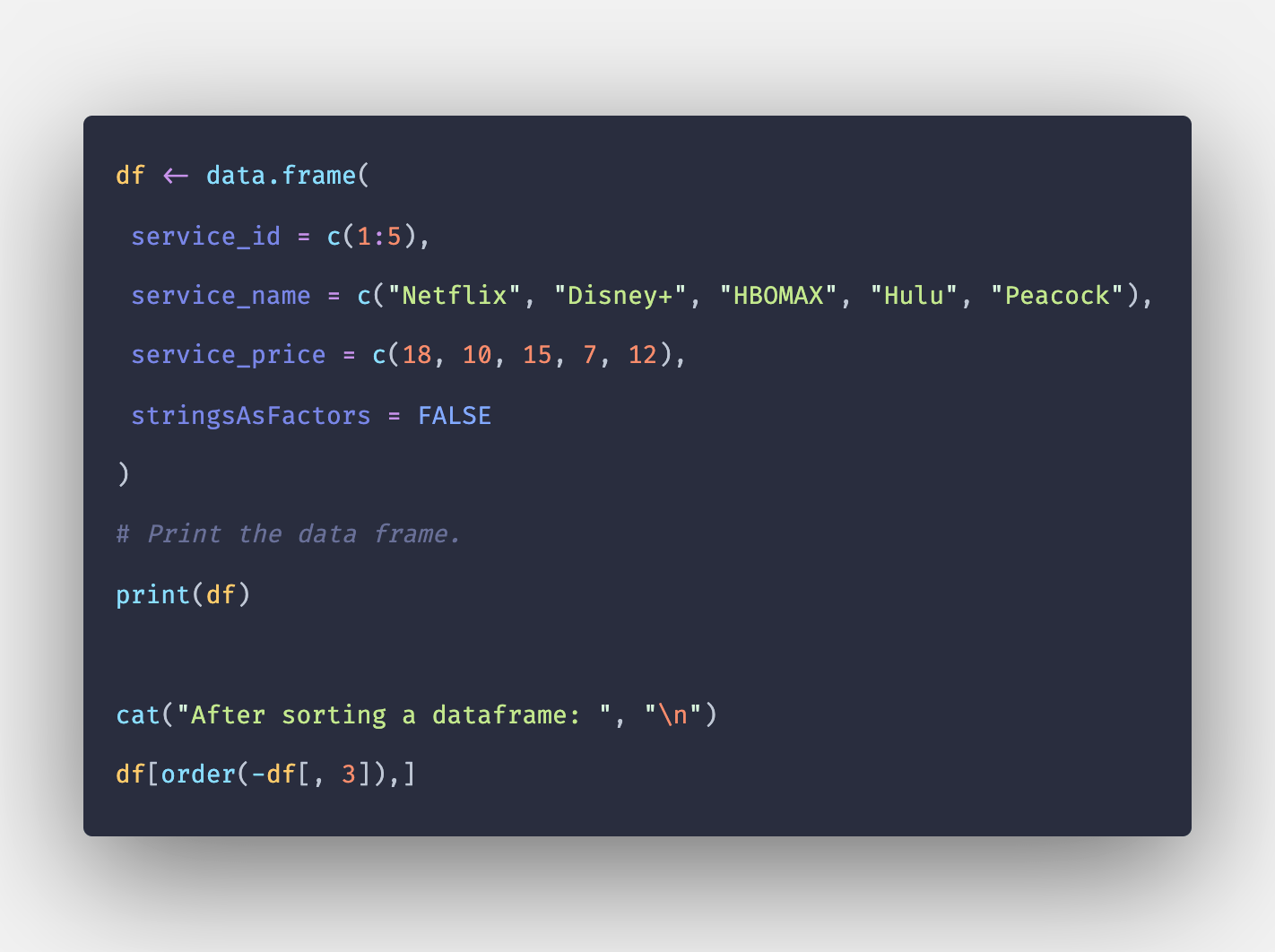
When working with data in R, one of the essential tasks is sorting that data to gain insights and extract valuable information. Sorting data allows us to arrange it in a meaningful order, making it easier to analyze and interpret. Whether you have a large dataset or a smaller one, the ability to sort data in R is incredibly useful.
In this article, we will explore various techniques and functions in R that enable us to sort data efficiently. We will cover sorting by one or multiple columns, sorting in ascending or descending order, and using custom sorting criteria. By the end of this article, you will have a solid understanding of different sorting methods in R and how to apply them to your own data analysis projects.
