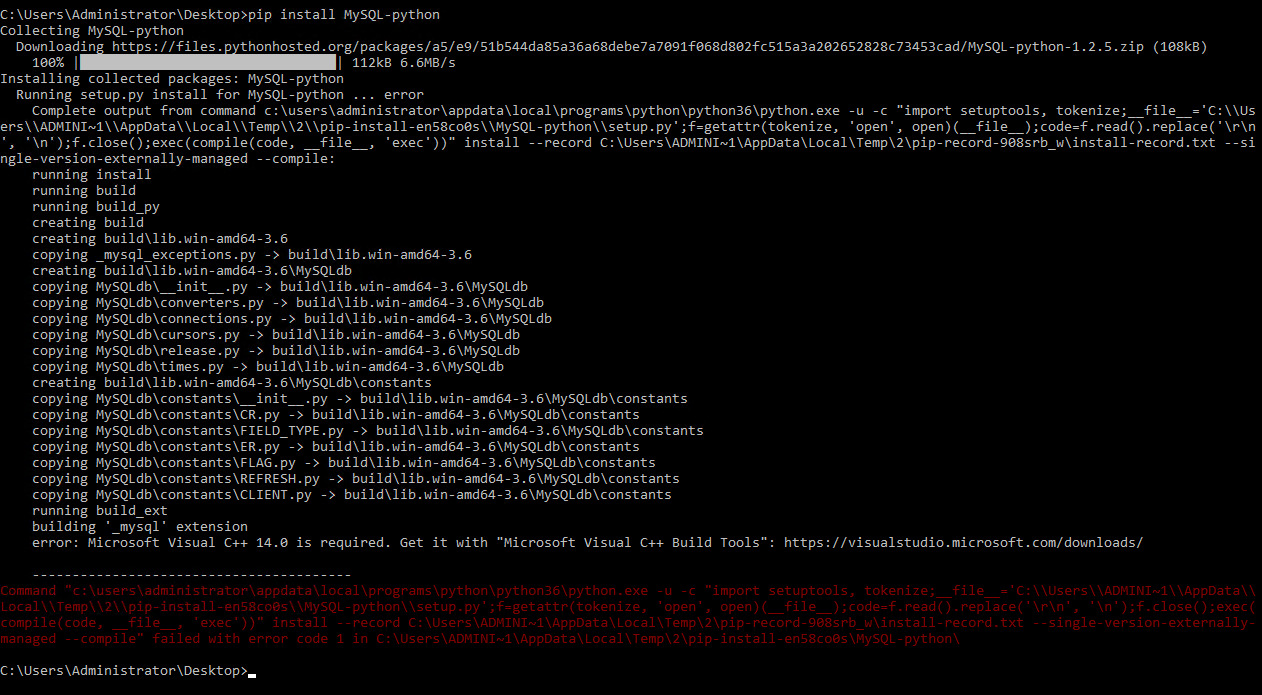
When it comes to working with databases using Python, one of the most popular choices is MySQL. MySQL is a widely used relational database management system that is known for its versatility and performance. In order to connect and interact with MySQL databases from Python, you will need to install the MySQL Connector library.
The MySQL Connector library is a Python driver that allows you to easily connect to and interact with MySQL databases. It provides a simple and efficient way to execute SQL statements, perform data manipulation, and retrieve query results. In this article, we will guide you step-by-step on how to install the MySQL Connector library in Python, so you can start working with MySQL databases in your Python projects.
Inside This Article
Prerequisites
Before installing MySQL Connector in Python, make sure you have the following prerequisites:
-
Python installed: Ensure that you have Python installed on your system. You can check if Python is installed by opening a terminal or command prompt and running the following command:
python --version
If Python is installed, you will see the version number displayed. If not, you will need to visit the official Python website (https://www.python.org) and download the appropriate version for your operating system.
-
MySQL Server installed: You will also need to have MySQL Server installed on your system. If you don’t have it installed yet, you can download it from the official MySQL website (https://www.mysql.com) and follow the installation instructions provided for your operating system.
-
Root access or appropriate privileges: To install MySQL Connector and connect to a MySQL database, you may need root access or appropriate privileges on your system. Make sure you have the necessary permissions to install software and configure database connections.
Once you have these prerequisites in place, you can proceed with the installation of MySQL Connector in Python.
Installing MySQL Connector
Before we dive into installing MySQL Connector, let’s take a moment to understand what it is. MySQL Connector is a Python module that allows us to connect and interact with a MySQL database using Python. It provides an interface for connecting to MySQL servers, executing SQL queries, and managing database operations, making it an essential component for working with MySQL databases in Python.
