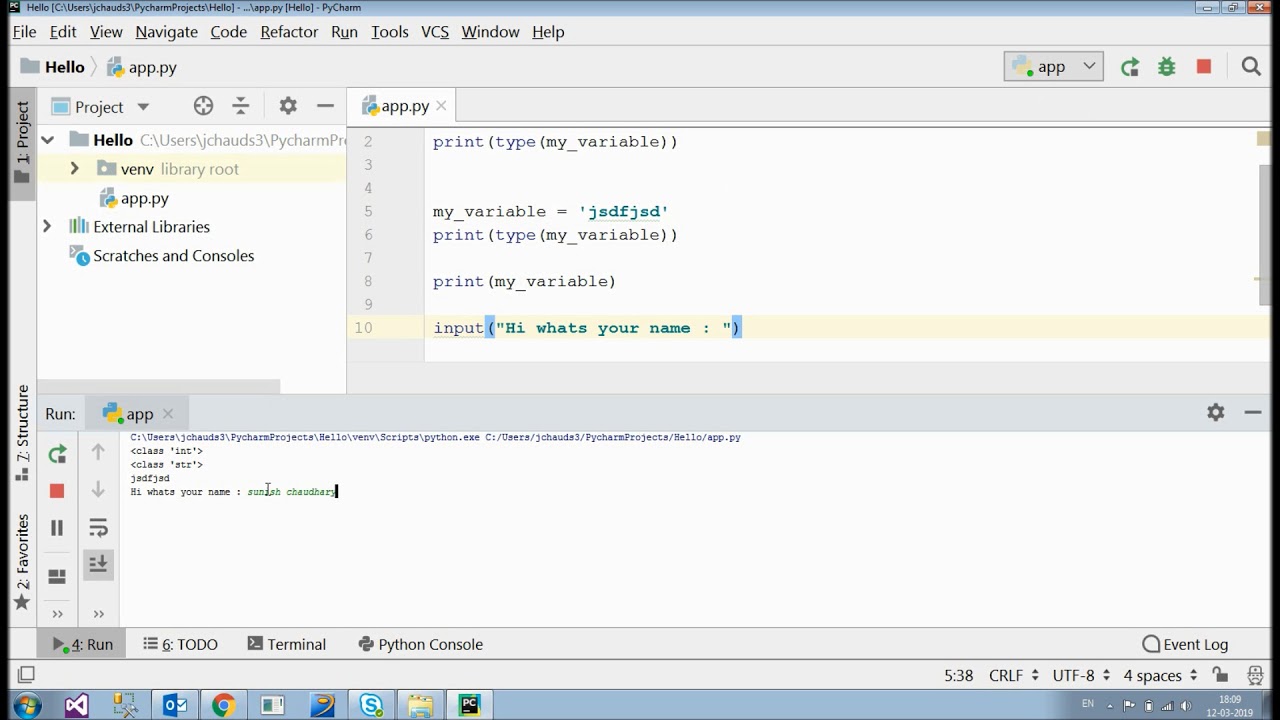
In Python, it is essential to understand the data type of a variable as it determines the operations that can be performed on it. Whether you are a beginner or an experienced programmer, knowing how to check the data type is a fundamental skill. Python provides a built-in function called type()
that allows you to inspect the data type of any variable or value. This simple and versatile function enables you to identify whether a variable is an integer, float, string, list, tuple, dictionary, or any other data type supported by Python. By understanding the data type, you can ensure that your code is robust, accurate, and effective. In this article, we will explore different methods that can be used to check the data type in Python, providing you with the knowledge and confidence to work with different types of data in your programming endeavors.
Inside This Article
- Getting the User Input
- Using the type() function
- Using the isinstance() function
- Checking if a Variable is of a Specific Type
- Conclusion
- FAQs
Getting the User Input
When writing a Python program, it’s common to need user input in order to perform certain tasks. Obtaining user input allows for more interactive and dynamic programs. Fortunately, Python provides a built-in function called input()
that makes it easy to get user input.
To get user input using the input()
function, you simply need to call the function and prompt the user with a message. The function will then wait for the user to enter their input. Once the user hits Enter, the function will return the input as a string.
For example:
python
name = input(“Please enter your name: “)
print(“Hello, ” + name + “! Nice to meet you.”)
This code prompts the user to enter their name and stores the input in the variable “name”. It then prints a friendly greeting using the entered name.
It’s worth noting that the input()
function always returns the user’s input as a string. If you need to convert the input to a different data type, such as an integer or a float, you can use type casting.
With the input()
function, you can easily create interactive programs that respond to user input and perform specific actions based on that input.
Using the type() function
When it comes to checking the data type of a variable in Python, one of the simplest and most straightforward methods is by using the type()
function. This function returns the type of an object or variable.
To use the type()
function, simply pass the variable as an argument within the parentheses. The function will then return the data type of the variable. For example:
x = 5
print(type(x))
The output will be:
<class 'int'>
In this example, the variable x
is assigned the value 5. By using the type()
function and printing the result, we see that the data type of x
is int
, which stands for integer.
