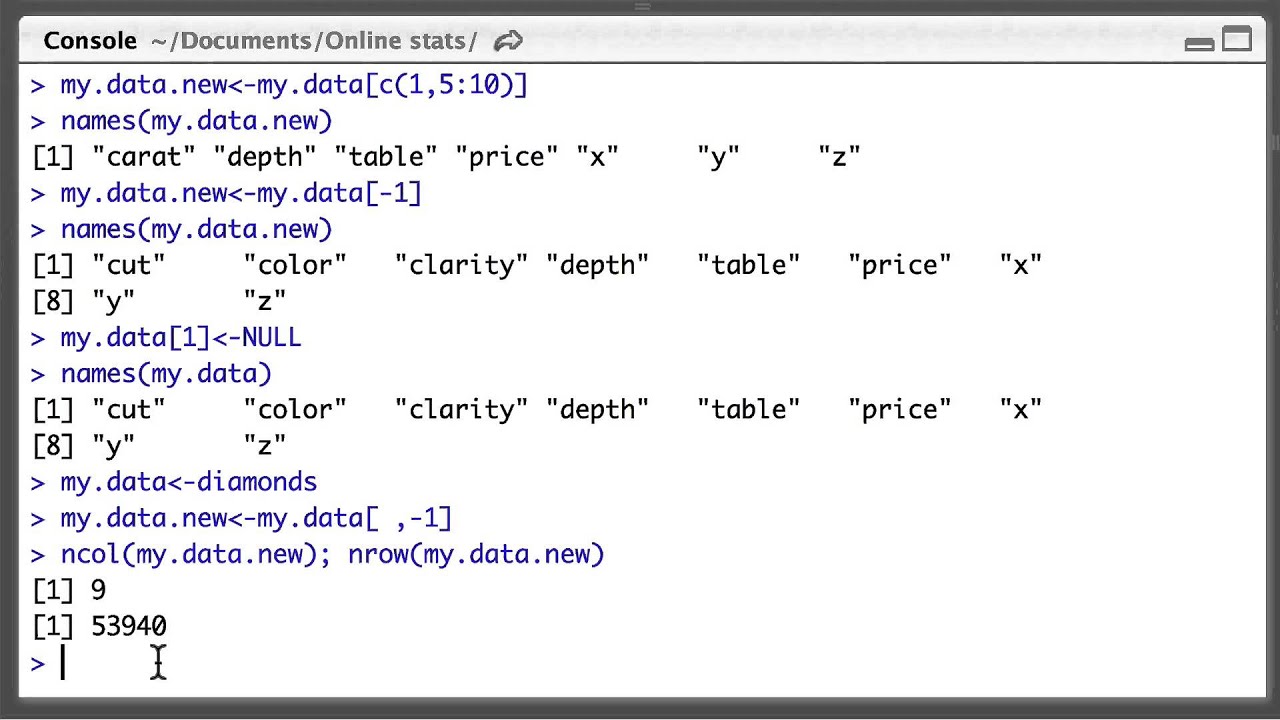
When it comes to data analysis and cleaning, R is a powerful tool that offers various functions and methods to remove data. Whether you want to get rid of duplicate entries, empty cells, or outliers, R provides a comprehensive set of options to effectively clean and manipulate your data. In this article, we will explore different techniques and approaches to remove unwanted data in R, allowing you to streamline your data analysis process and ensure accurate results. From using built-in functions like na.omit() and complete.cases() to applying filtering and subsetting methods, we will delve into the best practices and strategies for data removal in R. So, if you’re ready to optimize your data and eliminate any outliers or inconsistencies, let’s dive into the world of data cleansing in R!
Inside This Article
- Data Removal Methods in R
- Using the subset() Function
- Using the filter() Function from the dplyr Package
- Using the na.omit() Function
- Using the complete.cases() Function
- Conclusion
- FAQs
Data Removal Methods in R
When working with data in R, it is sometimes necessary to remove certain elements or observations from the dataset. This could be due to data inconsistencies, missing values, or the need to focus on a specific subset of the data. In this article, we will explore four popular methods for removing data in R.
