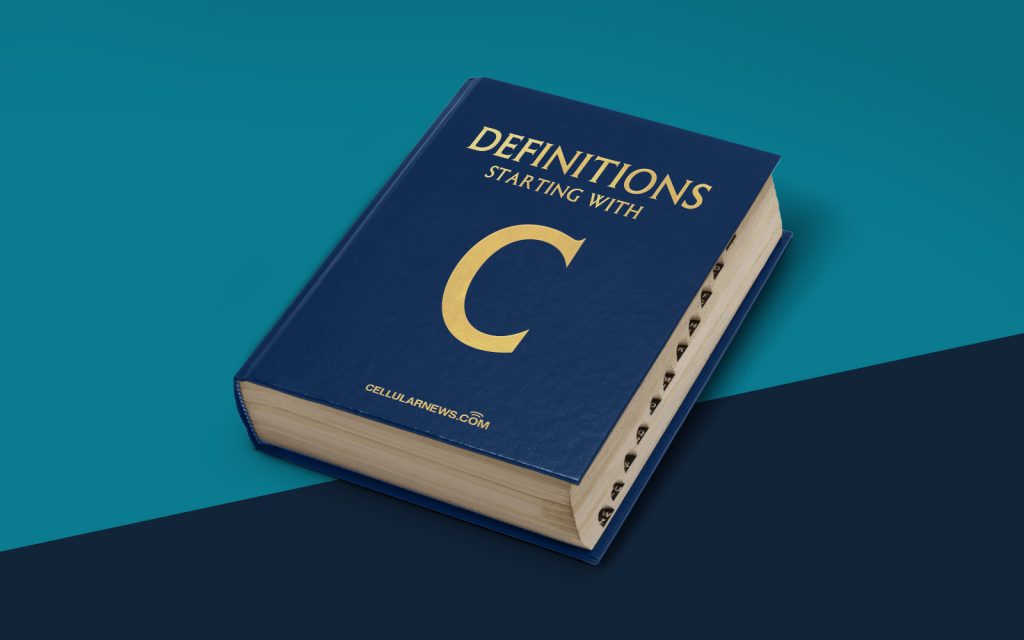
What is Cast in C#?
Cast in C# is a powerful concept that allows developers to convert an object of one type to another compatible type. In simple terms, casting is the process of changing the type of an object from one to another.
When working with C# programming language, you may come across situations where you need to convert certain types to perform specific operations. This is where casting comes into play.
Key Takeaways:
- Casting in C# allows you to convert an object from one type to another compatible type.
- Casting is used when you need to perform operations that are valid only for specific types.
Let’s dive deeper into the concept of casting and explore its various uses and implications.
Why Do We Need Casting in C#?
Casting becomes necessary in C# when you want to:
- Perform operations that are supported only by a specific type.
- Access additional fields or methods present in the target type.
- Change the behavior or representation of an object by converting it to a different type.
Types of Casting in C#
In C#, there are two types of casting: implicit casting and explicit casting.
Implicit Casting
Implicit casting, also known as widening conversion, occurs when there is no possibility of data loss during the type conversion. C# automatically performs implicit casting without requiring any explicit conversion syntax. For example:
int number = 10;
double decimalNumber = number; // Implicit casting from int to double
In the above example, C# implicitly casts the integer value “10” to a double value “10.0” because no data loss occurs during the conversion.
Explicit Casting
Explicit casting, also known as narrowing conversion, is required when there is a possibility of data loss during the type conversion. It involves explicitly specifying the desired type during the conversion. For example:
double decimalNumber = 10.5;
int number = (int)decimalNumber; // Explicit casting from double to int
In the above example, explicit casting is used to convert the double value “10.5” to an int value “10”. Since narrowing conversion may result in data loss (the fractional part is truncated), we need to explicitly specify the desired type during the conversion.
Conclusion
In summary, casting is an essential concept in C# that allows developers to convert objects from one type to another. It is crucial when you need to perform operations specific to a particular type or access additional fields and methods of the target type. Understanding the types of casting, implicit and explicit, is vital to ensure accurate and efficient type conversions.
Key Takeaways:
- Casting in C# allows you to convert an object from one type to another compatible type.
- Casting is used when you need to perform operations that are valid only for specific types.
So, the next time you encounter a scenario where a type conversion is required, remember the power and flexibility of casting in C#.