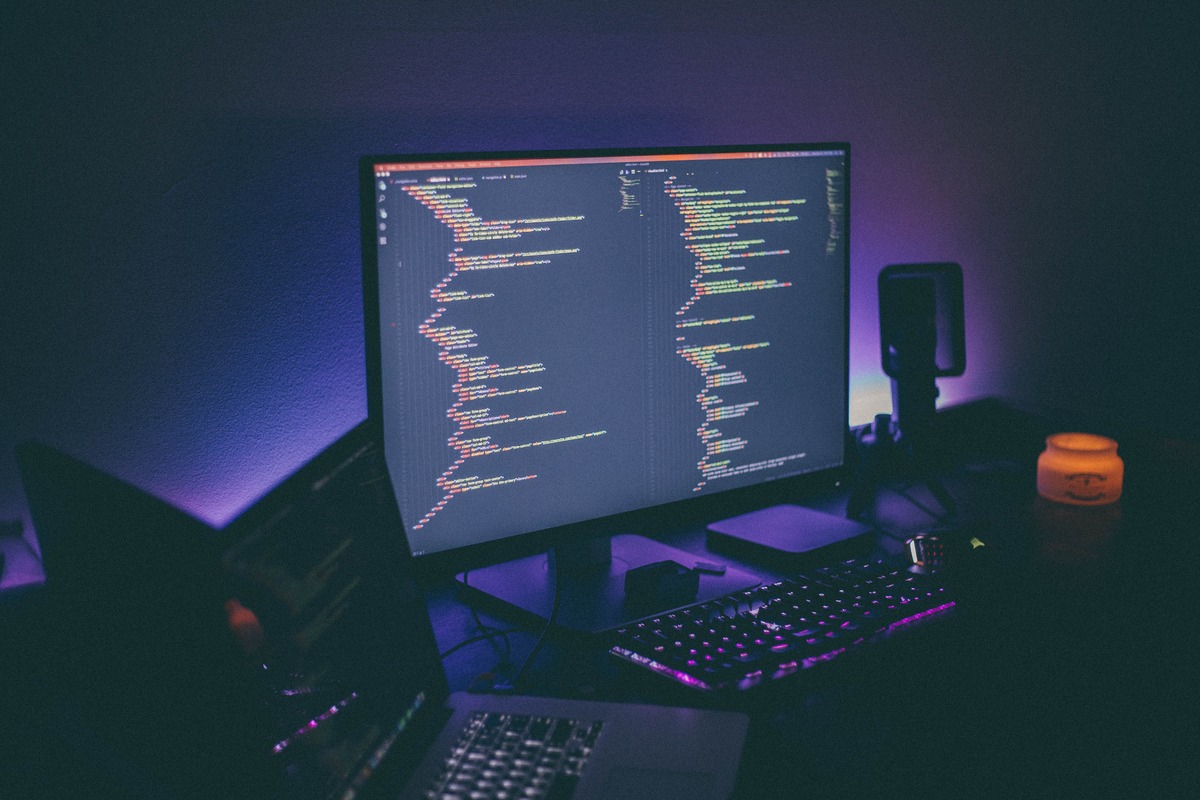
Are you looking for ways to optimize your data storage while working with Python? In this article, we will explore various techniques to save data efficiently in Python. Whether you are dealing with large datasets or want to minimize storage space, understanding the best practices for data storage is essential. From using built-in data structures like lists and dictionaries to leveraging external libraries such as Pandas and NumPy, we will cover different approaches to save data in Python. By implementing these techniques, you can improve the performance of your Python programs and ensure effective data management. So, let’s dive into the world of data storage in Python and learn how to save data effectively.
Inside This Article
- Methods for Saving Data in Python
- Saving Data in Text Files
- Saving Data in CSV Files
- Saving Data in JSON Files
- Saving Data in Databases
- Conclusion
- FAQs
Methods for Saving Data in Python
When working with Python, you may often need to save data for future use or analysis. Fortunately, Python provides several methods to accomplish this task efficiently. In this article, we will explore some of the most common methods for saving data in Python.
1. Saving Data in Text Files
One of the simplest ways to save data in Python is by writing it to a text file. Text files are easy to create and can store data in a human-readable format. You can use the built-in open()
function to create a file object and the write()
method to write data to the file.
2. Saving Data in CSV Files
CSV (Comma-Separated Values) files are commonly used for storing tabular data. Python provides the csv
module, which makes it easy to read and write data in CSV format. You can use the writer
object from the csv
module to write data to a CSV file. It allows you to specify delimiters, quote characters, and other options for customizing the file format.
