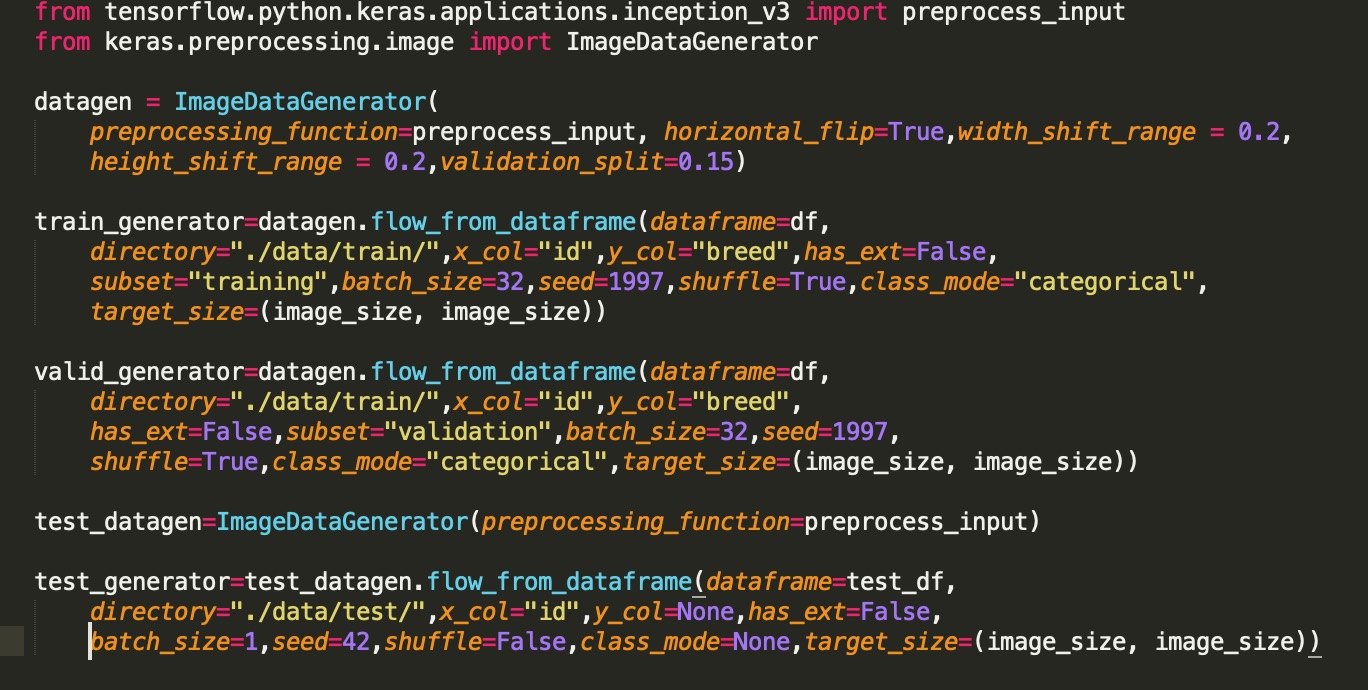
If you’re a Python developer, you know that data manipulation is a fundamental part of many projects. Whether you’re working with large datasets or simply need to rearrange the order of elements, shuffling data can be an essential task. Fortunately, Python provides a simple and efficient way to shuffle data using its built-in functions and libraries.
In this article, we’ll explore various methods to shuffle data in Python. We’ll learn how to randomly reorder elements in a list, shuffle rows in a DataFrame, and even shuffle the keys and values in a dictionary. Whether you’re a beginner or an experienced programmer, this guide will help you harness the power of Python to shuffle and randomize your data efficiently.
Inside This Article
- Overview
- Importing necessary libraries
- Reading data
- Shuffling data using random module
- Conclusion
- FAQs
Overview
In data analysis and machine learning tasks, shuffling data is a common technique used to ensure randomness and reduce bias in the training and testing datasets. Shuffling the data helps to eliminate any inherent order or patterns that may exist in the original dataset, allowing the model to learn from a more diverse and representative sample.
In Python, shuffling data can be easily achieved using the random
module. This module provides various functions and methods that allow us to generate random numbers, shuffle lists, and perform other randomization operations. By leveraging the random.shuffle()
function, we can effectively shuffle the data in Python.
In this article, we will explore the process of shuffling data in Python using the random.shuffle()
function. We will cover the steps involved in importing the necessary libraries, reading the data, and finally shuffling the data. By the end, you will have a solid understanding of how to shuffle data in Python, enabling you to enhance the robustness and reliability of your machine learning models.
Importing necessary libraries
When it comes to working with data in Python, it is essential to import the necessary libraries that provide the functionality needed for data manipulation and analysis. These libraries offer a wide range of functions and methods that can streamline the process and make it more efficient.
One of the most commonly used libraries in Python for data handling is the pandas library. Pandas is a powerful library that provides data structures and functions to easily manipulate and analyze data. To import pandas, you can use the following code:
python
import pandas as pd
In addition to pandas, another widely used library for scientific computing in Python is NumPy. NumPy provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. To import NumPy, you can use the following code:
python
import numpy as np
Furthermore, if your data requires visualization, you may want to import the matplotlib library. Matplotlib is a powerful plotting library that enables you to create various types of visualizations, such as line plots, scatter plots, histograms, and more. To import matplotlib, you can use the following code:
python
import matplotlib.pyplot as plt
Lastly, if you are planning to work with machine learning or data mining tasks, scikit-learn is a must-have library. Scikit-learn provides a wide range of machine learning algorithms and tools for tasks such as classification, regression, clustering, and dimensionality reduction. To import scikit-learn, you can use the following code:
python
import sklearn
By importing these libraries, you will have access to a plethora of functions and methods that can significantly simplify your data manipulation and analysis tasks in Python. Make sure to include these import statements at the beginning of your script or notebook to ensure smooth execution of your code.
