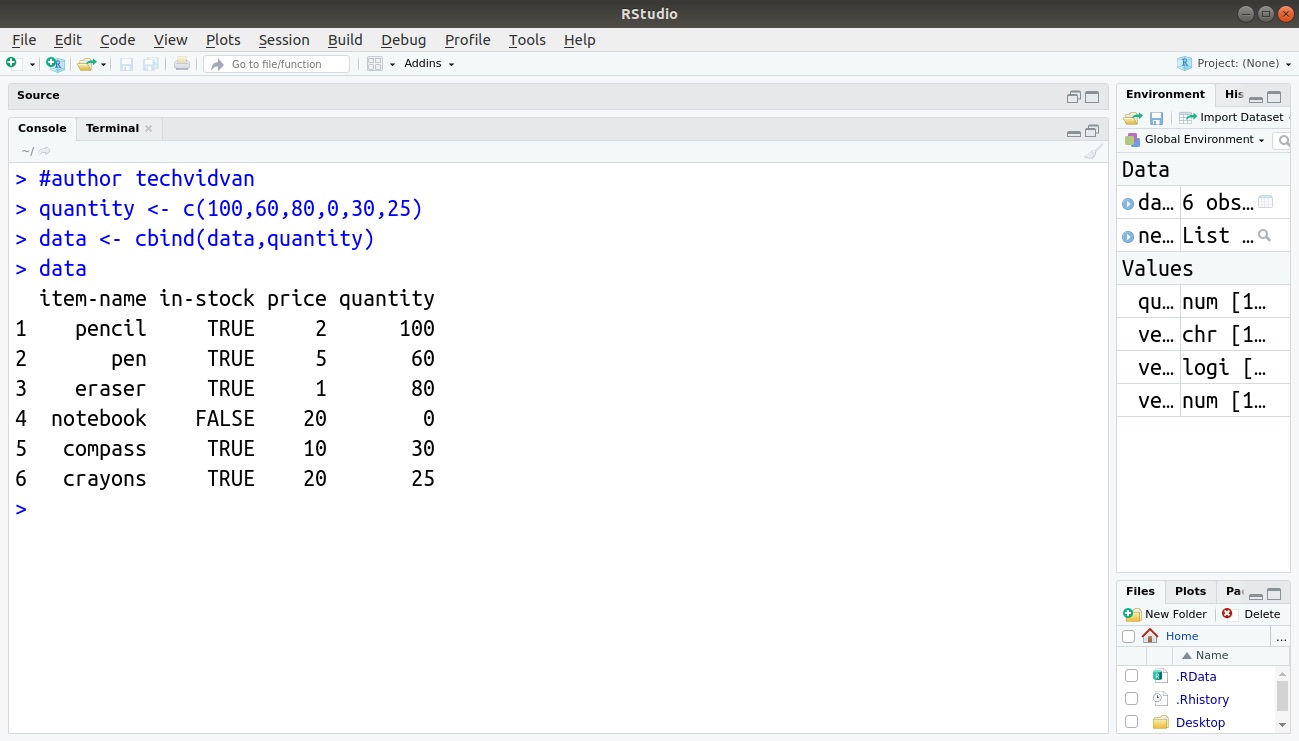
Are you looking to add a new column to your R data frame? Knowing how to add a column in R is a valuable skill for data analysts and programmers alike. Whether you need to add a calculated column, merge data from different sources, or simply insert a new column of data, the process can be accomplished with a few simple steps. In this article, we will guide you through the process of adding a column to an R data frame. We will cover the different methods available, including using base R functions, the dplyr package, and other useful techniques. By the end of this article, you will have the knowledge and confidence to add columns to your R data frame with ease. So let’s get started and level up your R programming skills!
Inside This Article
- Overview
- Method 1: Using the cbind() Function
- Method 2: Using the attach() Function
- Method 3: Using the ‘$’ Operator
- Method 4: Using the subset() Function
- Conclusion
- FAQs
Overview
Adding a column to an R data frame is a common task when working with datasets. A data frame is a two-dimensional tabular data structure in R, where each column can have different data types. Adding a new column to a data frame allows you to include additional information or perform calculations based on existing data.
There are several methods that you can use to add a column to an R data frame. In this article, we will explore four different approaches: using the cbind() function, using the attach() function, using the ‘$’ operator, and using the subset() function. Each method has its own advantages and may be more suitable for different situations.
Whether you need to add a calculated column, merge multiple data frames, or simply include additional information, understanding how to add a column will be beneficial in your data analysis tasks. Let’s dive into the various methods and see how you can easily add a column to an R data frame.