Inside This Article
- Section 1: Sorting Data in R – Sorting Numeric Data
- Section 1: Sorting Data in R – Sorting Character Data
- Section 1: Sorting Data in R – Sorting Date and Time Data
- Section 2: Sorting Data Frames in R – Sorting Single Column – Sorting Multiple Columns – Sorting Data Frame by a Specific Criteria
- Section 3: Sorting Factors in R
- Section 4: Sorting Lists and Arrays in R
- Conclusion
- FAQs
Section 1: Sorting Data in R – Sorting Numeric Data
In R, sorting numeric data is a common task that can be easily accomplished using built-in functions. The most commonly used function for sorting numeric data is sort()
. This function takes a vector or a dataframe column as input and returns the sorted values in ascending order.
To sort numeric data in descending order, you can use the rev()
function in combination with sort()
. For example, if you have a vector named my_numbers
, you can sort it in descending order using sort(rev(my_numbers))
.
Section 1: Sorting Data in R – Sorting Character Data
Sorting character data in R follows a similar approach to sorting numeric data. You can use the sort()
function to sort character vectors or dataframe columns in alphabetical order.
By default, the sort()
function arranges the elements in ascending order. However, you can also sort them in descending order by using the rev()
function in combination with sort()
. For instance, if you have a vector named my_names
, you can sort it in descending order using sort(rev(my_names))
.
Section 1: Sorting Data in R – Sorting Date and Time Data
Sorting date and time data in R requires the use of the as.Date()
function. This function converts character or numeric data into a Date class object, allowing for accurate sorting based on date and time values.
Once you have converted your data into the Date class object, you can use the sort()
function to sort the dates in ascending order. To sort the dates in descending order, you can combine the sort()
function with the rev()
function.
It’s important to note that you may need to customize the format of your date and time data before using the as.Date()
function, depending on the formatting of your data. If your data is not in a recognized date format, you may need to use additional functions, such as strptime()
, to convert it into a proper format before sorting.
Section 2: Sorting Data Frames in R – Sorting Single Column – Sorting Multiple Columns – Sorting Data Frame by a Specific Criteria
Sorting data frames in R is a fundamental operation that allows us to organize and analyze our data more effectively. In this section, we will explore different methods to sort data frames based on various criteria.
Sorting Single Column
To sort a data frame by a single column, we can use the order()
function. This function returns the indices that would sort the column in ascending order. We can then use these indices to rearrange the rows of the data frame.
Here’s an example:
R
# Create a data frame
df <- data.frame(name = c("Alice", "Bob", "Charlie"),
age = c(25, 30, 28),
salary = c(50000, 60000, 55000))
# Sort the data frame by the 'age' column
df_sorted <- df[order(df$age), ]
In this example, the data frame is sorted in ascending order based on the ‘age’ column. The resulting data frame, df_sorted
, will have the rows arranged from youngest to oldest.
Sorting Multiple Columns
Sorting a data frame by multiple columns requires specifying the order of columns to sort. We can achieve this by passing a vector of column names to the order()
function.
Here’s an example:
R
# Sort the data frame by multiple columns
df_sorted <- df[order(df$age, df$salary), ]
In this example, the data frame is sorted by the ‘age’ column first and then by the ‘salary’ column. This ensures that the rows are arranged in ascending order of age, and for rows with the same age, in ascending order of salary.
Sorting Data Frame by a Specific Criteria
Sometimes, we may need to sort a data frame based on a specific criteria that does not directly correspond to any column. In such cases, we can use the arrange()
function from the dplyr
package.
Here’s an example:
R
# Install and load the dplyr package
install.packages(“dplyr”)
library(dplyr)
# Sort the data frame by a specific criteria
df_sorted <- arrange(df, desc(salary), age)
In this example, the data frame is sorted in descending order of salary, and for rows with the same salary, in ascending order of age. The desc()
function is used to specify the descending order.
By using these techniques, you can easily sort data frames in R based on a single column, multiple columns, or a specific criteria. Sorting your data frames allows for better analysis and visualization, enabling you to gain deeper insights from your data.
Section 3: Sorting Factors in R
In R, factors are used to represent categorical variables. These variables can have a finite set of possible values, such as “male” or “female”, or “low”, “medium”, or “high”. Sorting factors allows us to organize and manipulate data based on these categories. Here, I will explain how to sort factors in R.
Sorting factors in R is straightforward. We can use the sort()
function to sort factors in alphabetical order. Let’s take an example:
R
# Create a factor variable
category <- factor(c("apple", "banana", "cherry", "apple", "banana", "cherry"))
# Sort the factor
sorted_category <- sort(category)
The resulting sorted_category
will have the factors arranged in alphabetical order: “apple”, “apple”, “banana”, “banana”, “cherry”, “cherry”.
If we want to sort the factors in reverse alphabetical order, the sort()
function can be modified using the additional argument decreasing = TRUE
:
R
# Sort the factor in reverse alphabetical order
reverse_sorted_category <- sort(category, decreasing = TRUE)
The reverse_sorted_category
will now have the factors arranged in reverse alphabetical order: “cherry”, “cherry”, “banana”, “banana”, “apple”, “apple”.