The type()
function can be used with various data types, including strings, floats, booleans, lists, dictionaries, and more. It provides a quick and easy way to determine the data type of a variable in Python.
Using the isinstance() function
The isinstance()
function is a built-in function in Python that allows us to check the data type of a variable. It takes two parameters: the variable we want to check and the data type we want to compare it with.
The syntax for using the isinstance()
function is as follows:
isinstance(variable, data_type)
Here, the variable
is the variable we want to check, and the data_type
is the data type we want to compare it with. The isinstance()
function returns True
if the variable is of the specified data type, and False
otherwise.
Let’s take a look at an example:
name = "John"
age = 25
print(isinstance(name, str)) # Output: True
print(isinstance(age, int)) # Output: True
print(isinstance(name, int)) # Output: False
In the example above, we have a variable name
which is a string, and a variable age
which is an integer. We use the isinstance()
function to check their data types. The first two print()
statements return True
because the variables are of the specified data types. The third print()
statement returns False
because the name
variable is not an integer.
The isinstance()
function is particularly useful when you need to validate the data type of user inputs or when you want to perform specific actions based on the data type of a variable. It allows for more precise control and error handling in your Python programs.
By using the isinstance()
function, you can easily check the data type of a variable in Python and make decisions based on its type. It is a powerful tool that helps you ensure the correct data type is used in your programs, enhancing the reliability and efficiency of your code.
Checking if a Variable is of a Specific Type
When working with variables in Python, it is often necessary to check if a variable is of a specific data type. This can be particularly useful when you want to ensure the data being input or processed is in the expected format.
In Python, there are a couple of different ways you can check if a variable is of a specific type. Let’s explore some of these methods:
- Using the type() function: The type() function can be used to determine the data type of a variable. It returns a type object, which can be compared to the desired type to check if the variable is of that specific type. For example, if we want to check if a variable
num
is an integer, we can use the following code:python
num = 42
if type(num) == int:
print(“Variable is an integer.”)This will output “Variable is an integer” if the variable `num` is indeed an integer.
- Using the isinstance() function: The isinstance() function allows you to check if a variable is an instance of a specific class or its subclasses. This function takes two arguments – the variable you want to check and the data type you want to check against. For example, if we want to check if a variable
name
is a string, we can use the following code:python
name = “John”
if isinstance(name, str):
print(“Variable is a string.”)This will output “Variable is a string” if the variable `name` is indeed a string.
By using either the type() function or the isinstance() function, you can easily check if a variable is of a specific type in Python. This can help you ensure the data being processed is valid and in the expected format. Remember, using type() is useful for checking exact data types, while isinstance() is useful for checking against a class or its subclasses.
Keep in mind that it’s always important to validate user input and handle exceptions appropriately to prevent any unexpected errors in your code.
Conclusion
In conclusion, being able to check the data type in Python is an essential skill for any programmer. It allows you to write more robust and error-free code by ensuring that the input you receive matches your expectations. Whether you need to validate user input, handle different data formats, or debug your code, understanding how to check the data type will greatly aid your programming endeavors.
In this article, we discussed several ways to check the data type in Python, including using the type() function, isinstance() function, and the built-in methods provided by various data types. We also explored the importance of handling edge cases and understanding the nuances of different data types.
By grasping the concepts and techniques presented in this article, you are better equipped to write efficient and reliable Python code. The ability to check data types will not only make you a more proficient programmer but also enhance your problem-solving skills. Keep practicing and experimenting with different scenarios to deepen your understanding and mastery of data types in Python.
FAQs
Q: What is the data type in Python?
A: In Python, data type refers to the category or classification of data that determines the values it can take, the operations that can be performed on it, and the storage method. The common data types in Python include integers, floats, strings, lists, tuples, dictionaries, and more.
Q: How to check the data type in Python?
A: You can use the type() function in Python to check the data type of a variable or value. For example, type(42) will return <class 'int'>
indicating that it is an integer. Similarly, type(3.14) will return <class 'float'>
indicating a floating-point number.
Q: Can I change the data type of a variable in Python?
A: Yes, Python allows you to change the data type of a variable using explicit type conversion. For example, you can convert an integer to a float using the float() function, or convert a string to an integer using the int() function. However, keep in mind that the conversion might result in a loss of precision or data.
Q: How can I check if a variable is of a specific data type?
A: You can use the isinstance() function in Python to check if a variable is of a specific data type. This function takes two arguments – the variable to be checked and the data type to check against. It returns True if the variable is of the specified data type, and False otherwise. For example, isinstance(42, int) will return True.
Q: How important is understanding data types in Python?
A: Understanding data types in Python is crucial as it allows you to handle and manipulate data efficiently. Knowing the data type helps in performing appropriate operations, avoiding errors, and ensuring data integrity. It also enables you to write cleaner, more readable code and makes debugging easier.