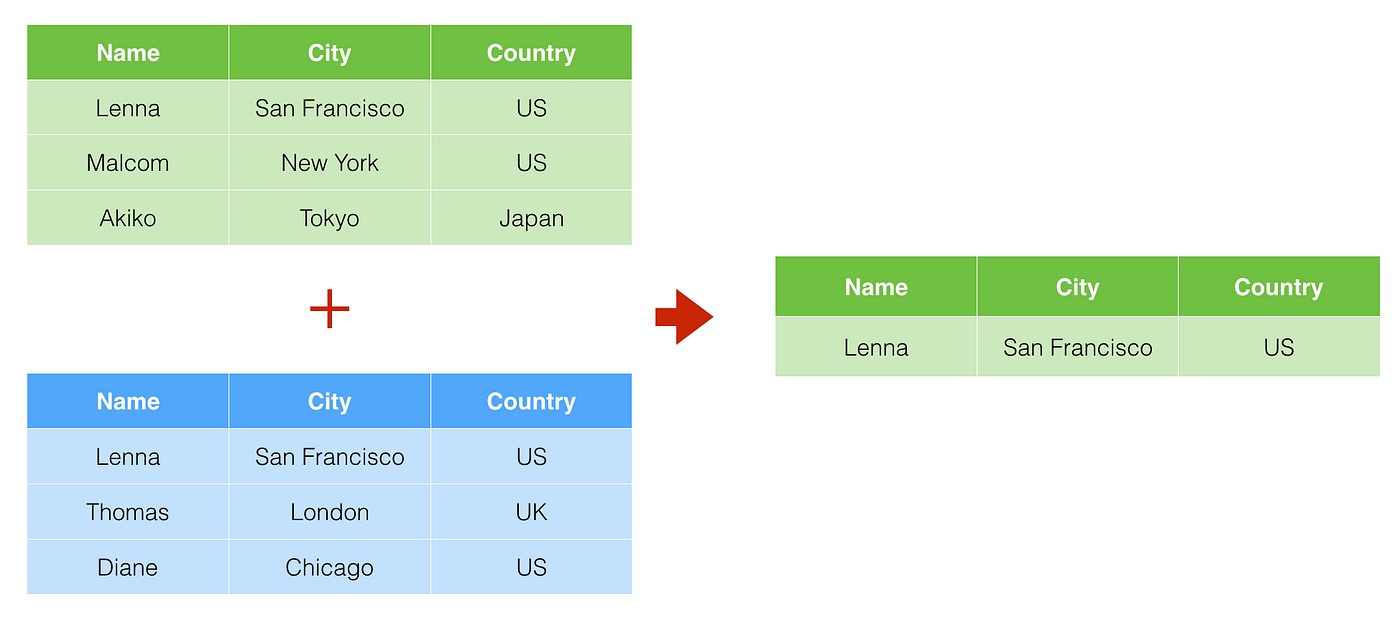
Combining two data frames is a common operation in data analysis and manipulation using the R programming language. Whether you need to merge data from different sources, add or update columns, or perform complex joins, the ability to combine data frames efficiently is essential for working with large and diverse datasets.
In this comprehensive guide, we will explore various methods and techniques to successfully combine two data frames in R. We will cover topics such as merging data frames based on keys or common columns, appending rows, and performing inner, left, right, and outer joins. By the end of this article, you will have a solid understanding of how to merge and combine data frames effectively, enabling you to derive valuable insights and make data-driven decisions.
Inside This Article
- Method 1: Using the merge() Function
- Method 2: Using the bind_rows() Function from dplyr Package
- Method 3: Using the cbind() or bind_cols() Function
- Method 4: Using the join() Function from the plyr Package
- Conclusion
- FAQs
Method 1: Using the merge() Function
The merge() function is a powerful and widely used function in R for combining two data frames based on common columns or key variables. It allows you to merge the data frames horizontally, joining them based on matching values in the specified columns. The merge() function provides flexibility in handling different types of joins such as inner join, left join, right join, and full join.