Method 1: Using the cbind() Function
One way to add a column to a data frame in R is by using the cbind() function. The cbind() function stands for “column bind” and is used to combine vectors into a matrix or data frame.
To add a column using the cbind() function, you need to create a new vector with the values you want to add as a column. Then, you can use the cbind() function to bind the new vector to the existing data frame.
Here’s an example to illustrate how to use the cbind() function:
R
# Create a data frame
df <- data.frame(A = c(1, 2, 3),
B = c(4, 5, 6))
# Create a new vector
new_column <- c(7, 8, 9)
# Add the new column to the data frame using cbind()
df <- cbind(df, new_column)
# View the updated data frame
print(df)
In this example, we first create a data frame called “df” with two columns, A and B. Next, we create a new vector called “new_column” with the values 7, 8, and 9. Finally, we use the cbind() function to add the new column to the data frame, and then we print the updated data frame.
By using the cbind() function, you can easily add a column to a data frame in R. This method is straightforward and provides a quick way to expand your data frame with new information.
Method 2: Using the attach() Function
In addition to the cbind() function, another way to add a column to an R data frame is by using the attach() function. This function allows you to temporarily attach the data frame to your R environment, making its columns directly accessible.
To add a column using the attach() function, follow these steps:
- Step 1: Start by attaching the data frame using the attach() function. For example, if your data frame is named “df”, you would use the code:
attach(df)
. - Step 2: Once the data frame is attached, you can now refer to its columns directly without specifying the data frame name. For instance, if you want to add a new column named “new_column” with values 1, 2, 3, 4, and 5, you can simply use the code:
new_column <- c(1, 2, 3, 4, 5)
. - Step 3: After adding the column, you can detach the data frame using the detach() function to avoid any potential conflicts or confusion. The code for detaching the data frame is:
detach(df)
.
It's important to note that the attach() function should be used with caution, as it can lead to potential naming conflicts if multiple data frames have columns with the same name. It is generally recommended to use attach() sparingly and detach the data frame once you are done working with it.
Using the attach() function can provide a convenient way to add a column to an R data frame without explicitly referencing the data frame name. However, it is important to be mindful of potential naming conflicts and to detach the data frame once you are finished. By following these steps, you can easily add a new column to your R data frame using the attach() function.
Method 3: Using the '
Another way to add a column to an R data frame is by using the '$' operator. This method is slightly different from the previous ones, as it allows you to directly access and modify a specific column in the data frame.
The syntax for using the '$' operator is as follows:
dataframe$column_name <- new_column_values
Here, "dataframe" refers to the name of your data frame, "column_name" is the name of the column you want to add or modify, and "new_column_values" are the values that you want to assign to the column.
Let's say you have a data frame called "df", and you want to add a new column called "age" with the corresponding age values. You can accomplish this using the '$' operator as shown below:
df$age <- c(25, 30, 35, 28, 32)
This will add a new column named "age" to your data frame "df" and assign the provided age values to it.
It's important to note that when using the '$' operator, the column name must be specified as a character string. If the column name contains spaces or special characters, you need to enclose it in backticks (`).
For example, if you want to add a column named "postal code" to your data frame using the '$' operator, you should use the following syntax:
dataframe$`postal code` <- new_column_values
By using the '$' operator, you have the flexibility to directly access and modify specific columns in your data frame, making it a convenient method for adding new columns.
Method 4: Using the subset() Function
Another way to add a column to a data frame in R is by using the subset() function. The subset() function allows you to select specific rows from a data frame based on certain conditions.
To add a column using the subset() function, you need to specify the data frame, the subset condition, and the new column you want to create. Here's an example:
new_column <- subset(dataframe, subset_condition)
In this example, dataframe
represents the name of your existing data frame, subset_condition
is the condition you want to use to select the rows, and new_column
is the name of the column you want to add.
The subset condition can be any valid R expression. For example, if you want to select rows where the values in an existing column are greater than a certain threshold, you can use the following condition:
new_column <- subset(dataframe, existing_column > threshold)
This will create a new column named new_column
that will contain the values from the existing_column
that are greater than the specified threshold.
It's important to note that the subset() function returns a new data frame with the selected rows and columns. If you want to update the original data frame with the new column, you can assign the result back to the original data frame as follows:
dataframe <- subset(dataframe, subset_condition)
This will replace the original data frame with the subsetted data frame containing the new column.
