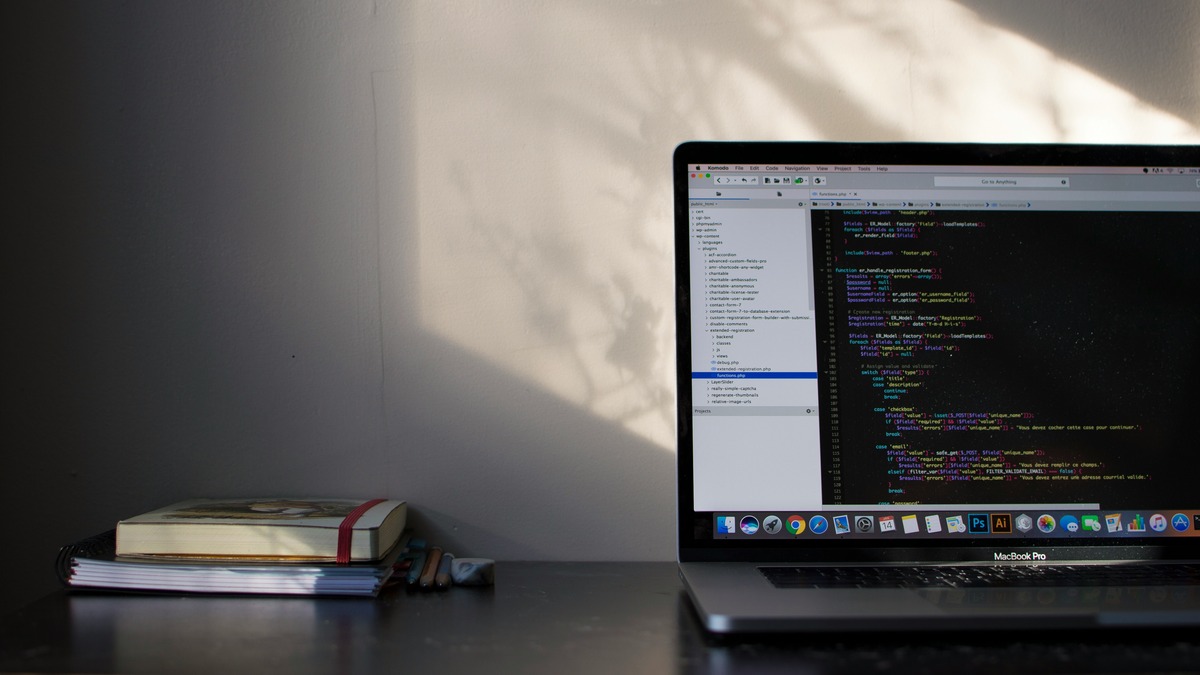
Are you looking to load data into Python for your data analysis or machine learning projects? Loading data is a critical first step in any data-driven project, as it allows you to access and manipulate the data effectively. Whether your data is stored in a CSV file, a database, or an API, Python provides several powerful tools and libraries to facilitate the process.
In this article, we will explore various methods and techniques to load data into Python. We will cover how to import CSV files, connect to databases, and retrieve data using APIs. Additionally, we will discuss popular Python libraries such as Pandas, SQLAlchemy, and Requests, which make data loading and manipulation a breeze.
By the end of this article, you will have a solid understanding of how to load data from different sources into Python and unleash the full potential of your data analysis projects.
Inside This Article
- Importing the necessary libraries and modules
- Reading data from different file formats
- Loading data from a CSV file
- Loading data from a JSON file
- Conclusion
- FAQs
Importing the necessary libraries and modules
When working with Python, it is crucial to import the necessary libraries and modules to leverage their functionality and streamline your workflow. These libraries and modules provide a wide range of tools and functions that can greatly enhance the capabilities of your Python scripts.
One commonly used library is Pandas, which is known for its powerful data manipulation and analysis capabilities. By importing Pandas, you can easily load, transform, and analyze large datasets, making it an essential tool for data-driven tasks.
Another important library is NumPy, which stands for Numerical Python. NumPy provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions. It is widely used in scientific and numerical computing, making it invaluable for tasks involving complex calculations.
For visualization purposes, the Matplotlib library is commonly used. Matplotlib enables you to create various types of plots and charts to visualize your data. Whether you need a scatter plot, line graph, or histogram, Matplotlib has got you covered.
