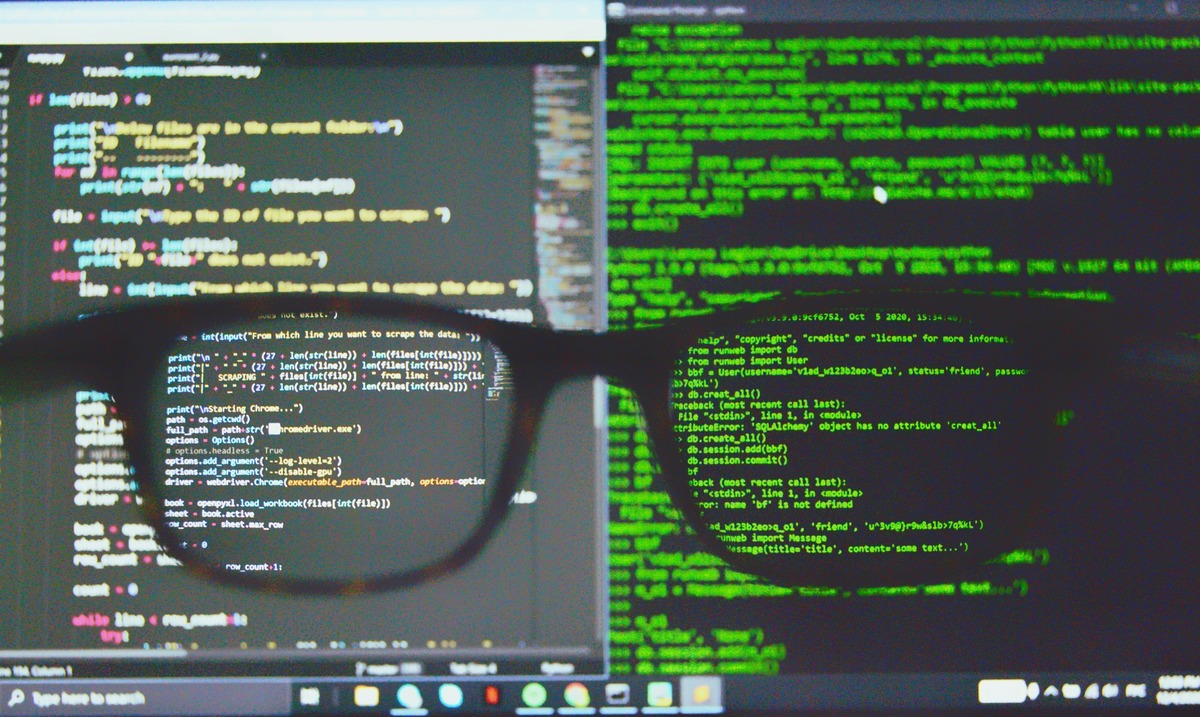
In the world of data analysis and machine learning, it’s not uncommon to encounter datasets with categorical variables. These variables can pose a challenge when it comes to analyzing and modeling data, as many machine learning algorithms require numerical data for processing. However, with the help of Python, converting categorical data to numerical data has never been easier.
In this article, we will explore various techniques and approaches to convert categorical data into numerical data using Python. We will discuss methods like label encoding, one-hot encoding, and feature hashing, highlighting their pros and cons. By the end of this article, you will have a solid understanding of how to transform categorical data into a format that can be readily used in machine learning algorithms.
Inside This Article
- Overview of Categorical and Numerical Data
- Techniques for Converting Categorical Data to Numerical Data in Python
- Label Encoding
- One-Hot Encoding
- Ordinal Encoding
- Binary Encoding
- Conclusion
- FAQs
Overview of Categorical and Numerical Data
Before diving into the techniques for converting categorical data to numerical data in Python, it’s important to understand the difference between categorical and numerical data.
Categorical data refers to data that can be divided into distinct categories or groups. Examples of categorical data include gender (male/female), color (red/blue/green), and level of education (high school/college/graduate). Categorical data is usually represented as labels or strings.
Numerical data, on the other hand, consists of numbers and can be further divided into continuous or discrete data types. Continuous numerical data can take any value within a range, such as height or weight, while discrete numerical data can only take specific, distinct values, such as the number of siblings or the grade level in school.
The goal of converting categorical data to numerical data is to transform non-numeric labels into a format that can be processed by machine learning algorithms or statistical models. This conversion allows us to apply mathematical operations and calculations to the data, enabling us to gain insights and make predictions.
There are several techniques available in Python for converting categorical data to numerical data, each with its own advantages and use cases. By understanding these techniques, you can choose the most suitable approach for your specific data and analysis requirements.
Techniques for Converting Categorical Data to Numerical Data in Python
When working with data in Python, it is common to encounter categorical data – variables that take on a limited number of distinct categories. However, many machine learning models and algorithms require numerical data as input. So, converting categorical data to numerical data becomes essential. In this article, we will explore different techniques for converting categorical data to numerical data in Python.