Reading data
When it comes to working with data in Python, the first step is often to read the data from an external source. This could be a CSV file, a database, or any other data storage format. Python provides several libraries for reading data, such as `pandas`, `numpy`, and `csv`. In this section, we will focus on reading data using the `pandas` library, which is a powerful and versatile tool for data manipulation and analysis.
To read data using `pandas`, you first need to import the library. You can do this by adding the following line of code at the beginning of your script:
import pandas as pd
Once you have imported `pandas`, you can use the `read_csv()` function to read data from a CSV file. This function takes the path to the CSV file as an argument and returns a `DataFrame`, which is a two-dimensional table-like data structure. The `DataFrame` is a central data structure in `pandas`, and it allows you to perform various data operations easily.
Here is an example of how to read data from a CSV file:
data = pd.read_csv(‘data.csv’)
In the above code, `data.csv` is the path to the CSV file. Make sure to replace it with the correct file path in your code. Once the data is read, you can perform various operations on it, such as filtering, sorting, and aggregating.
It is important to note that `pandas` supports reading data from various file formats, not just CSV. You can also use the `read_excel()` function to read data from Excel files, or the `read_sql()` function to read data from a SQL database. `pandas` provides a wide range of functions and methods for reading and manipulating data, so make sure to explore the official documentation for more details.
Shuffling data using random module
Shuffling data is a common operation in data science and analysis. It randomly reorders the elements of a dataset, ensuring that each element has an equal chance of being in any position. In Python, the random module provides a convenient way to shuffle data efficiently.
To shuffle data using the random module, you first need to import it into your Python script. You can do this by using the import
statement:
import random
Once you have imported the random module, you can use the shuffle
function to shuffle the elements of a list or any iterable object. The shuffle
function modifies the original list in place, so there is no need to assign the shuffled list to a new variable.
data = [1, 2, 3, 4, 5]
random.shuffle(data)
print(data) # Output: [3, 1, 4, 5, 2]
The shuffle
function randomizes the order of the elements in the list. Each time you run the script, you may get a different ordering, as the shuffling is based on randomization.
It’s important to note that the shuffle
function works in place and modifies the original list. If you want to keep the original list intact and create a shuffled copy, you can use the sample
function instead.
data = [1, 2, 3, 4, 5]
shuffled_data = random.sample(data, len(data))
print(shuffled_data) # Output: [4, 2, 3, 5, 1]
The sample
function returns a new list containing a random selection of elements from the original list. By specifying the length of the original list as the second argument, you ensure that the shuffled list contains all the elements from the original list.
Shuffling data is not only useful for randomizing the order of elements but also for creating randomized datasets for training and testing machine learning models. By shuffling the data, you reduce the risk of any inherent ordering patterns affecting the model’s performance.
Overall, the random module in Python provides a simple and effective way to shuffle data. Whether you need to randomize the order of elements in a list or create randomized datasets, the random module has the necessary functions to make the process seamless and efficient.
Conclusion
Shuffling data in Python is a common task that can be easily accomplished using various methods and libraries. In this article, we explored two popular approaches for shuffling data: using the random module and the numpy library.
By leveraging the random module’s shuffle function, we can randomize the order of elements in a list or string. Alternatively, by employing the shuffle function from numpy, we can shuffle multidimensional arrays or specific axes within an array.
Whether you need to randomize the elements in a dataset, create randomness for simulation purposes, or improve the performance of machine learning algorithms, shuffling your data is essential. Understanding the different methods available in Python ensures that you have the flexibility to suit your specific needs.
Remember to consider the size of your data and the specific requirements of your application when selecting the appropriate shuffling method. Experimenting with different approaches will help you find the most efficient and effective strategy for shuffling data in Python.
FAQs
1. How do I shuffle data in Python?
To shuffle data in Python, you can use the `random` module’s `shuffle()` function. First, import the random module using `import random`. Then, create a list or an array with the data you want to shuffle. Finally, apply the `shuffle()` function to the list or array to shuffle the data in place.
2. Can I shuffle data in a specific order using Python?
Yes, you can shuffle data in a specific order using Python. The `random` module’s `shuffle()` function shuffles data randomly, but if you want to shuffle data based on a specific order, you can make use of the `random` module’s `sample()` function. The `sample()` function allows you to specify the order of the shuffled elements by providing the desired amount of elements from the original data.
3. How can I handle shuffling data with large datasets?
When dealing with large datasets, shuffling can become memory-intensive and time-consuming. To handle shuffling data with large datasets efficiently, you can use the `numpy` library’s `shuffle()` function. The `numpy` library effectively handles large datasets and provides optimized speed for shuffling.
4. Does shuffling data in Python affect the original dataset?
Yes, when you shuffle data in Python, it modifies the original dataset in place. This means that the order of the elements in the original data will be altered, and subsequent operations performed on the dataset will reflect the shuffled order.
5. Can I shuffle data in a specific range or subset?
Yes, you can shuffle data within a specific range or subset using Python. To achieve this, you can create a slice or subset of the original data and then apply the shuffling technique. By doing so, you can shuffle only a specific range or subset of the data while leaving the remaining elements untouched.