To use the merge() function, you need to specify the two data frames you want to merge, as well as the common column(s) or key variable(s) you want to use for the merge. Here’s the basic syntax:
merged_df <- merge(df1, df2, by = "common_column")
Let's break down the syntax:
df1
anddf2
: The two data frames you want to merge.by = "common_column"
: The common column(s) or key variable(s) on which the merge should be performed.
The merge() function performs an inner join by default, which means it returns only the rows where there is a match in both data frames based on the common column(s). If you want to perform a different type of join, you can specify it using the all.x
, all.y
, or all
arguments.
Here's an example of using the merge() function to combine two data frames:
df1 <- data.frame(ID = c(1, 2, 3, 4), Name = c("John", "Amy", "David", "Sara"))
df2 <- data.frame(ID = c(1, 2, 3, 5), Age = c(25, 30, 35, 40))
merged_df <- merge(df1, df2, by = "ID")
The resulting merged_df data frame will contain all the columns from both df1 and df2, and only the rows where the ID column has matching values in both data frames.
With the merge() function, you can handle different scenarios where you need to combine two data frames based on their common columns or key variables. It provides a flexible and efficient way to perform joins in R, making it a valuable tool in data manipulation and analysis.
Method 2: Using the bind_rows() Function from dplyr Package
In addition to the merge() function, another handy way to combine two data frames in R is by using the bind_rows() function from the dplyr package. The dplyr package is a powerful tool for data manipulation and provides a variety of functions that make data cleaning, transformation, and analysis more efficient.
The bind_rows() function allows you to vertically stack data frames on top of each other, assuming they have the same columns. It is similar to the rbind() function, but with added flexibility and improved performance.
Let's take a look at how you can use the bind_rows() function to combine two data frames:
- Step 1: Load the dplyr package by using the library() function:
- Step 2: Create two data frames that you want to combine. Let's call them df1 and df2:
- Step 3: Use the bind_rows() function to combine the two data frames:
- Step 4: The resulting combined_df data frame will contain the rows from both df1 and df2:
library(dplyr)
# Create df1
df1 <- data.frame(ID = c(1, 2, 3),
Name = c("John", "Alice", "David"),
Age = c(25, 30, 35))
# Create df2
df2 <- data.frame(ID = c(4, 5, 6),
Name = c("Emma", "Michael", "Sophia"),
Age = c(20, 27, 32))
# Combine df1 and df2
combined_df <- bind_rows(df1, df2)
combined_df
ID Name Age
1 1 John 25
2 2 Alice 30
3 3 David 35
4 4 Emma 20
5 5 Michael 27
6 6 Sophia 32
By default, the bind_rows() function ignores the row names from individual data frames and generates a new set of row names for the combined data frame. However, you can use the rownames_to_column() function from the tibble package to add the row names as a separate column if needed.
Overall, the bind_rows() function from the dplyr package provides a convenient and efficient method to combine two data frames vertically. It is particularly useful when you have data frames with the same column structure and want to stack them together for further analysis or visualization.
Method 3: Using the cbind() or bind_cols() Function
In addition to the merge() and bind_rows() functions, R also provides the cbind() and bind_cols() functions to combine two data frames horizontally. These functions work by binding the columns of the two data frames together.
To use the cbind() function, you simply pass in the two data frames as arguments. The function will match the rows based on their indices and concatenate the columns together. Here's an example:
R
# Create two sample data frames
df1 <- data.frame(ID = 1:3, Name = c("John", "Emily", "Michael"))
df2 <- data.frame(ID = 4:6, Age = c(25, 30, 35))
# Combine the data frames using cbind()
combined <- cbind(df1, df2)
# Print the combined data frame
print(combined)
The output will be:
ID Name ID.1 Age
1 1 John 4 25
2 2 Emily 5 30
3 3 Michael 6 35
You can see that the columns from both data frames have been combined horizontally, with the matching row indices. The resulting data frame, combined, now has all the columns from df1 and df2.
Similarly, the bind_cols() function from the dplyr package is another convenient way to combine data frames horizontally. This function works similarly to cbind(), but provides some additional functionalities. Here's an example:
R
# Load the dplyr package
library(dplyr)
# Combine the data frames using bind_cols()
combined <- bind_cols(df1, df2)
# Print the combined data frame
print(combined)
The output will be:
ID Name ID Age
1 1 John 4 25
2 2 Emily 5 30
3 3 Michael 6 35
As you can see, the result is the same as the cbind() function. However, bind_cols() allows you to combine more than two data frames in a single operation, making it useful when you have multiple data frames to merge.