Another useful function for sorting factors in R is order()
. The order()
function returns a permutation that will order a vector or factor in ascending order. Let’s see an example:
R
# Create a factor variable
category <- factor(c("apple", "banana", "cherry", "apple", "banana", "cherry"))
# Use order() to get the indices for sorting
sorted_indices <- order(category)
# Sort the factor using the indices
sorted_category <- category[sorted_indices]
In this example, the sorted_indices
will be a vector of indices that represent the order in which the factors should be arranged. By using this vector to subset the category
factor, we obtain the same sorted result as before.
Sorting factors in R allows us to effectively analyze and summarize categorical data. Whether you need to sort factors in alphabetical or reverse alphabetical order, or if you want to use the order of the factors to sort them, R provides flexible functions to meet your needs.
Section 4: Sorting Lists and Arrays in R
In R, lists and arrays are commonly used to store and manipulate multiple values of different data types. Sorting lists and arrays can be helpful when you need to organize your data in a specific order. Let’s explore how you can sort lists and arrays in R.
1. Sorting Lists: Lists in R can contain elements of different types, including vectors, matrices, or even other lists. To sort a list, you can use the sort()
function along with the sapply()
function to apply the sorting operation to each element of the list. Here’s an example:
# Create a list
my_list <- list(c(3, 1, 4), c("orange", "apple", "banana"), c(FALSE, TRUE, FALSE))
# Sort the list elements
sorted_list <- sapply(my_list, sort)
# Print the sorted list
print(sorted_list)
This code will sort each element of the list in ascending order. You can modify the code to sort the elements in descending order by using the decreasing = TRUE
argument in the sort()
function.
2. Sorting Arrays: Arrays are multi-dimensional structures in R that can be sorted along specific dimensions. To sort an array, you can use the order()
function along with the apply()
function to apply the sorting operation to each dimension of the array. Here’s an example:
# Create an array
my_array <- array(1:12, dim = c(3, 4))
# Sort the array along rows
sorted_array_rows <- apply(my_array, 1, sort)
# Sort the array along columns
sorted_array_cols <- apply(my_array, 2, sort)
# Print the sorted arrays
print(sorted_array_rows)
print(sorted_array_cols)
This code will sort the array along the rows and columns, respectively. The resulting sorted arrays will have the elements arranged in ascending order.
In both cases, the sorting is done based on the values of the elements. If you want to sort the lists or arrays based on a specific criterion, you can define a custom comparison function and pass it as an argument to the sorting function.
Sorting lists and arrays in R allows you to organize your data in a meaningful way. Whether you’re working with lists or arrays, the sorting techniques described above will help you effectively rearrange the elements based on your requirements.
Now that you have learned about sorting lists and arrays in R, you can confidently navigate your way through manipulating and organizing your data for further analysis.
Conclusion
In conclusion, sorting data is a fundamental task in data analysis and R provides a wide range of functions and methods for sorting data efficiently. By using the various sorting functions available in R, such as sort(), order(), and rank(), you can easily arrange data in ascending or descending order based on specific variables or criteria.
Sorting data not only helps you organize and make sense of large datasets, but it also allows you to explore patterns, identify outliers, and perform further statistical analysis. With R’s powerful data manipulation and sorting capabilities, you can gain valuable insights and make informed decisions based on your data.
Remember to consider the nature of your data and the specific requirements of your analysis when choosing the appropriate sorting method. Experimenting with different sorting techniques will help you find the most efficient and accurate way to arrange your data for further analysis.
FAQs
Here are some frequently asked questions about sorting data in R:
1. How do I sort data in R?
To sort data in R, you can use the order()
function. This function takes one or more vectors as arguments and returns the index in ascending order. You can then use this index to reorder your data.
2. Can I sort data based on multiple columns?
Yes, you can sort data based on multiple columns using the order()
function. Simply pass multiple vectors as arguments to the function, and it will return the index based on the sorting order across all the columns provided.
3. How can I sort data in descending order?
By default, the order()
function sorts data in ascending order. However, if you want to sort data in descending order, you can use the decreasing
parameter. Set it to TRUE
to sort the data in descending order.
4. What if I want to sort data based on a specific column?
If you want to sort data based on a specific column, you can use the sort()
function in R. This function arranges the elements of a vector in ascending order. You need to specify the vector you want to sort as the argument to the sort()
function.
5. Is it possible to sort a data frame in R?
Yes, you can sort a data frame in R using the order()
function. However, it is important to note that when you use the order()
function on a data frame, it will return the index based on the sorting order of the rows. You can then use this index to reorder the rows of the data frame accordingly.