Many Android apps wouldn’t function as well without user-based decision-making. That’s because most apps require the user’s input on whether to proceed with certain actions or not. Thankfully, it’s easy to ask for user input if the developer makes a simple Android AlertDialog. But what is it and how does it work? Here’s what you need to know.
What Is Android AlertDialog?
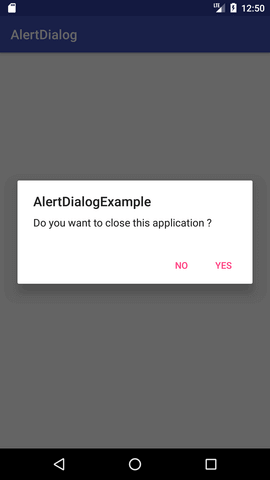
Android AlertDialog is a small pop-up message window that overlays various Android apps. It could be a message prompt just to inform you of the app’s current context. For example, it could be a message notifying you that the app has stopped responding as a prompt for you to force-quit and restart it.
Conversely, the AlertDialog Android prompt might occasionally ask for a response or user input. This is used for apps that need proper consent to run certain tasks. Alternatively, the app could also run different tasks based on the user’s input.
One common example would be an app asking for various permissions. This could be anything from asking for permission to use cookies or access the user’s location data. The app could also ask to proceed to the next page or stay on the current page. Regardless of the purpose, it is a useful tool that nearly all apps use.
Android AlertDialog Uses
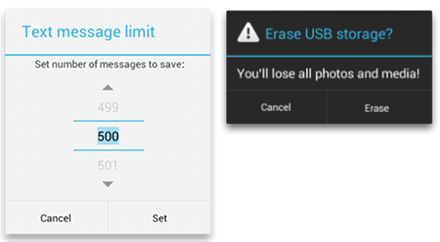
Through the course of your daily interactions with Android apps, you may not notice its boxes often. However, while they don’t particularly stand out to regular users, they’re fairly common among many apps.
There are numerous use cases for an Android AlertDialog. For example, most apps will flash a confirmation box when you exit on them. That’s because you may accidentally press the exit button without intending to on most apps. App developers mitigate this problem by flashing an alert for confirmation first.
The same is true when it comes to deleting files, folders, and other content or media from multiple applications. Deletion is usually permanent, especially if the app doesn’t have a temporary trash bin for deleted files.
There are yet other apps that use Android AlertDialog to obtain permissions. For example, many apps you find on the app store require permission to access certain data. These can include everything about the user and their phone usage.
One common permission apps ask for is location data if the app is mainly location-based, as is the case with Google Maps or Uber. Others ask for permission to access the phone’s media files before you upload photos to social media apps.
As much as this function is useful, there are certain limitations to it, such as how many buttons can exist in one pop-up. Moreover, Android AlertDialog boxes can only flash so much information in any given space.
Hence, developers might use separate screens to display long paragraphs of text. Nonetheless, Android AlertDialog boxes are still useful as long as they’re concise.
How To Use AlertDialog In Android?
Now that you know what Android AlertDialog is, it’s time to learn how to use it. This is very important if you’re an app developer looking to create even the simplest of apps. That’s because nearly any type of app uses Android custom AlertDialog prompts. We’ll begin with the components.
Components of Android AlertDialog

Android AlertDialog is composed of three main parts: the title, content, and action buttons. The title usually displays what the message is about in a word or short phrase. While this is a standard component, it’s still an optional field that not all alerts use.
The message itself is what the user will pay the most attention to. It could contain a prompt, question, list, or any other content that the developer might want to communicate.
Finally, the action buttons are clickable fields that the user can tap to dismiss the prompt. These buttons usually come in three types: positive, negative, and neutral. Let’s talk about each and the functions they perform.
Firstly, the Positive action button accepts the action. It’s usually displayed as “OK” or “Yes”. Once it is pressed, the user allows the app to perform the action it prompted. For example, it could be saying yes to allowing access to media files, location data, etc.
Secondly, the Negative action button denies or cancels the action. It usually comes in the form of a “No” or “Cancel” button. If the user doesn’t want to provide consent to the Android AlertDialog, they will press the negative action button.
Lastly, the Neutral action button is a way for the user to put off the decision for later. Usually, a “Later” button is displayed for this purpose.
Android AlertDialog Functions And Method Type
Not all prompts are the same and will vary with each app. Most developers like to customize their alerts to fit the context and app. It is thus important to understand the different functions and methods to customize them. If you’re not sure where to start, refer to the table below for a quick guide on method types:
Method Type | Description |
---|---|
setIcon(Drawable icon) | This sets the prompt’s icon |
setCancelable(boolean cancelable) | Sets whether or not the Android AlertDialog can be canceled |
setMessage(CharSequence message) | This function will set the message that the Android AlertDialog displays |
setOnCancelListener(DialogInterface.OnCancelListener onCancelListener) | Sets the callback if it is cancelled |
setTitle(CharSequence title) | This sets the title of the AlertDialog |
getListView() | Gets the AlertDialog’s listview |
setMultiChoiceItems(CharSequence[] items, boolean[] checkedItems, DialogInterface.OnMultiChoiceClickListenerlistener) | Sets the content in the AlertDialog as a list of items, with the listener notifying the selected option |
How To Set AlertDialog In Android

Creating it is fairly simple and straightforward when you know what you’re doing. However, if you’re not sure where to begin, here’s a quick guide on how to set it up.
#Step 1: Android AlertDialog Builder
Firstly, you need to make the Android AlertDialog builder an object. This is an inner class of AlertDialog and is fairly simple to create. Just use the following syntax:
AlertDialog.Builder alertDialogBuilder = new AlertDialog.Builder(this);
#Step 2: Creating Action Buttons
Secondly, you can set the action buttons you want to appear. You have to set these buttons with the AlertDialogBuilder class. Here are samples of the syntax for positive, negative, and neutral action buttons:
alertDialogBuilder.setPositiveButton(CharSequence text, DialogInterface.OnClickListener listener); alertDialogBuilder.setNegativeButton(CharSequence text, DialogInterface.OnClickListener listener); alertDialogBuilder.setNeutralButton(CharSequence text, DialogInterface.OnClickListener listener);
#Step 3: Adding Method Types And Customizations
Thirdly, you can use any of the different method types previously listed to add and customize your Android AlertDialog box. Feel free to make it cancellable, set the icon, provide a callback, and everything else you think is necessary.
#Step 4: Creating The Android AlertDialog
Lastly, you can finally create the Android AlertDialog by using the create() method of the builder class. You can do so with the following syntax:
AlertDialog alertDialog = alertDialogBuilder.create(); alertDialog.show();
#Step 5: Testing
Afterward, you should be able to see your Android AlertDialog successfully shown on the screen. You can try running the application on your Android device or through the emulator within Android Studio.
Of course, what we’ve provided so far are only the basic syntaxes to create a regular Android AlertDialog. It’s up to you to add the different parameters and results based on user input. Hence, you have to remember to code what happens when the positive, negative, and neutral buttons are pressed. Moreover, you also have to provide for what happens when the user presses the back button on their Android device.
The Final Word
Android AlertDialog boxes are nearly essential components of developing any kind of Android app. They help you ask for input without moving to a new page and provide prompts and alerts for users. They are thus very versatile and useful if applied appropriately.
Thankfully, despite it being such a crucial part of many apps, creating an Android AlertDialog is a fairly simple process. All you need is to decide which method types to use and employ the right syntaxes in your code.