1. Using the subset() Function
The subset() function in R allows you to create a new dataset by selecting specific observations based on certain conditions. By specifying the logical conditions within the subset() function, you can filter out unwanted data. For example, to remove all rows where the age column is less than 18 years, you can use the following code:
subset(data, age >= 18)
2. Using the filter() Function from the dplyr Package
The dplyr package in R provides powerful tools for data manipulation. The filter() function is one such tool that enables you to remove rows based on specific conditions. This function follows a similar syntax to the subset() function but offers more flexibility and readability. Here’s an example of how to remove all rows where the income column is missing:
library(dplyr) filter(data, !is.na(income))
3. Using the na.omit() Function
Sometimes, your dataset may contain missing values, denoted as NA. The na.omit() function in R automatically removes any rows that contain missing values from the dataset. This method is particularly useful when you need to deal with missing data before performing further analysis. Simply apply the na.omit() function to your dataset as shown below:
new_data <- na.omit(data)
4. Using the complete.cases() Function
The complete.cases() function in R allows you to identify and remove rows with missing values across multiple columns. It returns a logical vector where TRUE represents complete cases (no missing values) and FALSE represents incomplete cases. By subsetting the dataset based on this logical vector, you can remove the rows with missing data. Here's an example:
complete_cases <- complete.cases(data) new_data <- data[complete_cases,]
These are just a few methods for removing data in R. The choice of method depends on the specific requirements of your analysis and the nature of the dataset. Experiment with these functions and explore other techniques to efficiently manage and manipulate your data in R. Happy coding!
Using the subset() Function
In R, the subset()
function is a useful tool for removing data based on specific conditions. This function allows you to select a subset of a data frame based on chosen criteria, and exclude the unwanted rows or columns.
The general syntax for using the subset()
function is as follows:
subset(x, condition, select)
Here, x
refers to the data frame you want to subset, condition
represents the logical expression that determines which rows to include, and select
specifies the columns you want to retain.
Let's look at an example to better understand how the subset()
function works. Suppose we have a data frame called "students" that contains information about students, including their names, ages, and grades.
We can use the subset()
function to remove all the rows where the students' grades are below a certain threshold. Here's how:
threshold <- 80
filtered_students <- subset(students, grade >= threshold)
In the above code, we set the threshold to 80 and use the subset()
function to create a new data frame called "filtered_students" that only includes the rows where the grade is greater than or equal to the threshold.
Using the subset()
function allows you to easily filter out unwanted data based on specific conditions, making it a powerful tool for data removal in R.
Using the filter() Function from the dplyr Package
The filter() function from the dplyr package in R is a powerful and efficient way to remove specific data from your dataset. This function allows you to apply one or more conditions to filter out rows based on your specified criteria.
To use the filter() function, you'll first need to install and load the dplyr package in your R environment. You can do this by running the following commands:
install.packages("dplyr")
library(dplyr)
Once you have the package loaded, you can start utilizing the filter() function to remove data. The basic syntax of the filter() function is:
filtered_data <- filter(dataset, condition)
Here, dataset
is the name of your dataset, and condition
is the logical expression that specifies the criteria for data removal.
Let's say you have a dataset named my_data
with columns like 'Name', 'Age', and 'Salary'. If you want to remove all the rows where the age is greater than 50, you can use the filter() function as follows:
filtered_data <- filter(my_data, Age <= 50)
This will create a new dataset called filtered_data
that only contains rows where the age is less than or equal to 50. The original dataset my_data
remains unchanged.
You can also apply multiple conditions using the filter() function. For example, if you want to remove all the rows where the age is greater than 50 and the salary is less than 50000, you can use the following code:
filtered_data <- filter(my_data, Age <= 50 & Salary >= 50000)
This will create a new dataset called filtered_data
that satisfies both conditions: age less than or equal to 50 and salary greater than or equal to 50000.