3. Saving Data in JSON Files
JSON (JavaScript Object Notation) is a popular data interchange format. It provides a lightweight and easy-to-read representation of structured data. Python includes the json
module, which allows you to serialize Python objects into JSON format and vice versa. You can use the dump()
or dumps()
functions to save data as JSON.
4. Saving Data in Databases
If you are working with large amounts of data or need to perform complex queries, it might be more efficient to save the data in a database. Python supports various database management systems such as SQLite, MySQL, and PostgreSQL. You can use database APIs like SQLite3 or SQLAlchemy to connect to a database, create tables, and insert data for later retrieval.
Each method for saving data in Python has its own advantages and use cases. Consider the nature of your data, the required format, and the tools you are comfortable using when deciding which method to implement. By effectively saving data in Python, you can enhance your workflow, enable data analysis, and facilitate future development.
Saving Data in Text Files
One of the simplest ways to save data in Python is by writing it to a text file. This method is useful for storing data that is simple and doesn’t require complex data structures or formatting. To save data in a text file, you can use the built-in open()
function in Python.
Here’s an example of how to save data in a text file:
python
# Open the file in write mode
file = open(‘data.txt’, ‘w’)
# Write data to the file
file.write(‘Hello, World!\n’)
file.write(‘This is some data.’)
# Close the file
file.close()
In this example, we open the file named ‘data.txt’ in write mode by passing ‘w’ as the second argument to the open()
function. Then, we use the write()
method of the file object to write data to the file. Each call to write()
appends the data to the file. We use the \n
character to add a new line between the two lines of data.
Once we are done writing data to the file, it is important to close the file using the close()
method. This ensures that any changes made to the file are saved.
When you run this code, a new text file named ‘data.txt’ will be created in the same directory as your Python script. The file will contain the two lines of data that we wrote.
Saving data in text files is convenient for simple data storage. However, if you need to work with more complex data structures or if you want to perform advanced operations on your data, you may want to consider using other methods such as saving data in CSV or JSON files, or even storing it in databases.
Saving Data in CSV Files
CSV (Comma Separated Values) is a commonly used file format for storing tabular data. It provides a simple way to save data in plain text, where each value is separated by a comma. CSV files can be easily processed and manipulated using various programming languages, including Python. Here’s how you can save data in CSV files using Python:
1. Import the necessary modules:
To work with CSV files, you need to import the `csv` module in Python. You can do this by using the `import` statement:
python
import csv
2. Prepare the data:
Before saving the data, you need to have the data ready in a suitable format. Typically, data is represented as a list of dictionaries, where each dictionary represents a row in the CSV file. Each dictionary should have keys corresponding to the column names and values corresponding to the data in that column. For example:
python
data = [
{‘Name’: ‘John’, ‘Age’: 25, ‘Country’: ‘USA’},
{‘Name’: ‘Emily’, ‘Age’: 30, ‘Country’: ‘Canada’},
{‘Name’: ‘David’, ‘Age’: 35, ‘Country’: ‘UK’}
]
3. Open the CSV file in write mode:
To save the data in a CSV file, you need to open the file in write mode. You can use the `open()` function to open the file, specifying the file path and the write mode (‘w’):
python
with open(‘data.csv’, ‘w’, newline=”) as csvfile:
# Write the data to the CSV file
4. Create a CSV writer object:
Once the file is opened, you need to create a CSV writer object using the `csv.writer()` function. This object will allow you to write data to the CSV file. Pass the file object and any required parameters:
python
csv_writer = csv.writer(csvfile)
5. Write data to the CSV file:
You can use the `writerow()` method of the CSV writer object to write each row of data to the CSV file. Iterate over your data list and call `writerow()` for each dictionary:
python
for row in data:
csv_writer.writerow(row.values())
6. Close the CSV file:
Finally, don’t forget to close the CSV file once you’re done writing the data. You can do this using the `close()` method of the file object:
python
csvfile.close()
By following these steps, you can save data in a CSV file using Python. CSV files are widely used for data analysis, and this method provides a simple and efficient way to store and manipulate tabular data.
Saving Data in JSON Files
JSON (JavaScript Object Notation) is a popular data format used for storing and transmitting data. It is widely supported in Python and is a great choice for saving structured data.
Python provides the `json` module, which allows you to easily work with JSON data. You can use the `json.dump()` function to save data in JSON format to a file.
Here is an example of how to save data in JSON format:
import json
data = {
“name”: “John Doe”,
“age”: 25,
“city”: “New York”
}
with open(“data.json”, “w”) as json_file:
json.dump(data, json_file)
In the above example, we create a dictionary called `data` that contains some sample data. We then open a file called “data.json” in write mode using the `open()` function, and pass it as a parameter to `json.dump()`. This function converts the `data` dictionary into JSON format and writes it to the file.