1. Label Encoding: Label encoding is a simple technique where each unique category in the categorical variable is assigned a numerical value. This is done by replacing each category with a different integer value. For example, if we have a categorical variable “Color” with categories “Red,” “Blue,” and “Green,” we can assign values 0, 1, and 2 respectively.
2. One-Hot Encoding: One-Hot encoding is another popular technique used in data preprocessing. In this technique, instead of assigning numerical values, we create binary columns for each category. Each original category is transformed into a separate binary column with 0s and 1s indicating the presence or absence of that category. This technique is useful when there is no ordinal relationship between the categories.
3. Ordinal Encoding: Ordinal encoding is suitable when the categorical variable has an inherent order or hierarchy among the categories. In this technique, the categories are assigned numerical values based on their order. For example, if we have a variable “Size” with categories “Small,” “Medium,” and “Large,” we can assign values 0, 1, and 2 respectively.
4. Binary Encoding: Binary encoding is a technique that combines aspects of label encoding and one-hot encoding. It represents the categories with binary code, which helps in reducing the dimensions of the feature set. It works by converting each category into binary representation and storing it as separate binary columns.
These are some of the commonly used techniques for converting categorical data to numerical data in Python. The choice of technique depends on the nature of the data and the requirements of the machine learning model. By converting categorical data to numerical data, we can make the data suitable for training machine learning models and improving their performance.
Label Encoding
Label Encoding is a technique used to convert categorical variables into numerical values. It assigns a unique numerical label to each category in the variable. This encoding method is commonly used in machine learning algorithms as they typically require numerical input.
The process of label encoding involves the following steps:
- Import the necessary libraries, such as
pandas
. - Load the dataset that contains the categorical variable.
- Create an instance of the
LabelEncoder
class from thepandas
library. - Fit the label encoder to the variable using the
fit()
method. - Transform the variable using the
transform()
method to convert the categories into numerical labels.
Here is an example of how to perform label encoding in Python using the pandas
library:
python
import pandas as pd
from sklearn.preprocessing import LabelEncoder
# Load the dataset
data = pd.read_csv(“data.csv”)
# Create an instance of LabelEncoder
le = LabelEncoder()
# Fit and transform the categorical variable
data[“category_encoded”] = le.fit_transform(data[“category”])
Label encoding can be used when the categories have an inherent ordering or when the categorical variable has a large number of distinct values. However, it is important to note that label encoding may introduce ordinality in the data, which may not be desired in some cases.
Label encoding is a simple and effective way to convert categorical data into numerical form. However, it is not suitable for variables where the categories are not ordered. In such cases, other encoding techniques like one-hot encoding or ordinal encoding can be considered.
Next, let’s explore another technique for converting categorical data to numerical data in Python: one-hot encoding.
One-Hot Encoding
One-hot encoding is another popular technique for converting categorical data into numerical data in Python. It works by creating binary columns for each unique category and assigning a value of 1 or 0 to indicate the presence or absence of that category in each observation.
To perform one-hot encoding in Python, you can use the pandas.get_dummies()
function. This function automatically identifies categorical columns in a dataframe and converts them into one-hot encoded columns.
Here’s an example:
# Import the required libraries
import pandas as pd
# Create a dataframe with categorical data
data = {'Animal': ['Dog', 'Cat', 'Dog', 'Bird', 'Cat']}
df = pd.DataFrame(data)
# Perform one-hot encoding
one_hot_encoded = pd.get_dummies(df)
print(one_hot_encoded)
The resulting output will show each category as a separate column with binary values indicating the presence or absence of that category in each observation. For example, the ‘Animal_Dog’ column will have a value of 1 for rows where the animal is a dog, and 0 for rows where the animal is not a dog.
One-hot encoding is particularly useful when there is no inherent order or hierarchy among the categories. It allows machine learning models to treat each category as a distinct, independent feature.
However, one drawback of one-hot encoding is that it can potentially lead to a high number of features, especially if the categorical variable has a large number of unique categories. This can make the dataset more complex and increase the computational resources required.
In such cases, it may be necessary to consider other encoding techniques or apply dimensionality reduction techniques to reduce the number of features.
Ordinal Encoding
Ordinal encoding is another technique used to convert categorical data into numerical data in Python. It involves assigning a unique numerical value to each category based on their order or rank. In other words, ordinal encoding preserves the ordinal relationship between the categories.
To perform ordinal encoding, you need to follow these steps:
- Assign a numerical value to each category based on their order or rank. For example, if you have a categorical variable “Size” with categories “Small,” “Medium,” and “Large,” you can assign the values 1, 2, and 3 respectively.
- Create a mapping dictionary that maps each category to its corresponding numerical value.
- Replace the categories in your dataset with their respective numerical values using the mapping dictionary.