When dealing with machine learning and artificial intelligence, the Scikit-learn library is a must-have. Scikit-learn offers a wide range of machine learning algorithms, along with various pre-processing and evaluation tools. It simplifies the process of building and training machine learning models.
In addition to these libraries, there are numerous other modules available for specific purposes. For example, the datetime module provides functions to work with dates and times, the os module allows you to interact with the operating system, and the re module is used for regular expressions.
Importing these libraries and modules is easy. Simply use the import keyword followed by the name of the library or module you want to import. For example, to import Pandas, you can write:
import pandas as pd
This imports the Pandas library and gives it the alias “pd” for convenience. Now you can access all the functionalities provided by Pandas using the “pd” prefix.
Reading data from different file formats
In Python, there are various ways to read and load data from different file formats. Whether you have data stored in a CSV file, a JSON file, or any other format, Python provides libraries and modules that make the process seamless and efficient.
One popular library for handling CSV files is the csv
module. With this module, you can easily read data from a CSV file and load it into Python for further processing. The csv
module provides functions such as reader()
, which returns a reader object for iterating over the lines in the CSV file. You can then access the data in each line using indexing or looping through the reader object.
If you’re working with JSON files, Python provides the json
module for reading and loading JSON data. With the json
module, you can use the load()
or loads()
functions to read a JSON file and convert it into a Python object. The load()
function is used for reading from a file, while the loads()
function is used for reading from a string. Once the JSON data is loaded into a Python object, you can access the data using standard Python syntax.
For more complex file formats such as Excel files, Python offers the pandas
library. The pandas
library provides powerful data manipulation and analysis tools, including the ability to read Excel files using the read_excel()
function. This function allows you to specify the sheet name, header row, and other parameters to customize how the data is read and loaded into a pandas
DataFrame.
Aside from these commonly used file formats, Python provides modules for reading data from SQL databases, HDF5 files, XML files, and more. These modules offer specific functions and methods tailored for each file format, making it easy to read and load data into Python for analysis and processing.
Overall, Python’s versatility and rich ecosystem of libraries make it a powerful tool for reading and loading data from various file formats. Whether you’re dealing with CSV, JSON, Excel, or any other format, you can leverage Python’s functionalities to efficiently handle the data and unlock its potential.
Loading data from a CSV file
One of the most common ways to load data into Python is from a CSV (Comma-Separated Values) file. CSV files are simple and widely used for storing tabular data. Python provides built-in libraries that make it easy to load data from CSV files. Here’s how you can do it:
1. Import the necessary libraries: Before loading data from a CSV file, you need to import the required libraries. The two main libraries you’ll need are `csv` and `pandas`:
python
import csv
import pandas as pd
2. Open the CSV file: Once the libraries are imported, you can open the CSV file using the `open()` function and specify the file path:
python
with open(‘data.csv’, ‘r’) as file:
# Rest of the code goes here
3. Create a CSV reader object: Next, you need to create a CSV reader object using the `csv` module. This object will allow you to read the CSV file and access its contents:
python
csv_reader = csv.reader(file)
4. Load data into a pandas DataFrame: After creating the CSV reader object, you can load the data into a pandas DataFrame for further analysis. Use the `pd.DataFrame()` function and pass the CSV reader object as the parameter:
python
data = pd.DataFrame(csv_reader)
5. Optional: Specify column names: By default, pandas will use the first row of the CSV file as the column names. If your CSV file doesn’t have column names or you want to specify custom column names, you can do so by providing a list of column names as the `columns` parameter:
python
data = pd.DataFrame(csv_reader, columns=[‘Column 1’, ‘Column 2’, ‘Column 3’])
Note: Make sure the number of column names matches the number of columns in your CSV file.
6. Close the CSV file: After loading the data, it’s always a good practice to close the CSV file using the `close()` method:
python
file.close()
That’s it! You’ve successfully loaded data from a CSV file into Python using the `csv` module and pandas. Now you can perform various data manipulations and analysis on the loaded data.
Remember to adjust the file path and column names as per your specific CSV file. With this method, you can easily load data from any CSV file into Python and unleash the power of data analysis and manipulation.
Loading data from a JSON file
JSON (JavaScript Object Notation) is a popular data interchange format that is widely used for storing and transferring structured data. Python provides built-in support for working with JSON data, making it easy to load JSON files into your Python program.