Using the subset() function to add a column offers flexibility in selecting specific rows based on your desired conditions. It can effectively extend the functionality of your data frame and provide you with tailored data analysis options.
Operator
Another way to add a column to an R data frame is by using the '$' operator. This method is slightly different from the previous ones, as it allows you to directly access and modify a specific column in the data frame.
The syntax for using the '$' operator is as follows:
dataframe$column_name <- new_column_values
Here, "dataframe" refers to the name of your data frame, "column_name" is the name of the column you want to add or modify, and "new_column_values" are the values that you want to assign to the column.
Let's say you have a data frame called "df", and you want to add a new column called "age" with the corresponding age values. You can accomplish this using the '$' operator as shown below:
df$age <- c(25, 30, 35, 28, 32)
This will add a new column named "age" to your data frame "df" and assign the provided age values to it.
It's important to note that when using the '$' operator, the column name must be specified as a character string. If the column name contains spaces or special characters, you need to enclose it in backticks (`).
For example, if you want to add a column named "postal code" to your data frame using the '$' operator, you should use the following syntax:
dataframe$`postal code` <- new_column_values
By using the '$' operator, you have the flexibility to directly access and modify specific columns in your data frame, making it a convenient method for adding new columns.
Method 4: Using the subset() Function
Another way to add a column to a data frame in R is by using the subset() function. The subset() function allows you to select specific rows from a data frame based on certain conditions.
To add a column using the subset() function, you need to specify the data frame, the subset condition, and the new column you want to create. Here's an example:
new_column <- subset(dataframe, subset_condition)
In this example, dataframe
represents the name of your existing data frame, subset_condition
is the condition you want to use to select the rows, and new_column
is the name of the column you want to add.
The subset condition can be any valid R expression. For example, if you want to select rows where the values in an existing column are greater than a certain threshold, you can use the following condition:
new_column <- subset(dataframe, existing_column > threshold)
This will create a new column named new_column
that will contain the values from the existing_column
that are greater than the specified threshold.
It's important to note that the subset() function returns a new data frame with the selected rows and columns. If you want to update the original data frame with the new column, you can assign the result back to the original data frame as follows:
dataframe <- subset(dataframe, subset_condition)
This will replace the original data frame with the subsetted data frame containing the new column.
Using the subset() function to add a column offers flexibility in selecting specific rows based on your desired conditions. It can effectively extend the functionality of your data frame and provide you with tailored data analysis options.
Conclusion
Adding a column to a data frame in R is a fundamental operation that allows us to manipulate and analyze our data effectively. Whether it's for creating new variables, combining existing ones, or performing calculations, the ability to add columns gives us more flexibility and control in our data analysis process.
By using the base R functions or specialized packages like dplyr, we can easily add columns to our data frames based on specific conditions, mathematical operations, or applied functions. Additionally, we can also modify existing columns or create new columns using logical statements or vectorized operations.
Understanding how to add columns in R data frames opens up a plethora of possibilities for exploring, transforming, and visualizing data. With a solid grasp of this concept, we can confidently handle diverse data sets and leverage R's vast array of data manipulation and analysis capabilities to derive valuable insights.
FAQs
1. Why would I need to add a column in an R data frame?
Adding a column to an R data frame allows you to include new variables or modify existing ones within your dataset. This can be useful for various purposes, such as performing calculations, creating derived columns, or organizing the data in a way that better suits your analysis.
2. How can I add a column to an R data frame?
To add a column to an R data frame, you can use the assignment operator (<-
) to assign a new vector to the desired column name. For example, if you have a data frame named my_df
and you want to add a column named new_col
, you can use the following code:
my_df$new_col <- my_vector
3. Can I add a column with default values to an R data frame?
Yes, you can add a column with default values to an R data frame. To do this, you can assign a vector of the desired length, filled with the desired default value, to the new column. For example, if you want to add a column named default_col
with a default value of 0 to a data frame named my_df
, you can use the following code:
my_df$default_col <- rep(0, nrow(my_df))
4. Is it possible to add a column based on conditions in an R data frame?
Yes, it is possible to add a column based on conditions in an R data frame. You can use the ifelse()
function or other conditional statements to apply specific values or calculations to the new column based on certain conditions in the data frame. This can be helpful for data transformations or creating categorical variables based on certain criteria.
5. Can I add a column to an R data frame using existing columns?
Yes, you can add a column to an R data frame using existing columns. You can perform calculations or create new variables based on the values of one or more existing columns and assign the result to the new column. This allows you to derive new information from your dataset or perform data manipulations based on the existing data.