To install MySQL Connector, we need to follow these steps:
- Step 1: Install MySQL Server
- Step 2: Install pip
- Step 3: Install MySQL Connector
- Step 4: Verify the Installation
The first step is to ensure that MySQL Server is installed on your machine. If it is not already installed, you can download and install it from the official MySQL website (https://www.mysql.com/downloads/). Make sure to select the appropriate version for your operating system.
Pip is a popular package manager for Python, and it allows us to easily install and manage Python packages. To install pip, open a command prompt or terminal and run the following command:
python -m ensurepip --upgrade
This command will install or upgrade pip to the latest version.
With MySQL Server and pip installed, we can now proceed to install MySQL Connector. Open a command prompt or terminal and run the following command:
pip install mysql-connector-python
This will download and install the latest version of MySQL Connector from the Python Package Index (PyPI).
To verify that MySQL Connector is installed correctly, you can run the following Python code:
import mysql.connector
If there are no error messages, it means that MySQL Connector is successfully installed on your system.
Once you have followed these steps, you’re ready to start connecting to MySQL databases and performing various operations using Python and MySQL Connector.
Connecting to MySQL Database
Once you have completed the installation of the MySQL Connector for Python, you can now start connecting to your MySQL database. The Connector provides an easy-to-use interface for establishing a connection and executing SQL statements.
The first step is to import the necessary module by including the following line at the beginning of your Python script:
python
import mysql.connector
After importing the module, you need to establish a connection to the database. To do this, you will need to provide the hostname, username, password, and database name. The following code snippet demonstrates how to create a connection:
python
mydb = mysql.connector.connect(
host=”localhost,
user=”yourusername”,
password=”yourpassword”,
database=”yourdatabase”
)
Replace “localhost” with the hostname of your MySQL server. Enter your MySQL username, password, and the name of the database you want to connect to.
Once the connection is established, you can now execute SQL statements on the database. For example, if you want to retrieve data from a table named “users”, you can use the following code:
python
mycursor = mydb.cursor()
mycursor.execute(“SELECT * FROM users”)
result = mycursor.fetchall()
for row in result:
print(row)
The code above creates a cursor object, which is used to execute SQL statements. The execute()
method is used to execute the SQL query. The fetchall()
method retrieves all the rows returned by the query, and then we iterate over each row and print the result.
Remember to close the connection once you are done with your database operations. You can do this by invoking the close()
method on the connection object:
python
mydb.close()
By following these steps, you can successfully connect to your MySQL database and perform various operations like querying, inserting, updating, and deleting data.
Conclusion
Installing the MySQL Connector in Python opens up a world of possibilities for developers and data analysts. With this powerful tool, you can seamlessly connect your Python applications to MySQL databases, enabling you to retrieve, manipulate, and store data with ease.
In this article, we have explored the step-by-step process of installing the MySQL Connector in Python, emphasizing its compatibility with different operating systems. We discussed how to install it using pip, as well as through direct downloads from the MySQL website. We also touched upon some common troubleshooting tips.
By following the instructions provided, you can ensure a smooth installation experience and begin harnessing the power of MySQL within your Python projects. Whether you are building web applications, conducting data analysis, or working on any other project that requires database integration, the MySQL Connector in Python is an indispensable tool.
So, don’t hesitate to dive in and explore the endless possibilities that await you with the MySQL Connector in Python!
FAQs
Q: What is the purpose of installing MySQL Connector in Python?
A: The purpose of installing MySQL Connector in Python is to establish a connection between a Python program and a MySQL database. This allows the program to perform various database operations such as querying and modifying data.
Q: How do I install MySQL Connector in Python?
A: To install MySQL Connector in Python, you can use the pip package manager. Open your command line interface, navigate to the directory where Python is installed, and run the command “pip install mysql-connector-python”.
Q: Do I need to have MySQL installed on my computer to use MySQL Connector in Python?
A: Yes, you need to have MySQL installed on your computer in order to use MySQL Connector in Python. MySQL Connector is a library that enables communication between Python and an existing MySQL database. Without MySQL installed, you won’t be able to establish a connection.
Q: Can I use MySQL Connector in Python with other database management systems?
A: No, MySQL Connector is specifically designed for connecting Python programs to MySQL databases. If you need to connect to a different database management system, you will need to use a different connector or library specifically designed for that system.
Q: How can I establish a connection to a MySQL database using MySQL Connector in Python?
A: To establish a connection to a MySQL database using MySQL Connector in Python, you need to provide the necessary connection details such as the host, port, username, and password. Once the connection is established, you can use various functions and methods provided by MySQL Connector to interact with the database.