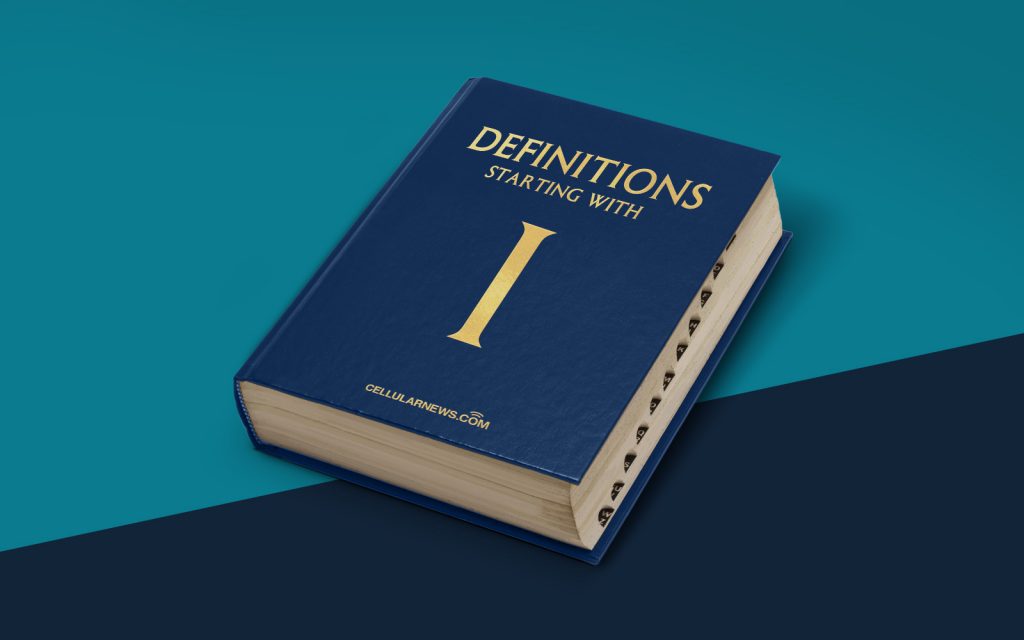
DEFINITIONS: What is an Insertion Sort?
Have you ever wondered how computers arrange and organize data? One of the fundamental algorithms used in computer science for sorting data is the Insertion Sort. In this blog post, we’ll explore the concept of Insertion Sort, how it works, and its significance in data processing. So, let’s dive in and demystify the Insertion Sort algorithm!
Key Takeaways:
- An Insertion Sort is a simple and efficient sorting algorithm.
- It works by repeatedly inserting an element into its correct position within a sorted subarray.
Understanding Insertion Sort:
The Insertion Sort algorithm is like arranging a deck of cards in your hand. Just as you would sort the cards one by one, Insertion Sort works by iterating through an unsorted list of elements and placing each element at its appropriate position within a sorted subarray. This process continues until the entire list is sorted. Let’s break down the steps of the Insertion Sort algorithm:
- Start with the second element in the list.
- Compare this element with the previous elements in the sorted subarray.
- If the current element is smaller, shift the larger elements to the right.
- Repeat steps 2 and 3 until the current element is in its correct sorted position.
- Move on to the next element in the unsorted list and repeat the process.
- Continue until all elements are sorted.
The beauty of the Insertion Sort algorithm lies in its efficiency for sorting small lists or partially sorted data. It has a time complexity of O(n^2), which means it takes approximately n^2 comparisons and swaps to sort an array of n elements. Although not the most efficient algorithm for large datasets, Insertion Sort excels in situations where the data is already partially sorted.
Benefits of Insertion Sort:
- Simple Implementation: Insertion Sort is easy to understand and implement, making it a great choice for beginners in programming.
- Adaptive: Insertion Sort performs well when the data is already mostly sorted, requiring fewer comparisons and swaps.
- In-Place Sorting: The algorithm does not require additional memory, as it rearranges the elements within the existing array or list.
In conclusion, an Insertion Sort algorithm is a sorting technique that arranges elements by repeatedly inserting each element into its correct position within a sorted subarray. While it may not be the fastest sorting algorithm for large datasets, it shines in situations where the data is mostly sorted or when simplicity and clarity are of utmost importance.
Next time you’re faced with an unsorted list of elements, keep the Insertion Sort in mind as a powerful tool to bring order to chaos. Happy sorting!