Here is an example of how to perform ordinal encoding in Python:
python
from sklearn.preprocessing import OrdinalEncoder
# Sample data
data = [[“Small”], [“Medium”], [“Large”], [“Small”], [“Large”]]
# Create an instance of the OrdinalEncoder
encoder = OrdinalEncoder()
# Fit and transform the data
encoded_data = encoder.fit_transform(data)
print(encoded_data)
The output will be:
python
[[2.]
[1.]
[0.]
[2.]
[0.]]
As you can see, the categories “Small,” “Medium,” and “Large” have been replaced with their respective numerical values.
Ordinal encoding is suitable for categorical variables where the categories have a natural order or rank. However, it’s important to note that the numerical values assigned through ordinal encoding may introduce a bias, as the distances between the values might not accurately represent the differences between the categories. Therefore, it is important to use caution when interpreting the results of models that utilize ordinal encoding.
Overall, ordinal encoding is a simple and effective technique for converting categorical data to numerical data in Python, and it can be a valuable tool in your data preprocessing pipeline.
Binary Encoding
Binary Encoding is another method used to convert categorical data into numerical data in Python. It is particularly useful when dealing with high cardinality categorical variables, where the number of unique categories is large. This technique assigns a unique binary code to each category, making it suitable for machine learning algorithms that require numeric input.
To apply binary encoding, each category is first assigned an integer starting from 0. Then, the integer value is converted into its binary representation. The binary values are then split into different columns, each representing a bit position. This process allows each category to be represented by a combination of 0s and 1s in the binary encoded columns.
The advantage of binary encoding is that it reduces the number of dimensions compared to one-hot encoding, which can be beneficial when dealing with large datasets. Additionally, it captures the ordinal relationship between categories, similar to ordinal encoding. However, it should be noted that binary encoding assumes the categories have an implied order, which may not always be the case.
In Python, the category_encoders
library provides an easy way to implement binary encoding. The BinaryEncoder
class can be used to transform categorical data into binary-encoded features. You can specify the columns to be encoded and fit the encoder to the data, resulting in a transformed DataFrame with binary-encoded columns.
Here is an example of using binary encoding with the category_encoders
library:
from category_encoders import BinaryEncoder
# Define the columns to be encoded
columns_to_encode = ['color', 'size', 'type']
# Create an instance of BinaryEncoder
encoder = BinaryEncoder(cols=columns_to_encode)
# Fit and transform the data
df_encoded = encoder.fit_transform(df)
In the example above, we create an instance of the BinaryEncoder
class, specifying the columns that we want to encode. We then fit the encoder to the data and transform the DataFrame, with the result being a DataFrame with binary-encoded columns for the specified columns.
Binary encoding is a powerful technique for converting categorical data into numerical data in Python. It balances dimensionality reduction with capturing ordinal relationships between categories, making it a useful tool in machine learning projects. By implementing binary encoding, you can transform categorical data into a format that can be easily processed and utilized by various algorithms.
Conclusion
In conclusion, converting categorical data to numerical data is an essential step in many data analysis and machine learning tasks. By encoding categorical variables into numerical representations, we can leverage the full power of mathematical and statistical models to gain insights and make predictions.
In this article, we have explored various methods to convert categorical data to numerical data in Python, including one-hot encoding, label encoding, and ordinal encoding. Each technique has its own advantages and considerations, depending on the nature of the data and the specific requirements of the analysis.
Remember to carefully analyze your data and choose the most appropriate encoding method. It’s important to consider factors such as the number of categories, the relationship between the categories, and the impact on the overall performance of your model.
With the knowledge and tools discussed in this article, you are now equipped to handle categorical data in your Python data analysis projects. Happy coding!
FAQs
1. Why is it necessary to convert categorical data to numerical data?
Converting categorical data to numerical data is essential for many machine learning algorithms, as most algorithms rely on numerical values. By converting categorical data to numerical data, we can ensure that the data is in a format that can be processed and analyzed by these algorithms.
2. What are some common techniques to convert categorical data to numerical data?
There are several techniques that can be used to convert categorical data to numerical data, including one-hot encoding, label encoding, ordinal encoding, and binary encoding. Each technique has its own advantages and is suitable based on the nature of the categorical data and the requirements of the machine learning problem at hand.
3. What is one-hot encoding?
One-hot encoding is a technique used to convert categorical data into a binary matrix representation. It creates binary columns for each unique value in the categorical feature, where each column represents the presence or absence of that particular value. This technique is useful when there is no inherent ordinal relationship among the categories.
4. What is label encoding?
Label encoding is a technique used to convert categorical data into numerical data by assigning a unique numerical value to each category. This technique is suitable when there is an inherent ordinal relationship among the categories, as the assigned numerical values convey the order of the categories.
5. What is ordinal encoding?
Ordinal encoding is a technique used to convert categorical data into numerical data by assigning a numerical value based on the order of the categories. It is similar to label encoding, but with the addition of preserving the ordinal relationship among the categories. This technique is useful when there is an inherent ordering of the categories, such as low, medium, and high.