Both cbind() and bind_cols() functions are efficient ways to horizontally combine data frames in R. They offer flexibility and ease of use when it comes to merging columns from multiple data frames.
Method 4: Using the join() Function from the plyr Package
If you're working with large datasets in R and need a more efficient way to combine two data frames, the plyr package provides a powerful function called join(). This function allows you to perform different types of joins, such as inner, left, right, and outer joins, with ease.
To use the join() function, you first need to install and load the plyr package. You can do this by running the following code:
R
install.packages("plyr")
library(plyr)
Once the package is loaded, you can use the join() function to merge two data frames based on a common column or columns. The syntax for the join() function looks like this:
R
join(x, y, by = NULL, type = "inner")
Here, x
and y
are the data frames you want to join, by
specifies the column(s) to join on, and type
determines the type of join you want to perform.
For example, let's say you have two data frames: df1
and df2
. You want to join them based on the "id" column. Here's how you can do it:
R
joined_df <- join(df1, df2, by = "id")
This will perform an inner join by default, meaning only the rows with matching values in the "id" column will be included in the resulting data frame.
If you want to perform a different type of join, you can specify it using the type
parameter. For example, if you want to perform a left join, you can do it like this:
R
left_join_df <- join(df1, df2, by = "id", type = "left")
Similarly, you can perform right and outer joins by setting the type
parameter to "right" and "outer", respectively.
The join() function also allows you to join on multiple columns by specifying them as a vector in the by
parameter. For example, if you want to join based on both the "id" and "name" columns, you can do it like this:
R
joined_df <- join(df1, df2, by = c("id", "name"))
In addition to the basic join() function, the plyr package provides other join functions like semi_join(), anti_join(), and not_join() that allow you to perform more advanced operations on your data frames.
Overall, the join() function from the plyr package offers a flexible and efficient way to combine two data frames in R. Whether you need to perform an inner join, left join, right join, or outer join, this function has you covered.
Conclusion
In conclusion, combining two data frames in R is a common task that can be accomplished using different methods such as merge(), cbind(), and rbind(). Each method has its advantages and is suitable for different scenarios. The merge() function allows for more complex merging based on specified variables, while cbind() and rbind() are useful for simple concatenation of data frames.
When combining data frames, it is important to pay attention to the structure of the data and the matching variables. Ensuring that the data frames have the same column names or compatible column types will result in successful merging. It is also crucial to handle missing data and duplicate entries appropriately to avoid any unexpected results.
By understanding these methods and considering the specific requirements of your data, you will be able to effectively combine two data frames and perform further analysis or manipulations in R.
FAQs
Q: How do I combine two data frames in R?
Combining two data frames in R can be done using the `merge()` function. This function allows you to merge two data frames based on a common column or key. By specifying the appropriate arguments, you can perform inner, left, right, or full outer joins to combine your data frames.
Q: What is an inner join?
An inner join is a type of merge that returns only the matching rows between the two data frames. It combines the rows based on the values in the common column or key present in both data frames. Rows that do not have matching values in both data frames are excluded from the result.
Q: How do I perform an inner join in R?
To perform an inner join in R, you can use the `merge()` function with the `all = FALSE` argument. This will ensure that only the matching rows are included in the result. For example:
```r
merged_df <- merge(df1, df2, by = "common_column", all = FALSE)
```
Q: What is an outer join?
An outer join is a type of merge that returns all the rows from both data frames, matching the values if available and filling in missing values with NA where there is no match. It combines the rows based on the values in the common column or key present in both data frames.
Q: How do I perform an outer join in R?
To perform an outer join in R, you can use the `merge()` function with the `all = TRUE` argument. This will include all the rows from both data frames in the result, matching the values if available and filling in missing values with NA where there is no match. For example:
```r
merged_df <- merge(df1, df2, by = "common_column", all = TRUE)
```