To load data from a JSON file in Python, you’ll need to follow a few simple steps:
- First, import the `json` module, which provides the necessary functions for working with JSON data.
- Next, open the JSON file using the `open()` function. Make sure to specify the file path and the appropriate file mode (e.g., “r” for reading).
- Once the file is opened, use the `json.load()` function to load the JSON data from the file. This function reads the file contents and converts it into Python objects, such as dictionaries and lists.
- Finally, you can access the data in your Python program by assigning the loaded JSON data to a variable.
Here’s an example that demonstrates how to load data from a JSON file:
import json
# Open the JSON file
with open('data.json', 'r') as file:
# Load the JSON data
data = json.load(file)
# Access the loaded data
print(data)
In this example, the `json.load()` function is called with the opened file as an argument. The loaded JSON data is then assigned to the `data` variable, which can be used to access and manipulate the data as needed. Finally, the data is printed to the console using the `print()` function.
It’s important to note that the file path provided to the `open()` function should be the relative or absolute path to the JSON file on your system. Make sure to replace “data.json” with the actual file name and path in your code.
Additionally, if the JSON file contains an array of objects, you can iterate over the loaded data using a `for` loop to access individual objects:
import json
# Open the JSON file
with open('data.json', 'r') as file:
# Load the JSON data
data = json.load(file)
# Iterate over the objects
for obj in data:
print(obj)
In this example, each object within the loaded JSON data is printed to the console using the `print()` function. You can replace the `print(obj)` statement with your own logic to process and analyze the data.
Overall, loading data from a JSON file in Python is a straightforward process thanks to the built-in JSON support. By following these steps, you can easily access and work with JSON data within your Python programs.
Conclusion
In conclusion, loading data into Python is an essential skill for anyone working with data analysis, machine learning, or data science. Whether you are working with small datasets or large-scale data, Python provides a wide array of tools and libraries to efficiently load and manipulate data.
Throughout this article, we discussed various methods to load data into Python, including using libraries like Pandas, NumPy, and CSV. We explored how to load data from different file formats such as CSV, JSON, and Excel, as well as connecting to databases and performing data retrieval.
Remember, understanding how to load data effectively is just the first step in your data analysis journey. It is equally important to clean, preprocess, and analyze the data to derive meaningful insights. Python offers a wealth of resources and libraries to further explore and manipulate data.
By mastering the art of loading data into Python, you will unlock the potential to uncover valuable insights and make data-driven decisions.
FAQs
1. How do I load data into Python?
To load data into Python, you can use various methods depending on the file type and data format. Some commonly used methods include using the built-in open()
function to read text files, using the pandas
library to read CSV or Excel files, and using external libraries like json
or pickle
to handle data in their respective formats. It is important to have a clear understanding of the file structure and data format in order to choose the appropriate method for loading data into Python.
2. Can I load data from a database into Python?
Yes, you can load data from a database into Python. There are several ways to accomplish this, depending on the type of database and the Python libraries available. For instance, you can use libraries such as psycopg2
for PostgreSQL, mysql-connector-python
for MySQL, or pymongo
for MongoDB to establish a connection to the database and retrieve data. You will need to provide the necessary credentials and queries to retrieve the desired data from the database.
3. How can I load large datasets efficiently?
Loading large datasets into Python requires efficient memory management and optimization techniques. One approach is to use libraries like dask
or modin
, which provide parallel and distributed computing capabilities to handle large datasets using multiple cores or distributed systems. Another technique is to load the data in chunks rather than all at once, using tools like pandas
with the chunksize
parameter or using generators to process data incrementally.
4. Are there any specific considerations for loading different file formats?
Yes, different file formats may require specific considerations when loading data into Python. For example, when loading CSV files, you need to specify the delimiter and handle any missing or inconsistent values. When loading JSON files, you may need to navigate through nested structures or select specific keys for data extraction. Additionally, file compression formats like gzip or zip may require additional steps to decompress the data before loading it into Python.
5. How do I handle encoding issues when loading data into Python?
Encoding issues can arise when loading data into Python, especially with text files that contain different character encodings. To handle this, you can specify the encoding parameter when opening the file using the open()
function, or use libraries like pandas
that automatically detect and handle different encodings. In some cases, you may need to manually convert the text encoding using functions like decode()
or encode()
to ensure proper handling of non-standard characters or multilingual data.