The filter() function offers a flexible and convenient way to remove data based on specific conditions in R. It allows you to refine your datasets and focus on the relevant information for your analysis. Experiment with different conditions and explore the power of the filter() function to effectively remove data from your dataset.
Using the na.omit() Function
Another method to remove missing or NA values from a dataset in R is by using the na.omit() function. This function is particularly useful when working with large datasets that contain missing values.
The na.omit() function works by removing any rows that have missing values in any of its columns. It returns a new data frame with the incomplete cases omitted.
To use the na.omit() function, you simply need to pass your dataset as an argument:
new_data <- na.omit(data)
In the code snippet above, the na.omit() function will remove any rows with missing values from the 'data' dataset and assign the result to the 'new_data' data frame.
It's important to note that the na.omit() function effectively removes all rows that contain NA values, which may result in a smaller dataset. Therefore, it's crucial to consider the implications of removing these missing values on your analysis.
Once you have used the na.omit() function to remove the missing values, you can proceed with your data analysis or further cleaning steps. This function is a handy option for quickly handling missing values without the need for manual deletion or imputation.
Using the complete.cases() Function
The complete.cases()
function is another powerful method to remove data in R. It allows you to filter out rows containing missing values in any of the variables in your dataset.
To use the complete.cases()
function, you simply pass your dataset as an argument. It will return a logical vector indicating whether each row is complete or not. By subsetting your dataset using this logical vector, you can remove the rows with missing values.
Here's an example to demonstrate how to use the complete.cases()
function:
data <- data[complete.cases(data), ]
In the example above, the complete.cases()
function filters out the rows with missing values from the data
dataset. By assigning the filtered dataset back to data
, you effectively remove the rows with missing values.
This method is particularly useful when you have missing values scattered across different variables in your dataset. It ensures that only complete cases are retained, giving you a clean and consistent dataset to work with.
Conclusion
In conclusion, understanding how to remove data in R is an essential skill for anyone working with data analysis or statistical modeling. R provides powerful functions and techniques to manipulate and filter data, allowing you to extract the relevant information and improve the quality of your analysis.
Whether you need to remove specific observations, delete variables, or eliminate duplicates, the various methods discussed in this article offer flexible solutions to meet your data cleansing needs. By utilizing these techniques, you can ensure that your data is accurate, consistent, and free from errors or outliers, leading to more robust and reliable insights.
Remember to document your data removal processes and keep a backup of your original dataset before applying any modifications. This will allow you to track your steps and revert changes if needed, maintaining data integrity throughout your analysis.
With the knowledge gained from this article, you can confidently tackle data cleaning tasks in R, enhancing the quality and reliability of your analyses. So, dive in and start uncovering valuable insights from your data!
FAQs
1. Why is it important to remove data in R?
Removing data in R is important to maintain data integrity and ensure accurate analysis. By removing irrelevant or erroneous data, you can prevent it from skewing your results or leading to incorrect conclusions.
2. What are some common methods to remove data in R?
There are several common methods to remove data in R, including using subsetting techniques, filtering functions, and removing missing values. You can subset data by specifying specific rows or columns to keep and removing the rest. Filtering functions like filter()
or subset()
can be used to remove data based on specific conditions. Additionally, you can use functions like na.omit()
to remove rows with missing values.
3. How do I remove specific rows or columns in R?
To remove specific rows or columns in R, you can use the subsetting technique. You can use the square bracket notation to specify the rows or columns you want to keep. For example, to remove the first and third column from a data frame "df", you can use the following code:
df <- df[, -c(1, 3)]
4. What is the purpose of filtering functions in R?
Filtering functions in R, such as filter()
or subset()
, allow you to remove data based on specific conditions. These functions provide a convenient way to subset your data based on logical expressions. For example, you can filter out rows where a certain variable is above a certain threshold or exclude rows that meet certain criteria.
5. How do I remove missing values in R?
To remove missing values in R, you can use the na.omit()
function. This function removes any rows that contain missing values from your data frame. For example, if you have a data frame called "df" and you want to remove rows with missing values, you can use the following code:
df <- na.omit(df)