You can also specify additional parameters when using `json.dump()`. For example, you can use the `indent` parameter to specify the number of spaces to use for indentation:
with open(“data.json”, “w”) as json_file:
json.dump(data, json_file, indent=4)
This will result in a neatly indented JSON file, which can be easier to read and work with.
To load and access the saved JSON data, you can use the `json.load()` function:
with open(“data.json”, “r”) as json_file:
loaded_data = json.load(json_file)
print(loaded_data[“name”]) # Output: John Doe
In the above example, we open the “data.json” file in read mode using `open()`, and then use `json.load()` to load the JSON data into a dictionary called `loaded_data`. We can then access the values in the dictionary as usual.
Saving data in JSON format is a versatile and efficient way to store and access structured data in Python. Whether you’re working with small or large datasets, JSON files provide a convenient solution for saving and retrieving data.
Saving Data in Databases
One of the most powerful and efficient ways to save data in Python is by using databases. Databases allow for structured storage and retrieval of data, making them ideal for applications that require complex data management.
In Python, there are several database management systems (DBMS) that you can use, such as SQLite, MySQL, PostgreSQL, and MongoDB. Each DBMS has its own unique features and advantages, so it’s important to choose the one that best fits your project requirements.
To save data in a database, you will need to establish a connection to the database server using appropriate credentials. Once connected, you can create a table that defines the structure of your data. Each column in the table represents a specific data attribute, while each row represents an individual data entry.
Once the table is set up, you can start inserting data into the database. This can be done using SQL (Structured Query Language) statements, which allow you to insert, update, and retrieve data from the database. Python provides several libraries that simplify working with databases, including SQLite3, MySQLdb, psycopg2, and pymongo.
When saving data in databases, it’s important to consider data normalization and integrity. Normalization helps eliminate redundancy and ensure data consistency, while integrity constraints enforce rules for maintaining data accuracy and integrity.
Another advantage of using databases is the ability to perform advanced queries and data manipulation. You can use SQL to filter, sort, aggregate, and join data from multiple tables. This makes it easier to extract valuable insights and perform complex data analysis.
Databases also provide mechanisms for data backup and recovery, ensuring the safety and security of your data. By implementing regular backups, you can restore your database in the event of data loss or system failure.
Overall, saving data in databases offers a robust and scalable solution for managing and organizing data in Python. Whether you’re working on a small project or a large-scale application, databases provide the necessary tools and features to handle data efficiently.
Conclusion
In conclusion, learning how to save data in Python is an essential skill for any programmer. By understanding different data storage methods such as text files, CSV files, and databases, you can effectively store and retrieve data to enhance your applications and analysis. Whether you need to save user input, log data, or process large datasets, Python provides a range of libraries and modules to efficiently handle your data storage needs. Remember to choose the appropriate method based on the nature and size of your data, and consider factors such as security, scalability, and ease of use. With the knowledge gained from this guide, you can confidently store and manipulate data in Python, empowering you to create robust and successful applications.
FAQs
1. Why is saving data important in Python?
Saving data in Python is crucial for various reasons. Firstly, it allows you to store and retrieve information for future use. Secondly, it enables data persistence, ensuring that your data remains intact even after the program terminates. Lastly, saving data allows for data analysis and manipulation, making it easier to derive insights and make informed decisions.
2. What are the different ways to save data in Python?
Python offers several methods to save data. The most common ones include writing data to files, saving data in databases, using serialization to save and load objects, and utilizing external libraries such as Pandas for advanced data storage and manipulation.
3. How can I save data to a file in Python?
To save data to a file in Python, you can use the built-in open
function to open a file in write mode. Then, you can write the data to the file using the file’s write method. Finally, don’t forget to close the file to ensure the data is properly saved. Alternatively, you can use the csv
module to save data in CSV format or the json
module for saving data in JSON format.
4. How do I save data in a database using Python?
Python provides various database libraries, such as SQLite, MySQL, and PostgreSQL, to save data in databases. You can establish a connection to the database, execute SQL queries to create tables or insert data, and commit the changes to save the data permanently. Additionally, ORMs (Object-Relational Mapping) like SQLAlchemy provide a high-level, Pythonic way to interact with databases and save data efficiently.
5. Can I save objects and their data in Python?
Yes, you can save objects and their data in Python using serialization techniques. The pickle
module is a built-in Python module that allows you to serialize and save objects to a file. You can pickle objects by converting them into a byte stream and then save the byte stream to a file. Similarly, you can load and unpickle objects from the file to recreate the original object and its data.